In Java, Question 15: Answer the following questions You are asked to Implement an ADT for MyQueue. The following is a class definition of a linked list Node: class Node { String content; Node next; } The following is a class definition of a linked list MyQueue: class MyQueue { Node head; } Implement the following methods for your class MyQueue. a) Constructor that does not requre any parameters b) Constructor that accepts a parameter of type MyQueue and creates a new instance of MyQueue that is a clone of the one passed as parameter c) public int AppendCopy method that accepts a single parameter of type MyQueue and clones all elements from the MyQueue instance passed as a parameter and appends them to the instance on which we called the method. Return the number of elements that are cloned and appended d) public int FindElements(String filter) - finds all elements that match the filter and returns their count e) overload the FindElementsmethod to include a bolean flag whether to remove all matching elements from the queue. If the flag is true, remove the elements from the queue. Give the flag parameter a meaningful name f) public void addToEnd() - add a node to the rear of the queue g) overload addToEnd method to include a value for the content of the newly created node. h) public Node removeFromFront() - remove an element from the front of the queue Hint: Some of the methods have overlapping functionality. Feel free to create utility methods and/or to work on these methods in a different order than what is given below and then reference the methods you already created in other steps. E.g., you may decide to implement AppendCopy and use it in another method.
In Java,
Question 15: Answer the following questions
You are asked to Implement an ADT for MyQueue.
The following is a class definition of a linked list Node:
class Node
{
String content;
Node next;
}
The following is a class definition of a linked list MyQueue:
class MyQueue
{
Node head;
}
Implement the following methods for your class MyQueue.
a) Constructor that does not requre any parameters
b) Constructor that accepts a parameter of type MyQueue and creates a new instance of MyQueue that is a clone of the one passed as parameter
c) public int AppendCopy method that accepts a single parameter of type MyQueue and clones all elements from the MyQueue instance passed as a parameter and appends them to the instance on which we called the method. Return the number of elements that are cloned and appended
d) public int FindElements(String filter) - finds all elements that match the filter and returns their count
e) overload the FindElementsmethod to include a bolean flag whether to remove all matching elements from the queue. If the flag is true, remove the elements from the queue. Give the flag parameter a meaningful name
f) public void addToEnd() - add a node to the rear of the queue
g) overload addToEnd method to include a value for the content of the newly created node.
h) public Node removeFromFront() - remove an element from the front of the queue
Hint: Some of the methods have overlapping functionality. Feel free to create utility methods and/or to work on these methods in a different order than what is given below and then reference the methods you already created in other steps. E.g., you may decide to implement AppendCopy and use it in another method.

Step by step
Solved in 2 steps with 2 images

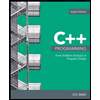
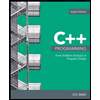