import java.util.Arrays; public class Solver { // This constructor is called once to make a Solver object. // My tests will then use that one Solver object to solve many puzzles. // The most direct/intuitive solution leaves this constrctor blank. // However, adding some "preprocessing" code to this constructor // that executes before any puzzles are solved could potentially // speed up your solve method. public Solver() { } /** * Solves a given puzzle represented as a 5-by-5 boolean array with * 16 trues and 9 falses. * @param start the intial puzzle/board configuration * @return the sequence of moves that solves the puzzle. */ public char[] solve(boolean[][] puzzle) { //throw new RuntimeException("Not implemented"); } // A simple toy exmaple of how a Solver object is used. public static void main(String[] args) { boolean[][] bb = { { true, true, true, true, true}, {false, true, true, false, false}, { true, false, false, false, true}, { true, false, false, false, true}, { true, true, true, true, true} }; boolean[][] Xbb = { { true, true, true, true, true}, {false, true, true, false, false}, { true, false, true, false, true}, { true, false, false, false, true}, { true, true, false, true, true} }; Solver solver = new Solver(); char[] sol = solver.solve(bb); System.out.println(Arrays.toString(sol)); sol = solver.solve(Xbb); System.out.println(Arrays.toString(sol)); } } Implement the Solver class. In doing so, you are allowed to define other classes to help you (as well as use "built-in" Java classes or the book's classes). The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of booleans and returns a char array containing a minimal sequence of moves that will lead to the solved board (all the cells around the edges being filled). The board configuration is passed in as a 5-by-5 boolean array of Booleans with exactly 16 true cells (filled) and 9 false cells (empty). The solve method then returns an array of characters representing a minimal sequence of moves that solves the puzzle. In other words, if the characters from the returned array are used in order as input to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. Furthermore, the solution must be minimal in the sense that there are no solutions that use fewer moves (although there could be other solutions that use the same number of moves). If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. The class also has a no argument constructor that will be called to construct/initialize the solver object. (There are perfectly valid solutions to the project where the constructor does absolutely nothing, but there are also solutions where some preprocessing work done in the constructor allows the solve method to be much faster.) This is a 2D array. Include a breadth-first search/BinarySearchST in the method. Use the Array import to solve. You may use other imports as well.
import java.util.Arrays;
public class Solver {
// This constructor is called once to make a Solver object.
// My tests will then use that one Solver object to solve many puzzles.
// The most direct/intuitive solution leaves this constrctor blank.
// However, adding some "preprocessing" code to this constructor
// that executes before any puzzles are solved could potentially
// speed up your solve method.
public Solver() {
}
/** * Solves a given puzzle represented as a 5-by-5 boolean array with
* 16 trues and 9 falses.
* @param start the intial puzzle/board configuration
* @return the sequence of moves that solves the puzzle.
*/
public char[] solve(boolean[][] puzzle) {
//throw new RuntimeException("Not implemented");
}
// A simple toy exmaple of how a Solver object is used.
public static void main(String[] args) {
boolean[][] bb = {
{ true, true, true, true, true},
{false, true, true, false, false},
{ true, false, false, false, true},
{ true, false, false, false, true},
{ true, true, true, true, true}
};
boolean[][] Xbb = {
{ true, true, true, true, true},
{false, true, true, false, false},
{ true, false, true, false, true},
{ true, false, false, false, true},
{ true, true, false, true, true}
};
Solver solver = new Solver();
char[] sol = solver.solve(bb);
System.out.println(Arrays.toString(sol));
sol = solver.solve(Xbb);
System.out.println(Arrays.toString(sol));
}
}
Implement the Solver class. In doing so, you are allowed to define other classes to help you (as well as use "built-in" Java classes or the book's classes). The point of the solver class is the solve method which takes a board/puzzle configuration represented as a 2D array of booleans and returns a char array containing a minimal sequence of moves that will lead to the solved board (all the cells around the edges being filled). The board configuration is passed in as a 5-by-5 boolean array of Booleans with exactly 16 true cells (filled) and 9 false cells (empty). The solve method then returns an array of characters representing a minimal sequence of moves that solves the puzzle. In other words, if the characters from the returned array are used in order as input to the move method on the Board object representing the initial configuration, the resulting board configuration represents the solved board. Furthermore, the solution must be minimal in the sense that there are no solutions that use fewer moves (although there could be other solutions that use the same number of moves). If the input to the solve method is a board configuration that is already solved, then solution requires no moves and an array of size 0 must be returned. The class also has a no argument constructor that will be called to construct/initialize the solver object. (There are perfectly valid solutions to the project where the constructor does absolutely nothing, but there are also solutions where some preprocessing work done in the constructor allows the solve method to be much faster.)
This is a 2D array. Include a breadth-first search/BinarySearchST in the method. Use the Array import to solve. You may use other imports as well.

Step by step
Solved in 4 steps with 5 images

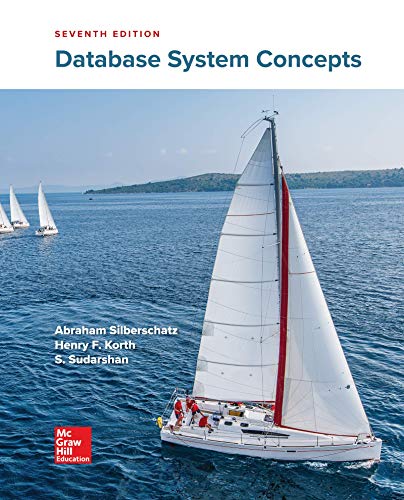
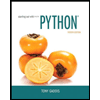
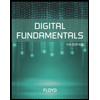
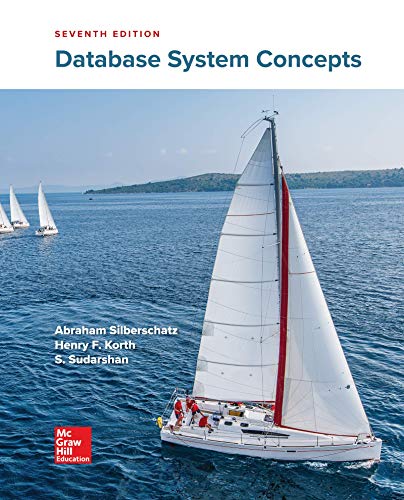
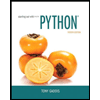
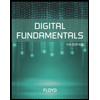
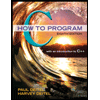
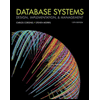
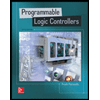