import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set and target as arguments boolean result = linearSearch(numbers, target); // Check the result and print the appropriate message if (result) { System.out.println("Target found in the Set."); } else { System.out.println("Target not found in the Set."); } } } --------------------------------------------------------------------------------------------------------------------------------------------------------- Define the class LinearSearchSet: This class will contain the method to perform a linear search on a Set of integers. Define the linearSearch method: This method takes in a Set of integers and an integer target as parameters. The method uses a for-each loop to iterate over all of the elements in the Set. For each element, the method checks if the current value is equal to the target. If the target is found, the method returns true. If the loop completes and the target was not found, the method returns false. Define the main method: In this method, create a HashSet of integers called numbers and add the following integers to it: Copy 1 2 3 4 5 6 Set numbers = new HashSet<>(); numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); Your target is an integer with a value of 6. Call the linearSearch method with the set and the target as arguments. Store the result of the search as a boolean variable called result. If the result is true, the message "Target found in the Set." should be printed to the console. If the result is false, the message "Target not found in the Set." should be printed to the console.
-
Define the class LinearSearchSet:
-
This class will contain the method to perform a linear search on a Set of integers.
-
-
Define the linearSearch method:
-
This method takes in a Set of integers and an integer target as parameters.
-
The method uses a for-each loop to iterate over all of the elements in the Set.
-
For each element, the method checks if the current value is equal to the target.
-
If the target is found, the method returns true.
-
If the loop completes and the target was not found, the method returns false.
-
-
Define the main method:
-
In this method, create a HashSet of integers called numbers and add the following integers to it:
Copy123456Set<Integer> numbers = new HashSet<>();numbers.add(3);numbers.add(6);numbers.add(2);numbers.add(9);numbers.add(11); -
Your target is an integer with a value of 6.
-
Call the linearSearch method with the set and the target as arguments.
-
Store the result of the search as a boolean variable called result.
-
If the result is true, the message "Target found in the Set." should be printed to the console.
-
If the result is false, the message "Target not found in the Set." should be printed to the console.
-

Step by step
Solved in 4 steps with 2 images

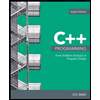
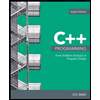