Write a program that implements four classes: NPC, Flying, Walking, and Generic for a fantasy roleplaying game. Each class should have the following attributes and methods: NPC -a parent class that defines methods and an attribute common to all non-player characters (npc) in the game. a private string variable named name, for storing the name of the npc. a default constructor for setting name to "placeholder". an overloaded constructor that sets name to a string argument passed to it. setName - a mutator for updating the name attribute getName - an accessor for returning the npc name printStats - a pure virtual function that will be overridden by each NPC subclass. Flying - a subclass of NPC that defines a flying npc in the game a private int variable named flightSpeed for tracking the speed of the npc. a default constructor for setting flightSpeed to 0 and name to "Flying" using setName. setFlightSpeed - a mutator that accepts an integer as it's only argument and updates flightSpeed. getFlightSpeed - an accessor that returns the flightSpeed. printStats - prints the name and current flightspeed to the screen as well as the string "Flying Monster". Walking - a subclass of NPC that defines a walking npc in the game a private int variable named walkSpeed for tracking the speed of the npc. a default constructor for setting walkSpeed to 0 and name to "Walking" using setName. setWalkSpeed - a mutator that accepts an integer as it's only argument and updates walkSpeed. getWalkSpeed - an accessor that returns the walkSpeed. printStats - prints the name and current walkSpeed to the screen as well as the string "Walking Monster". Generic - a subclass of NPC that defines a "generic" npc in the game a private int variable named stat for tracking some undetermined value. a default constructor for setting stat to 0 and name to "Generic" using setName. an overloaded constructor that accepts a string and an integer as it's only arguments. Sets stat to the integer argument and name to the string argument. setStat - a mutator that accepts an integer as it's only argument and updates stat. getStat - an accessor that returns the stat. printStats - prints the name and current stat to the screen as well as the string "Generic Monster". Demonstrate the classes in a program that has an array of NPC pointers. The array elements should be assigned the addresses of dynamically allocated Flying, Walking, and Generic objects. The program should then traverse the array, calling each object's printStats() method. Assign whatever values you wish to the attributes. Implement your classes using the following UML diagram. Do no use global variables. Ensure there are no memory leaks. Use only what we've covered in the course to write your solution. Classes have to be implemented using Uml design and This is required output of program
C++ Language Please
Write a program that implements four classes: NPC, Flying, Walking, and Generic for a fantasy roleplaying game. Each class should have the following attributes and methods:
- NPC -a parent class that defines methods and an attribute common to all non-player characters (npc) in the game.
- a private string variable named name, for storing the name of the npc.
- a default constructor for setting name to "placeholder".
- an overloaded constructor that sets name to a string argument passed to it.
- setName - a mutator for updating the name attribute
- getName - an accessor for returning the npc name
- printStats - a pure virtual function that will be overridden by each NPC subclass.
- Flying - a subclass of NPC that defines a flying npc in the game
- a private int variable named flightSpeed for tracking the speed of the npc.
- a default constructor for setting flightSpeed to 0 and name to "Flying" using setName.
- setFlightSpeed - a mutator that accepts an integer as it's only argument and updates flightSpeed.
- getFlightSpeed - an accessor that returns the flightSpeed.
- printStats - prints the name and current flightspeed to the screen as well as the string "Flying Monster".
- Walking - a subclass of NPC that defines a walking npc in the game
- a private int variable named walkSpeed for tracking the speed of the npc.
- a default constructor for setting walkSpeed to 0 and name to "Walking" using setName.
- setWalkSpeed - a mutator that accepts an integer as it's only argument and updates walkSpeed.
- getWalkSpeed - an accessor that returns the walkSpeed.
- printStats - prints the name and current walkSpeed to the screen as well as the string "Walking Monster".
- Generic - a subclass of NPC that defines a "generic" npc in the game
- a private int variable named stat for tracking some undetermined value.
- a default constructor for setting stat to 0 and name to "Generic" using setName.
- an overloaded constructor that accepts a string and an integer as it's only arguments. Sets stat to the integer argument and name to the string argument.
- setStat - a mutator that accepts an integer as it's only argument and updates stat.
- getStat - an accessor that returns the stat.
- printStats - prints the name and current stat to the screen as well as the string "Generic Monster".
Demonstrate the classes in a program that has an array of NPC pointers. The array elements should be assigned the addresses of dynamically allocated Flying, Walking, and Generic objects. The program should then traverse the array, calling each object's printStats() method. Assign whatever values you wish to the attributes.
Implement your classes using the following UML diagram. Do no use global variables. Ensure there are no memory leaks. Use only what we've covered in the course to write your solution.
Classes have to be implemented using Uml design and This is required output of program
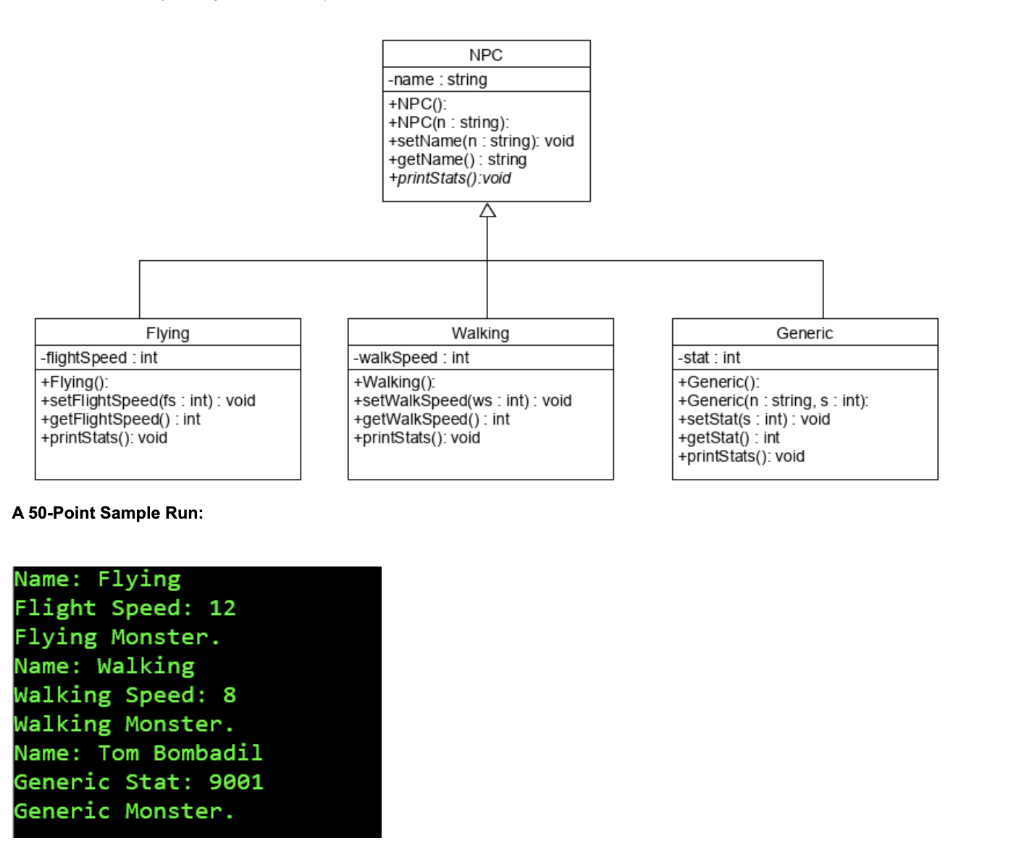

Trending now
This is a popular solution!
Step by step
Solved in 2 steps
