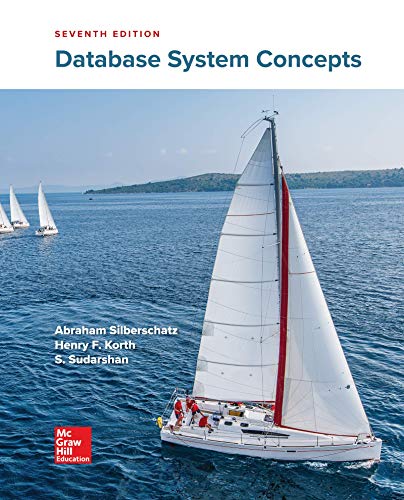
import java.util.Scanner;
public class InstrumentInformation {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Instrument myInstrument = new Instrument();
StringInstrument myStringInstrument = new StringInstrument();
String instrumentName, manufacturerName, stringInstrumentName, stringManufacturer;
int yearBuilt, cost, stringYearBuilt, stringCost, numStrings, numFrets;
boolean bowed;
instrumentName = scnr.nextLine();
manufacturerName = scnr.nextLine();
yearBuilt = scnr.nextInt();
scnr.nextLine();
cost = scnr.nextInt();
scnr.nextLine();
stringInstrumentName = scnr.nextLine();
stringManufacturer = scnr.nextLine();
stringYearBuilt = scnr.nextInt();
stringCost = scnr.nextInt();
numStrings = scnr.nextInt();
numFrets = scnr.nextInt();
bowed = scnr.nextBoolean();
myInstrument.setName(instrumentName);
myInstrument.setManufacturer(manufacturerName);
myInstrument.setYearBuilt(yearBuilt);
myInstrument.setCost(cost);
myInstrument.printInfo();
myStringInstrument.setName(stringInstrumentName);
myStringInstrument.setManufacturer(stringManufacturer);
myStringInstrument.setYearBuilt(stringYearBuilt);
myStringInstrument.setCost(stringCost);
myStringInstrument.setNumOfStrings(numStrings);
myStringInstrument.setNumOfFrets(numFrets);
myStringInstrument.setIsBowed(bowed);
myStringInstrument.printInfo();
System.out.println(" Number of strings: " + myStringInstrument.getNumOfStrings());
System.out.println(" Number of frets: " + myStringInstrument.getNumOfFrets());
System.out.println(" Is bowed: " + myStringInstrument.getIsBowed());
}
}
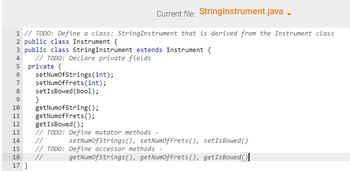
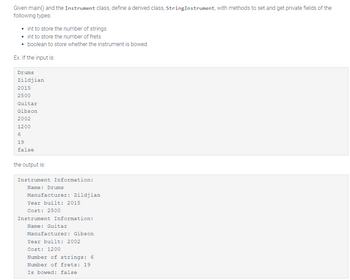

Step by stepSolved in 4 steps with 4 images

- Here is a statement: class temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; How do I write the definition of the member function set so that the instance variables are set according to the parameters?arrow_forwardpublic class KnowledgeCheckTrek { public static final String TNG = "The Next Generation"; public static final String DS9 = "Deep Space Nine"; public static final String VOYAGER = "Voyager"; public static String trek(String character) { if (character != null && (character.equalsIgnoreCase("Picard") || character.equalsIgnoreCase("Data"))) { // IF ONE return TNG; } else if (character != null && character.contains("7")) { // IF TWO return VOYAGER; } else if ((character.contains("Quark") || character.contains("Odo"))) { // IF THREE return DS9; } return null; } public static void main(String[] args) { System.out.println(trek("Captain Picard")); System.out.println(trek("7 of Nine")); System.out.println(trek("Odo")); System.out.println(trek("Quark")); System.out.println(trek("Data").equalsIgnoreCase(TNG));…arrow_forwardimport java.util.Scanner;/** This program computes the time required to double an investment with an annual contribution.*/public class DoubleInvestment{ public static void main(String[] args) { final double RATE = 5; final double INITIAL_BALANCE = 10000; final double TARGET = 2 * INITIAL_BALANCE; Scanner in = new Scanner(System.in); System.out.print("Annual contribution: "); double contribution = in.nextDouble(); double balance = INITIAL_BALANCE; int year = 0; // TODO: Add annual contribution, but not in year 0 do { year++; double interest = balance*(RATE/100); balance = (balance+contribution+interest); } while(balance< TARGET); System.out.println("Year: " + year); System.out.printf("Balance: %.2f%n", balance); }}arrow_forward
- Complete the isExact Reverse() method in Reverse.java as follows: The method takes two Strings x and y as parameters and returns a boolean. The method determines if the String x is the exact reverse of the String y. If x is the exact reverse of y, the method returns true, otherwise, the method returns false. Other than uncommenting the code, do not modify the main method in Reverse.java. Sample runs provided below. Argument String x "ba" "desserts" "apple" "regal" "war" "pal" Argument String y "stressed" "apple" "lager" "raw" "slap" Return Value false true false true true falsearrow_forwardFix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forwardpublic class Side Plot { * public static String PLOT_CHAR = "*"; public static void main(String[] args) { plotxSquared (-6,6); * plotNegXSquaredPlus20 (-5,5); plotAbsXplus1 (-4,4); plotSinWave (-9,9); public static void plotXSquared (int minX, int maxx) { System.out.println("Sideways Plot"); System.out.println("y = x*x where + minX + "= minX; row--) { for (int col = 0; col row * row; col++) { System.out.print (PLOT_CHAR); if (row== 0){ } System.out.print(" "); } for(int i = 0; i <= maxx* maxX; i++) { System.out.print("-"); } System.out.println(); Sideways PHOT y = x*x where -6<=x<=6 Sideways Plot y = x*x where -6<=x<=6 How do I fix my code to get a plot like 3rd picture?arrow_forward
- java languageSchedule the People classIn main file, create an object of class PeopleMake the showPeople method callarrow_forwardcomplete the missing code. public class Exercise09_04Extra { public static void main(String[] args) { SimpleTime time = new SimpleTime(); time.hour = 2; time.minute = 3; time.second = 4; System.out.println("Hour: " + time.hour + " Minute: " + time.minute + " Second: " + time.second); } } class SimpleTime { // Complete the code to declare the data fields hour, // minute, and second of the int type }arrow_forwardpublic class LabProgram { public static void main(String args[]) { Course course = new Course(); String first; // first name String last; // last name double gpa; // grade point average first = "Henry"; last = "Cabot"; gpa = 3.5; course.addStudent(new Student(first, last, gpa)); // Add 1st student first = "Brenda"; last = "Stern"; gpa = 2.0; course.addStudent(new Student(first, last, gpa)); // Add 2nd student first = "Jane"; last = "Flynn"; gpa = 3.9; course.addStudent(new Student(first, last, gpa)); // Add 3rd student first = "Lynda"; last = "Robison"; gpa = 3.2; course.addStudent(new Student(first, last, gpa)); // Add 4th student course.printRoster(); } } // Class representing a student public class Student { private String first; // first name private String last; // last name private double gpa; // grade point average…arrow_forward
- class TenNums {private: int *p; public: TenNums() { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = i; } void display() { for (int i = 0; i < 10; i++) cout << p[i] << " "; }};int main() { TenNums a; a.display(); TenNums b = a; b.display(); return 0;} Continuing from Question 4, let's say I added the following overloaded operator method to the class. Which statement will invoke this method? TenNums TenNums::operator+(const TenNums& b) { TenNums o; for (int i = 0; i < 10; i++) o.p[i] = p[i] + b.p [i]; return o;} Group of answer choices TenNums a; TenNums b = a; TenNums c = a + b; TenNums c = a.add(b);arrow_forwardpublic class Animal { public static int population; private int age; } public Animal (int age) { this.age = age; population++; } + msg); public void say (String msg) { System.out.print("Saying: " System.out.print(" for "); System.out.print(getHuman Years()); System.out.println(" years."); } public int getHuman Years() { return age; } public class Mammal extends Animal { private String species; public Mammal(String species, int age) { super (age); this. species species; } } } = public int getHuman Years() { if (species.equals("dog")) 1 else return super.getHumanYears() * 7; return super.getHumanYears(); €arrow_forwardimport java.util.Scanner;public class MP2{public static void main (String[] args){//initialization and declarationScanner input = new Scanner (System.in);String message;int Option1;int Option2;int Quantity;int TotalBill;int Cash;int Change;final int SPECIALS = 1;final int BREAKFAST = 2;final int LUNCH = 3;final int SANDWICHES = 4;final int DRINKS = 5;final int DESSERTS = 6;//For the Special, Breakfast, Lunch Optionsfinal int Meals1 = 11;final int Meals2 = 12;final int Meals3 = 13;final int Meals4 = 14;//First menu and First OptionSystem.out.println("\t Welcome to My Kitchen ");System.out.println("\t\t SPECIALS");System.out.println("\t\t [2] BREAKFAST MEALS");System.out.println("\t\t [3] LUNCH MEALS");System.out.println("\t\t [4] SANDWICHES");System.out.println("\t\t [5] DRINKS");System.out.println("\t\t [6] DESSERTS");System.out.println("\t\t [0] EXIT");System.out.print("Choose an option ");Option1 = input.nextInt();//first switch case statementsswitch(Option1){case (SPECIALS):message…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
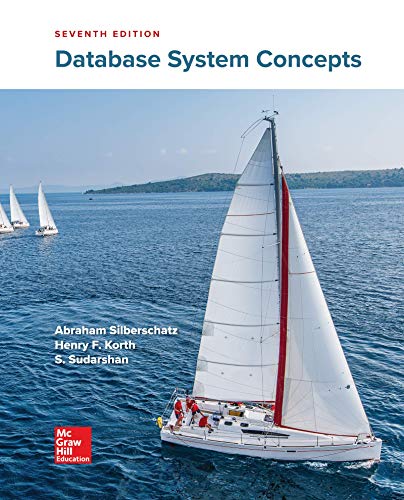
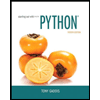
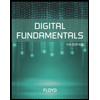
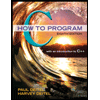
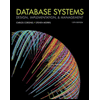
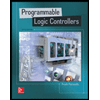