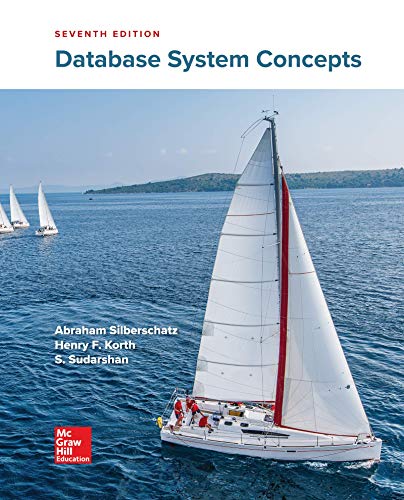
I need this code to be modular with function prototypes, definitions and call
#include<iostream>
#include<fstream>
#include<cstdlib>
using namespace std;
int main()
{
ifstream inFile;
inFile.open ("numbers.txt");
double highest;
double number;
if (inFile.fail())
{
cout << "Error opening file." <<endl;
exit (1);
}
double current ;
if( inFile >> current )
{
double highest = current;
while( inFile >> current )
{
if( current > highest ) highest = current;
}
cout << "The highest number is " << highest;
}
else
{
cout << "Failed to read any number from file!" <<endl;
return 1 ;
} }
the numbers in the file are:
78
85
2
45
12
99
65
9
15
65

Step by stepSolved in 2 steps with 1 images

- I need help making this C program which uses pointers. #include<stdio.h> //for printf and scanf #include<ctype.h> //for tolower function //function prototypes void Greeting(); //welcome the user to the gas station app void ViewAndGetSelection(char* selectionPtr); //input: the user's selection (input/output parameter) //display the program options and get the users selection //use an input/output parameter for the selection void ProcessSelection(char selection, double* balancePtr); //input: the user's selection by copy (input parameter) //input: the account balance (input/output parameter) //display a message that the selection has been entered //display the balance when the user enters 'b' //allow the user to add money to the account when the user enters 'u' int main() { char choiceInMain; double balanceInMain = 0.00; //call the greeting function //view and get the selection - function call //change the selection to lower or upper case //make sure the user did not enter q…arrow_forward#include#include#includeusing namespace std;// outputHtmlTitle// parameters// This function...void outputHtmlTitle(ofstream & fout, string title){fout << "" << endl;fout << "" << endl;fout << "" << endl;fout << "" << endl;fout << title << endl;fout << "" << endl;}void outputHtmlFooter(ofstream & fout){fout << "" << endl;fout << "" << endl;}void outputHtmlList(ostream & fout, string first, string second, string third){fout << "" << endl;fout << "\t" << first << "" << endl;fout << "\t" << second << "" << endl;fout << "\t" << third << "" << endl;fout << "\t" << endl;}void main(int argc, char * *argv){ofstream htmlFile("myIntro.html");string title;cout << "Please enter the title: ";getline(cin, title);outputHtmlTitle(htmlFile, title);string name;string course1, course2, course3;cout…arrow_forward#include <stdio.h>#include <stdlib.h> int cent50 = 0;int cent20 = 0;int cent10 = 0;int cent05 = 0; //Function definitionvoid calculateChange(int change) {if(change > 0) {if(change >= 50) {change -= 50;cent50++;} else if(change >= 20) {change -= 20;cent20++;} else if(change >= 10) {change -= 10;cent10++;} else if(change >= 05) {change -= 05;cent05++;}calculateChange(change);}} //Define the functionvoid printChange() { if(cent50)printf("\n50 Cents : %d coins", cent50); if(cent20)printf("\n20 Cents : %d coins", cent20); if(cent10)printf("\n10 Cents : %d coins", cent10); if(cent05)printf("\n05 Cents : %d coins", cent05);cent50 = 0;cent20 = 0;cent10 = 0;cent05 = 0; } //Function's definitionint TakeChange() { int change;printf("\nEnter the amount : ");scanf("%d", &change);return change; }//main functionint main() {//call the functionint change = TakeChange(); //use while-loop to repeatedly ask for input to the userwhile(change != -1){if((change %…arrow_forward
- C++languagearrow_forward#include <iostream> #include <cmath> using namespace std; // declare functions void display_menu(); void convert_temp(); double to_celsius(double fahrenheit); double to_fahrenheit(double celsius); int main() { cout << "Convert Temperatures\n\n"; display_menu(); char again = 'y'; while (again == 'y') { convert_temp(); cout << "Convert another temperature? (y/n): "; cin >> again; cout << endl; } cout << "Bye!\n"; } // define functions void display_menu() { cout << "MENU\n" << "1. Fahrenheit to Celsius\n" << "2. Celsius to Fahrenheit\n\n"; } void convert_temp() { int option; cout << "Enter a menu option: "; cin >> option; double f = 0.0; double c = 0.0; switch (option) { case 1: cout << "Enter degrees Fahrenheit: "; cin >> f; c =…arrow_forward#include <iostream> using namespace std; int rectArea (int len, int wid) { return len * wid; } int main () { int length, width; cin >> length >> width; cout << "The area of a " << length << " by " << width << " rectangle is " << /*add code to call the reacArea function */ << "." << endl; return 0; }arrow_forward
- Create a flowchart for this program in c++, #include <iostream>#include <vector> // for vectors#include <algorithm>#include <cmath> // math for function like pow ,sin, log#include <numeric>using std::vector;using namespace std;int main(){ vector <float> x, y;//vector x for x and y for y float x_tmp = -2.5; // initial value of x float my_function(float x); while (x_tmp <= 2.5) // the last value of x { x.push_back(x_tmp); y.push_back(my_function(x_tmp)); // calculate function's value for given x x_tmp += 1;// add step } cout << "my name's khaled , my variant is 21 ," << " my function is y = 0.05 * x^3 + 6sin(3x) + 4 " << endl; cout << "x\t"; for (auto x_tmp1 : x) cout << '\t' << x_tmp1;//printing x values with tops cout << endl; cout << "y\t"; for (auto y_tmp1 : y) cout << '\t' << y_tmp1;//printing y values with tops…arrow_forwardProbelm part 1: Define a function called common_divisors that takes two positive integers m and n as its arguments and returns the list of all common divisors (including 1) of the two integers, unless 1 is the only common divisor. If 1 is the only common divisor, the function should print a statement indicating the two numbers are relatively prime. Otherwise, the function prints the number of common divisors and the list of common divisors. Complete each to do with comments to expalin your process. Problem part 2: complete the following todo's and make comments to explain each step # TODO: Define the function 'common_divisors' with two arguments 'm' and 'n'. # TODO: Start with an empty list and append common divisors to the list. # TODO: Create a conditional statement to print the appropriate statement as indicated above. Problem part 3: Call the function common_divisors by passing 5 and 38. Your output should look like this: 5 and 38 are relatively prime. # TODO: Call…arrow_forward#include <iostream> #include <cmath> using namespace std; // declare functions void display_menu(); void convert_temp(); double to_celsius(double fahrenheit); double to_fahrenheit(double celsius); int main() { cout << "Convert Temperatures\n\n"; display_menu(); char again = 'y'; while (again == 'y') { convert_temp(); cout << "Convert another temperature? (y/n): "; cin >> again; cout << endl; } cout << "Bye!\n"; } // define functions void display_menu() { cout << "MENU\n" << "1. Fahrenheit to Celsius\n" << "2. Celsius to Fahrenheit\n\n"; } void convert_temp() { int option; cout << "Enter a menu option: "; cin >> option; double f = 0.0; double c = 0.0; switch (option) { case 1: cout << "Enter degrees Fahrenheit: "; cin >> f; c =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
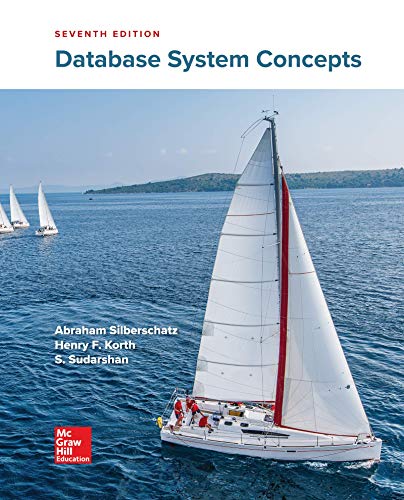
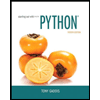
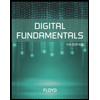
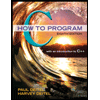
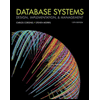
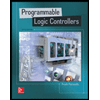