import java.text.DecimalFormat; // Class representing a student public class Student { private String first; // first name private String last; // last name private double gpa; // grade point average // Student class constructor public Student(String first, String last, double gpa) { this.first = first; // first name this.last = last; // last name this.gpa = gpa; // grade point average } public double getGPA() { return gpa; } public String getLast() { return last; } // Returns a String representation of the Student object, with the GPA formatted to one decimal public String toString() { DecimalFormat fmt = new DecimalFormat("#.0"); return first + " " + last + " " + "(GPA: " + fmt.format(gpa) + ")"; } } public class LabProgram { public static void main(String args[]) { Scanner scnr = new Scanner(System.in); Course course = new Course(); int beforeCount; // Example students for testing course.addStudent(new Student("Henry", "Nguyen", 3.5)); course.addStudent(new Student("Brenda", "Stern", 2.0)); course.addStudent(new Student("Lynda", "Robison", 3.2)); course.addStudent(new Student("Sonya", "King", 3.9)); Student student = course.findStudentHighestGpa(); System.out.println("Top student: " + student); } }
import java.text.DecimalFormat; // Class representing a student public class Student { private String first; // first name private String last; // last name private double gpa; // grade point average // Student class constructor public Student(String first, String last, double gpa) { this.first = first; // first name this.last = last; // last name this.gpa = gpa; // grade point average } public double getGPA() { return gpa; } public String getLast() { return last; } // Returns a String representation of the Student object, with the GPA formatted to one decimal public String toString() { DecimalFormat fmt = new DecimalFormat("#.0"); return first + " " + last + " " + "(GPA: " + fmt.format(gpa) + ")"; } } public class LabProgram { public static void main(String args[]) { Scanner scnr = new Scanner(System.in); Course course = new Course(); int beforeCount; // Example students for testing course.addStudent(new Student("Henry", "Nguyen", 3.5)); course.addStudent(new Student("Brenda", "Stern", 2.0)); course.addStudent(new Student("Lynda", "Robison", 3.2)); course.addStudent(new Student("Sonya", "King", 3.9)); Student student = course.findStudentHighestGpa(); System.out.println("Top student: " + student); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
import java.text.DecimalFormat;
// Class representing a student
public class Student {
private String first; // first name
private String last; // last name
private double gpa; // grade point average
// Student class constructor
public Student(String first, String last, double gpa) {
this.first = first; // first name
this.last = last; // last name
this.gpa = gpa; // grade point average
}
public double getGPA() {
return gpa;
}
public String getLast() {
return last;
}
// Returns a String representation of the Student object, with the GPA formatted to one decimal
public String toString() {
DecimalFormat fmt = new DecimalFormat("#.0");
return first + " " + last + " " + "(GPA: " + fmt.format(gpa) + ")";
}
}
public class LabProgram {
public static void main(String args[]) {
Scanner scnr = new Scanner(System.in);
Course course = new Course();
int beforeCount;
// Example students for testing
course.addStudent(new Student("Henry", "Nguyen", 3.5));
course.addStudent(new Student("Brenda", "Stern", 2.0));
course.addStudent(new Student("Lynda", "Robison", 3.2));
course.addStudent(new Student("Sonya", "King", 3.9));
Student student = course.findStudentHighestGpa();
System.out.println("Top student: " + student);
}
}
public static void main(String args[]) {
Scanner scnr = new Scanner(System.in);
Course course = new Course();
int beforeCount;
// Example students for testing
course.addStudent(new Student("Henry", "Nguyen", 3.5));
course.addStudent(new Student("Brenda", "Stern", 2.0));
course.addStudent(new Student("Lynda", "Robison", 3.2));
course.addStudent(new Student("Sonya", "King", 3.9));
Student student = course.findStudentHighestGpa();
System.out.println("Top student: " + student);
}
}
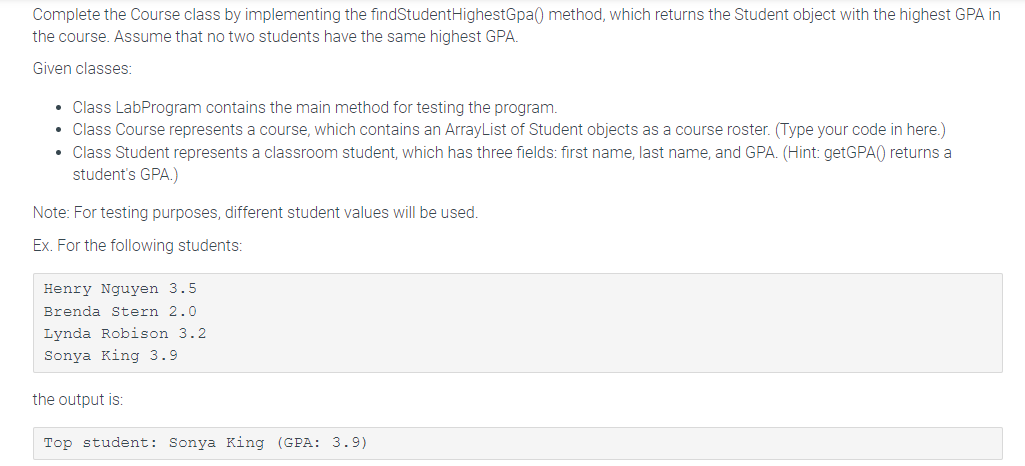
Transcribed Image Text:Complete the Course class by implementing the findStudentHighestGpa() method, which returns the Student object with the highest GPA in
the course. Assume that no two students have the same highest GPA.
Given classes:
• Class LabProgram contains the main method for testing the program.
• Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.)
• Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: getGPA() returns a
student's GPA.)
Note: For testing purposes, different student values will be used.
Ex. For the following students:
Henry Nguyen 3.5
Brenda Stern 2.0
Lynda Robison 3.2
Sonya King 3.9
the output is:
Top student: Sonya King (GPA: 3.9)
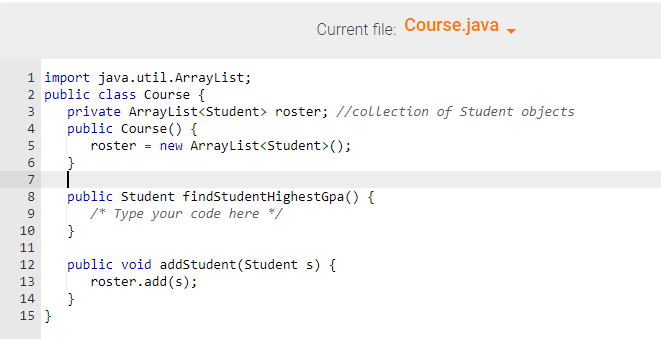
Transcribed Image Text:1 import java.util.ArrayList;
2 public class Course {
3 private ArrayList<Student> roster; //collection of Student objects
public Course() {
4
5
roster = new ArrayList<Student> ();
6710
9
}
8 public Student findStudentHighestGpa () {
/* Type your code here */
10 }
11
12
13
14
15 }
Current file: Course.java
public void addStudent (Student s) {
roster.add(s);
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
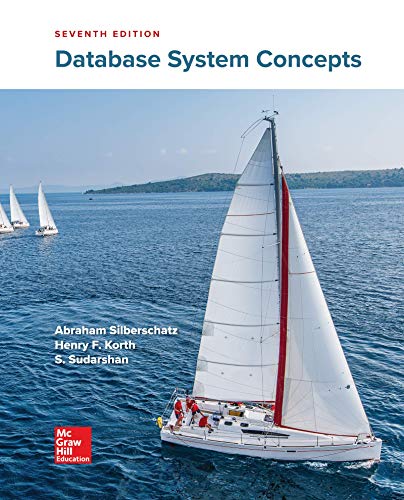
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
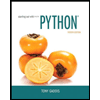
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
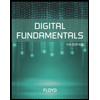
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
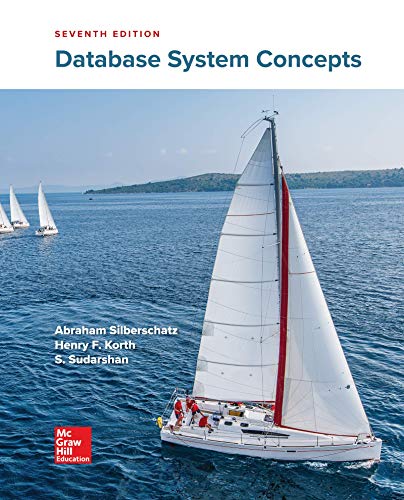
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
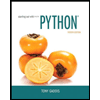
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
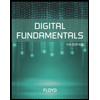
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
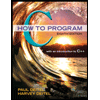
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
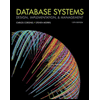
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
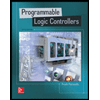
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education