in c++ I have this working code to read a .dat file #include <iostream>#include <fstream>#include <string>using namespace std; //function declarationvoid calculations(float *hours, float *pay_hour, float *personal_tax_rate); int main(){//variable to store employee detailsstring ssn ,lastname, firstname;float hours, pay_hour, personal_tax_rate;//ifstream to read file named employee.datifstream myfile;myfile.open("employee.dat");//while loop to go through all the data in filewhile (myfile >> ssn >> lastname >> firstname >> hours >> pay_hour >> personal_tax_rate){//if condition to print eligible employees for overtimecout << ssn << " " << lastname << " " << firstname << " " << hours << " " << pay_hour << " " << personal_tax_rate << " ";calculations(&hours,&pay_hour,&personal_tax_rate);} //End of if} //End of while//End of main //function definitionvoid calculations(float *hours, float *pay_hour, float *personal_tax_rate){float reghours, overtime, reg_pay, over_pay, tax_rate;reghours = *hours;overtime=(*hours)-40.0;reg_pay=40.0*(*pay_hour);over_pay=1.5*(*pay_hour)*overtime;//print overtime hours, regular pay and overtime paycout << "\n";cout<<"Regular Hours"<< reghours<<endl;if (overtime>0){cout<<"Overtime: "<<overtime<<endl;}cout<<"Regular pay : "<<reg_pay<<endl;if (over_pay>0){cout<<"Overtime pay : "<<over_pay<<endl;} } I was wondering if there is a way where i can pass all the values of the void calculations() back to the main function and have it display in the main funtion?
in c++
I have this working code to read a .dat file
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
//function declaration
void calculations(float *hours, float *pay_hour, float *personal_tax_rate);
int main()
{
//variable to store employee details
string ssn ,lastname, firstname;
float hours, pay_hour, personal_tax_rate;
//ifstream to read file named employee.dat
ifstream myfile;
myfile.open("employee.dat");
//while loop to go through all the data in file
while (myfile >> ssn >> lastname >> firstname >> hours >> pay_hour >> personal_tax_rate)
{
//if condition to print eligible employees for overtime
cout << ssn << " " << lastname << " " << firstname << " " << hours << " " << pay_hour << " " << personal_tax_rate << " ";
calculations(&hours,&pay_hour,&personal_tax_rate);
} //End of if
} //End of while
//End of main
//function definition
void calculations(float *hours, float *pay_hour, float *personal_tax_rate)
{
float reghours, overtime, reg_pay, over_pay, tax_rate;
reghours = *hours;
overtime=(*hours)-40.0;
reg_pay=40.0*(*pay_hour);
over_pay=1.5*(*pay_hour)*overtime;
//print overtime hours, regular pay and overtime pay
cout << "\n";
cout<<"Regular Hours"<< reghours<<endl;
if (overtime>0)
{
cout<<"Overtime: "<<overtime<<endl;
}
cout<<"Regular pay : "<<reg_pay<<endl;
if (over_pay>0)
{
cout<<"Overtime pay : "<<over_pay<<endl;
}
}
I was wondering if there is a way where i can pass all the values of the void calculations() back to the main function and have it display in the main funtion?

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

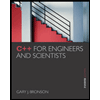
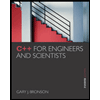