IMPORTANT: Your isHappy function must not call itself. Subtask III: There are in fact many levels of happiness. The larger the number, the happier we feel about that number. The k-th happy number is the k-th smallest happy number. This means, the 1st happy number is the smallest happy number, which is 1. The 2nd happy number is the second smallest happy number, which is 7. Below is a list of the first few happy numbers: 1, 7, 10, 13, 19, 23, 28, 31, 32, 44, 49, 68, 70, 79, 82, 86, 91, 94, 97, 100, 103, 109, 129, 130, 133, 139, 167, 176, 188, 190, ... Implement a function kThHappy (k: int) -> int that computes and returns the k-th happy num- ber. For example: kThHappy (1) returns 1 kThHappy (3) returns 10 kThHappy (11) returns 49 kThHappy (19) returns 97
IMPORTANT: Your isHappy function must not call itself. Subtask III: There are in fact many levels of happiness. The larger the number, the happier we feel about that number. The k-th happy number is the k-th smallest happy number. This means, the 1st happy number is the smallest happy number, which is 1. The 2nd happy number is the second smallest happy number, which is 7. Below is a list of the first few happy numbers: 1, 7, 10, 13, 19, 23, 28, 31, 32, 44, 49, 68, 70, 79, 82, 86, 91, 94, 97, 100, 103, 109, 129, 130, 133, 139, 167, 176, 188, 190, ... Implement a function kThHappy (k: int) -> int that computes and returns the k-th happy num- ber. For example: kThHappy (1) returns 1 kThHappy (3) returns 10 kThHappy (11) returns 49 kThHappy (19) returns 97
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter10: Classes And Data Abstraction
Section: Chapter Questions
Problem 19PE
Related questions
Question
100%
Python Language
Make a happy number function
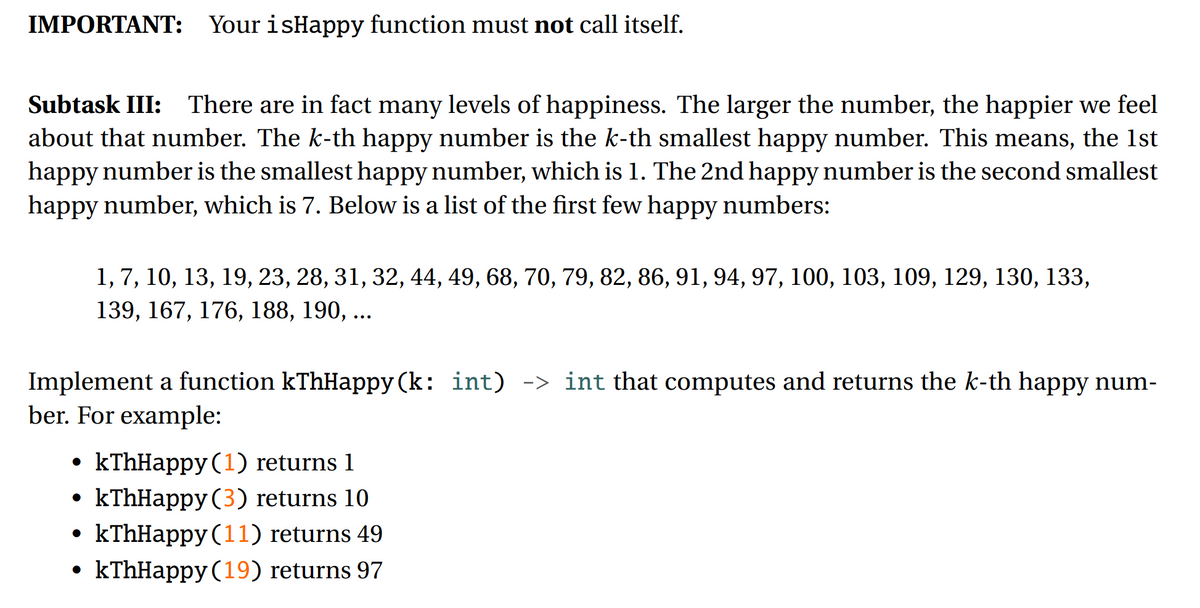
Transcribed Image Text:IMPORTANT: Your isHappy function must not call itself.
Subtask III: There are in fact many levels of happiness. The larger the number, the happier we feel
about that number. The k-th happy number is the k-th smallest happy number. This means, the 1st
happy number is the smallest happy number, which is 1. The 2nd happy number is the second smallest
happy number, which is 7. Below is a list of the first few happy numbers:
1,7, 10, 13, 19, 23, 28, 31, 32, 44, 49, 68, 70, 79, 82, 86, 91, 94, 97, 100, 103, 109, 129, 130, 133,
139, 167, 176, 188, 190, ...
Implement a function kThHappy (k: int) -> int that computes and returns the k-th happy num-
ber. For example:
kThHappy (1) returns 1
kThHappy (3) returns 10
• kThHappy (11) returns 49
• kThHappy (19) returns 97
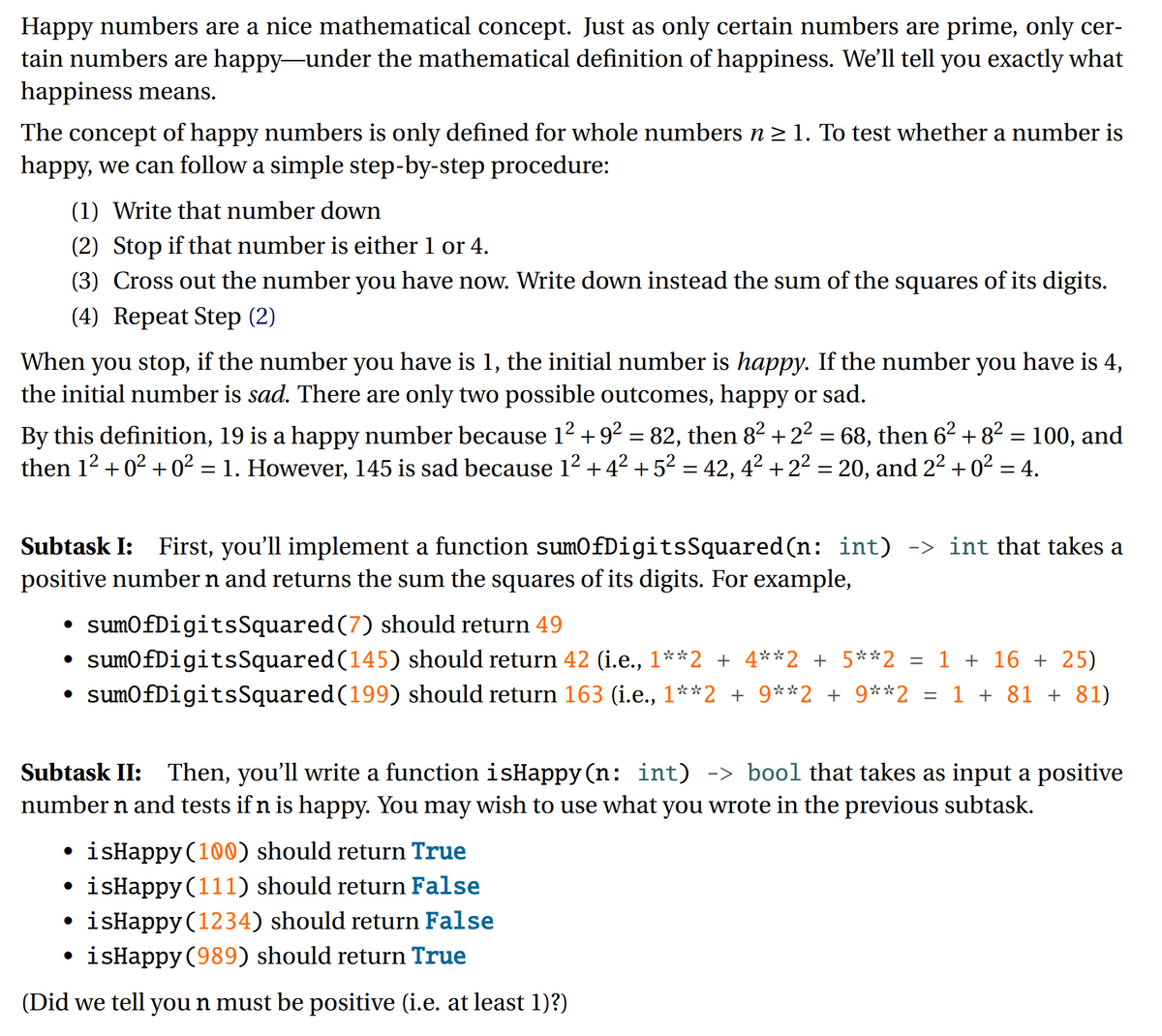
Transcribed Image Text:Happy numbers are a nice mathematical concept. Just as only certain numbers are prime, only cer-
tain numbers are happy-under the mathematical definition of happiness. We'll tell you exactly what
happiness means.
The concept of happy numbers is only defined for whole numbers n> 1. To test whether a number is
happy, we can follow a simple step-by-step procedure:
(1) Write that number down
(2) Stop if that number is either 1 or 4.
(3) Cross out the number you have now. Write down instead the sum of the squares of its digits.
(4) Repeat Step (2)
When you stop, if the number you have is 1, the initial number is happy. If the number you have is 4,
the initial number is sad. There are only two possible outcomes, happy or sad.
By this definition, 19 is a happy number because 12 + 92 = 82, then 82 + 22 = 68, then 62 + 8² = 100, and
then 12 + 02 + 0² = 1. However, 145 is sad because 12 + 42 +52 = 42, 42 +22 = 20, and 22 +02 = 4.
Subtask I: First, you'll implement a function sumOfDigitsSquared(n: int) -> int that takes a
positive number n and returns the sum the squares of its digits. For example,
• sumOfDigitsSquared(7) should return 49
sumOfDigitsSquared(145) should return 42 (i.e., 1**2 + 4**2 + 5**2 = 1 + 16 + 25)
• sumOfDigitsSquared(199) should return 163 (i.e., 1**2 + 9**2 + 9**2 = 1 + 81 + 81)
Subtask II: Then, you'll write a function isHappy (n: int) -> bool that takes as input a positive
number n and tests if n is happy. You may wish to use what you wrote in the previous subtask.
isHappy (100) should return True
• isHappy (111) should return False
• isHappy (1234) should return False
isHappy (989) should return True
(Did we tell you n must be positive (i.e. at least 1)?)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
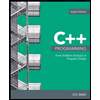
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
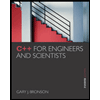
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
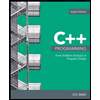
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
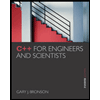
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr