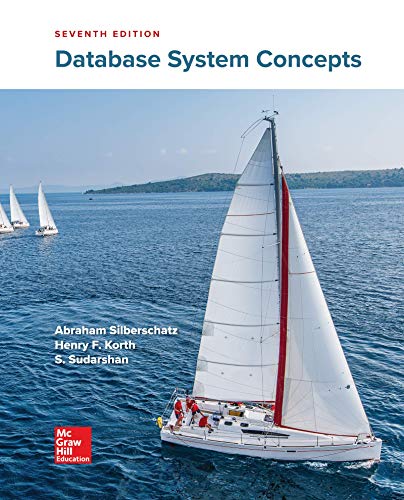
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
import java.util.HashMap;
import java.util.Map;
public class LinearSearchMap {
// Define a method that takes in a map and a target value as parameters
public static boolean linearSearch(Map<String, Integer> map, int target) {
// Iterate through each entry in the map
for () {
// Check if the value of the current entry is equal to the target
if () {
// If the value is equal to the target, return true
}
}
// If no entry with the target value is found, return false
}
public static void main(String[] args) {
// Create a HashMap of strings and integers
Map<String, Integer> numbers = new HashMap<>();
// Populate the HashMap with key-value pairs
numbers.put("One", 1);
numbers.put("Two", 2);
numbers.put("Three", 3);
numbers.put("Four", 4);
numbers.put("Five", 5);
// Set the target value to search for Call the linearSearch method with the map
// and target value as parameters
boolean result = linearSearch(numbers, target);
// Check if the target was found in the map
if (result) {
System.out.println("Target found in the Map.");
} else {
System.out.println("Target not found in the Map.");
}
}
}
---------------------------------------------------------------------------------------------------------------------------------------------------------
-
Set the target value be an integer with a value of 3.
-
Call the linearSearch method with the Map and target value as parameters.
-
Store the result of the search as a boolean variable called result.
-
If the result is true, the message "Target found in the Map." should be printed to the console.
-
If the result is false, the message "Target not found in the Map." should be printed to the console.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- import java.util.*;public class MyLinkedListQueue{ class Node { public Object data; public Node next; private Node first; public MyLinkedListQueue() { first = null; } public Object peek() { if(first==null) { throw new NoSuchElementException(); } return first.data; } public void enqueue(Object element) { Node newNode = new newNode(); newNode.data = element; newNode.next = first; first = newNode; } public boolean isEmpty() { return(first==null); } public int size() { int count = 0; Node p = first; while(p ==! null) { count++; p = p.next; } return count; } } } Hello, I am getting an error message when I execute this program. This is what I get: MyLinkedListQueue.java:11: error: invalid method declaration; return type required public MyLinkedListQueue() ^1 error I also need to have a dequeue method in my program. It is very similar to the enqueue method. I'll…arrow_forwardImplement move the following way. public int[] walk(int... stepCounts) { // Implement the logic for walking the players returnnewint[0]; } public class WalkingBoardWithPlayers extends WalkingBoard{ privatePlayer[] players; privateintround; publicstaticfinalintSCORE_EACH_STEP=13; publicWalkingBoardWithPlayers(int[][] board, intplayerCount) { super(board); initPlayers(playerCount); } publicWalkingBoardWithPlayers(intsize, intplayerCount) { super(size); initPlayers(playerCount); } privatevoidinitPlayers(intplayerCount) { if(playerCount <2){ thrownewIllegalArgumentException("Player count must be at least 2"); } else { this.players=newPlayer[playerCount]; this.players[0] =newMadlyRotatingBuccaneer(); for (inti=1; i < playerCount; i++) { this.players[i] =newPlayer(); } } } package walking.game.player; import walking.game.util.Direction; public class Player{ privateintscore; protectedDirectiondirection=Direction.UP; publicPlayer() {} publicintgetScore() { return score; }…arrow_forward4. Show the output of the following code: public class Test { public static void main(String[] args) { Map map = new LinkedHashMap(); map.put("123", "John Smith"); map.put ("111", "George Smith"); map.put("123", "Steve Yao"); map.put("222", "Steve Yao"); System.out:println("(1) System.out.println(" (2) } uutr wtni " + map); + new TreeMap (map)) ; }arrow_forward
- BuiltInFunctionDefinitionNode.java has an error so make sure to fix it. BuiltInFunctionDefinitionNode.java import java.util.HashMap; import java.util.function.Function; public class BuiltInFunctionDefinitionNode extends FunctionDefinitionNode { private Function<HashMap<String, InterpreterDataType>, String> execute; private boolean isVariadic; public BuiltInFunctionDefinitionNode(Function<HashMap<String, InterpreterDataType>, String> execute, boolean isVariadic) { this.execute = execute; this.isVariadic = isVariadic; } public String execute(HashMap<String, InterpreterDataType> parameters) { return this.execute.apply(parameters); } }arrow_forwardConsider the GameOfLife class public class GameOfLife { private BooleanProperty[][] cells; public GameOfLife() { cells = new BooleanProperty[10][10]; for (int x = 0; x < 10; x++) { for (int y = 0; y < 10; y++) { cells[x][y] = new SimpleBooleanProperty(); public void ensureAlive(int x, int y) { cells[x][y].set(true); public void ensureDead(int x, int y) { cells[x][y]•set(false); public boolean isAlive(int x, int y) { return cells[x][y]•get(); } a. Identify one code smell in this program. b. Identify and explain the most pertinent (problematic) design smell in this program.arrow_forwardConsider the following class map, class map { public: map(ifstream &fin); void print(int,int,int,int); bool isLegal(int i, int j); void setMap(int i, int j, int n); int getMap(int i, int j) const; int getReverseMapI(int n) const; int getReverseMapJ(int n) const; void mapToGraph(graph &g); bool findPathRecursive(graph &g, stack<int> &moves); bool findPathNonRecursive1(graph &g, stack<int> &moves); bool findPathNonRecursive2(graph &g, queue<int> &moves); bool findShortestPath1(graph &g, stack<int> &bestMoves); bool findShortestPath2(graph &, vector<int> &bestMoves); void map::printPath(stack<int> &s); int numRows(){return rows;}; int numCols(){return cols;}; private: int rows; // number of latitudes/rows in the map int cols; // number of longitudes/columns in the map matrix<bool> value; matrix<int> mapping; // Mapping from latitude and longitude co-ordinates (i,j) values to node index…arrow_forward
- Can someone help me with this code, I not really understanding how to do this? import java.util.ArrayList;import java.util.HashMap;import java.util.Map;/*** @version Spring 2019* @author Kyle*/public class MapProblems {/*** Modify and return the given map as follows: if the key "a" has a value, set the key "b" to* have that value, and set the key "a" to have the value "". Basically "b" is confiscating the* value and replacing it with the empty string.** @param map to be edited* @return map*/public Map<String, String> confiscate(Map<String, String> map) {if (map.containsKey("a") && map.containsValue("a")) {}}/*** Modify and return the given map as follows: if the key "duck" has a value, set the key* "goose" to have that same value. In all cases remove the key "swan", the rest of the map* should not change.** @param map to be edited* @return map*/public Map<String, String> mapBird1(Map<String, String> map) {throw new UnsupportedOperationException("Not…arrow_forwardimport java.util.Comparator; import java.util.PriorityQueue; class StringLengthComparator implements Comparator public int compare(String o1, String 02) if (01.length()02.length()){ return 1; }else{ } } } } class StringLengthComparator1 implements Comparator { return 0; public int compare(String o1, String o2) { if(01.length()02.length()){ return -1;//means here we swap it }else{ } } return 0; public class StringLength { public static void main(String[] args) { String arr[] = {"this", "at", "a", "their", "queues"}; StringLengthComparator string Comparator = new StringLengthComparator(); StringLengthComparator1 string Comparator1 = new StringLengthComparator1(); PriorityQueue pq = new PriorityQueue(stringComparator); PriorityQueue pq1 = new PriorityQueue(stringComparator1); for(int i=0;iarrow_forwardWrite a recursive instance method isSorted that takes a Link parameter and determines whether a linked list is sorted in descending order or not (return a boolean value).arrow_forward
- implement changeArrayLength(int m) – void method; public class Course { public String code; public int capacity; public SLinkedList<Student>[] studentTable; public int size; public SLinkedList<Student> waitlist; public Course(String code) { this.code = code; this.studentTable = new SLinkedList[10]; this.size = 0; this.waitlist = new SLinkedList<Student>(); this.capacity = 10; } public Course(String code, int capacity) { this.code = code; this.studentTable = new SLinkedList[capacity]; this.size = 0; this.waitlist = new SLinkedList<>(); this.capacity = capacity; } public void changeArrayLength(int m) { // insert your solution here } This method creates a new student table for this course; this new table has the given length m; the method moves all registered students to this table, as described in more detail below. Note that this method itself…arrow_forward25. Show the output of the following code: public class Test { public static void main(String[] args) { Map map = new LinkedHashMap(); map.put("123", "John Smith"); map.put("111", "George Smith"); map.put("123", "Steve Yao"); map.put("222", "Steve Yao"); System.out.printin("(1) " + map): System.out.printin("(2) "+ new TreeMap(map)): } }arrow_forwardExercise 2: Consider the following class: public class Sequence { private ArrayList values; public Sequence() { values = new ArrayList(); } public void add(int n) { values.add(n); } public String toString() { return values.toString(); } } Add a method public Sequence merge(Sequence other) that merges two sequences, alternating ele- ments from both sequences. If one sequence is shorter than the other, then alternate as long as you can and then append the remaining elements from the longer sequence. For example, if a is 1 4 9 16 and b is 9 7 4 9 11 then a.merge(b) returns the sequence 1 9 4 7 9 4 16 9 11 without modifying a or b.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
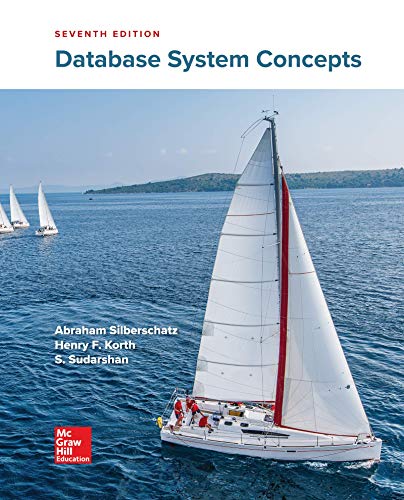
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
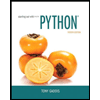
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
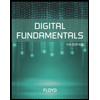
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
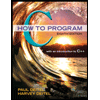
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
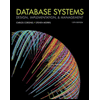
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
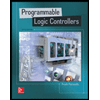
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education