In C++, Can you please look at the code below and revise/fix so it will work according to instructions and criteria. Instruction 1) Write a function that copies a 1D array to a 2D array. The function’s prototype is bool copy1DTo2D(int d1[], int size, int d2[][NCOLS], int nrows); where size > 0 NCOLS > 0 nrows > 0 NCOLS is a global constant size = NCOLS * nrows the function returns true if the parameters and constants satisfy these conditions and false otherwise. the relation between 1d array indices and 2d array indices is 2d row index = 1d array index / NCOLS 2d column index = 1d array index modulus operator NCOLS 2) Write a function that copies a 2D array to a 1D array. The function’s prototype is bool copy2DTo1D(int d2[][NCOLS, int nrows, int d1[], int size); where size > 0 NCOLS > 0 nrows > 0 NCOLS is a global constant the function return true if the parameters and constants satisfy these conditions and false otherwise. the relation between 1d array indices and 2d array indices is 1d array index = NCOLS x 2d array row index + 2d array column index Criteria compiles, runs, tests the program compiles with error, runs without crashing, and tests the functions thoroughly copy 1d to 2d function the function returns true if the parameters and global constant satisfy list of the relationships and restrictions in the problem and false otherwise. the function copies a fixed size 1d array to a fixed size2d array. the function uses the formulas listed in the problem to calculate 2d array index pairs from 1d array indices. copy 2d to 1d function the function returns true if the parameters and global constant satisfy list of the relationships and restrictions in the problem and false otherwise. the function copies a fixed size 2d array to a fixed size 1d array. the function uses the formulas listed in the problem to calculate 1d array indices from 2d array index pairs. Please fix/revise programbelow: #include using namespace std; const int NCOLS = 3; bool copy1DTo2D(int d1[], int size, int d2[][NCOLS], int nrows) { int i = 0, k, j = 0; //if if (size > 0 && NCOLS > 0 && nrows > 0 && size == NCOLS * nrows) { //copying for (k = 0;k < size;k++) { i = k / NCOLS; j = k % NCOLS; d2[i][j] = d1[k]; } return true; } return false; } bool copy2DTo1D(int d2[][NCOLS], int nrows, int d1[], int size) { int i, j, k; if (size > 0 && NCOLS > 0 && nrows > 0) { //copying for (i = 0;i < nrows;i++) { for (j = 0;j < NCOLS;j++) d1[NCOLS * i + j] = d2[i][j]; } return true; } return false; } int main() { int d1[6] = { 1,2,3,4,5,6 }; int d2[2][3]; //copying cout << "Function returned: " << copy1DTo2D(d1, 6, d2, 2) << endl; //printing 2d array for (int i = 0;i < 2;i++) { for (int j = 0;j < 3;j++) cout << d2[i][j] << " "; cout << endl; } //making all elements in d1 as zero d1[6] = { 0 }; //copting cout << "Function returned: " << copy2DTo1D(d2, 2, d1, 6) << endl; //printing d1 for (int i = 0;i < 6;i++) cout << d1[i] << " "; return 0; }
In C++,
Can you please look at the code below and revise/fix so it will work according to instructions and criteria.
Instruction
1) Write a function that copies a 1D array to a 2D array. The function’s prototype is
bool copy1DTo2D(int d1[], int size, int d2[][NCOLS], int nrows);
where
size > 0
NCOLS > 0
nrows > 0
NCOLS is a global constant
size = NCOLS * nrows
the function returns true if the parameters and constants satisfy these conditions and false otherwise.
the relation between 1d array indices and 2d array indices is
2d row index = 1d array index / NCOLS
2d column index = 1d array index modulus operator NCOLS
2) Write a function that copies a 2D array to a 1D array. The function’s prototype is
bool copy2DTo1D(int d2[][NCOLS, int nrows, int d1[], int size);
where
size > 0
NCOLS > 0
nrows > 0
NCOLS is a global constant
the function return true if the parameters and constants satisfy these conditions and false otherwise.
the relation between 1d array indices and 2d array indices is
1d array index = NCOLS x 2d array row index + 2d array column index
Criteria
compiles, runs, tests
the program compiles with error, runs without crashing, and tests the functions thoroughly
copy 1d to 2d function
the function returns true if the parameters and global constant satisfy list of the relationships and restrictions in the problem and false otherwise.
the function copies a fixed size 1d array to a fixed size2d array. the function uses the formulas listed in the problem to calculate 2d array index pairs from 1d array indices.
copy 2d to 1d function
the function returns true if the parameters and global constant satisfy list of the relationships and restrictions in the problem and false otherwise.
the function copies a fixed size 2d array to a fixed size 1d array. the function uses the formulas listed in the problem to calculate 1d array indices from 2d array index pairs.
Please fix/revise programbelow:
#include <iostream>
using namespace std;
const int NCOLS = 3;
bool copy1DTo2D(int d1[], int size, int d2[][NCOLS], int nrows)
{
int i = 0, k, j = 0;
//if
if (size > 0 && NCOLS > 0 && nrows > 0 && size == NCOLS * nrows)
{
//copying
for (k = 0;k < size;k++)
{
i = k / NCOLS;
j = k % NCOLS;
d2[i][j] = d1[k];
}
return true;
}
return false;
}
bool copy2DTo1D(int d2[][NCOLS], int nrows, int d1[], int size) {
int i, j, k;
if (size > 0 && NCOLS > 0 && nrows > 0)
{
//copying
for (i = 0;i < nrows;i++)
{
for (j = 0;j < NCOLS;j++)
d1[NCOLS * i + j] = d2[i][j];
}
return true;
}
return false;
}
int main()
{
int d1[6] = { 1,2,3,4,5,6 };
int d2[2][3];
//copying
cout << "Function returned: " << copy1DTo2D(d1, 6, d2, 2) << endl;
//printing 2d array
for (int i = 0;i < 2;i++) {
for (int j = 0;j < 3;j++)
cout << d2[i][j] << " ";
cout << endl;
}
//making all elements in d1 as zero
d1[6] = { 0 };
//copting
cout << "Function returned: " << copy2DTo1D(d2, 2, d1, 6) << endl;
//printing d1
for (int i = 0;i < 6;i++)
cout << d1[i] << " ";
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

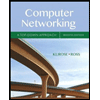
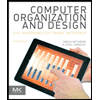
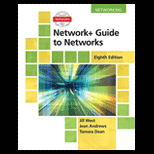
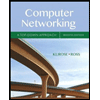
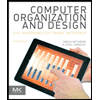
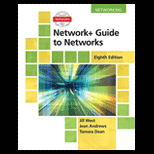
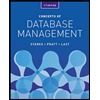
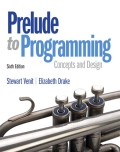
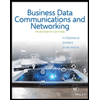