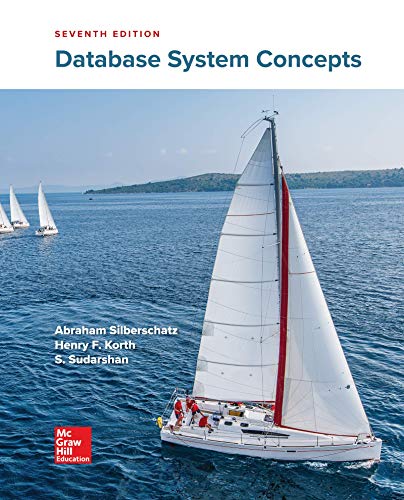
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
In c++, Thanks!!!
Write the simplest program that will demonstrate iteration vs recursion using the following guidelines -
- Write two primary helper functions - one iterative (IsArrayPrimeIter) and one recursive (IsArrayPrimeRecur) - each of which
- Take an array and its size as input params and return a bool such that
- 'true' ==> array and all elements are prime,
- 'false' ==> at least one element in array is not prime, so array is not prime.
- Print out a message "Entering <function_name>" as the first statement of each function.
- Perform the code to test whether every element of the array is a Prime number.
- Print out a message "Leaving <function_name>" as the last statement before returning from the function.
- Remember - there will be nested loops for the iterative function and there can be no loops at all in the recursive function. You will need to define one other helper function (IsPrimeRecur) for the recursion which should also not contain any loops to make it a true recursive method.
- Remember - replace <function_name> with the actual name of each function.
- Including your 'main', there will be a total of 4 functions in your program and only the primary helper functions can be invoked from 'main'. Note - for all functions except 'main', the 'Entering <function name>' and 'Leaving <function name'> statements should be printed.
- Hint - try to complete your iterative code first and then convert it piece by piece to the recursive code.
- Take an array and its size as input params and return a bool such that
- In your main:
- You can expect the user will input the number of elements for the array on one line, not to exceed SORT_MAX_SIZE = 16 (validate input).
- Create an array based on the size input provided by the user.
- On the next input line you can expect the user will provide all the array elements separated by spaces. Validate that the integers input by the user are between 1 and 9999, both inclusive. Remember: 1 is not a prime number.
- Make a call to the primary iterative function passing the array and its size.
- If every member is a Prime, then the program should print out 'Prime Array using iteration', otherwise print out 'Not a Prime Array using iteration'.
- Then make a call to the primary recursive function passing the array and its size.
- If every member is a Prime, then the program should print out 'Prime Array using recursion', otherwise print out 'Not a Prime Array using recursion'.
- If your functions are coded correctly, both should come up with the same answer, except you should have lots more output statements using recursion.
- There is no sample output - you are allowed to provide user interactivity as you see fit but programs will be graded for clarity of interaction.
- You can use language native arrays - DO NOT USE
VECTORS , COLLECTIONS, SETS, BAGS or any other data structures from yourprogramming language. - There will be only one code file in your submission.
- Remember to take multiple screenshots so that they are clearly readable without needing to zoom in.
- For documentation, include your name block as well pre/post and pseudocode for the 3 functions which are not 'main'. For pre/post documentation and pseudocode examples, see Design Tools and Documentation.pdf download
- Upload your code file and the screenshots in one zip file. Do not include anything else.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Modify the recursive Fibonacci function to employ the memoization technique discussed in this chapter. The function creates a dictionary and then defines a nested recursive helper function named memoizedFib. You will need to create a dictionary to cache the sum of the fib function. The base case of the fib function is the same as before. However, before making any recursive calls, the helper function looks up the value for the function’s current argument in the dictionary (use the method get, with None as the default value). If the value exists, the function returns it and skips any recursive calls. Otherwise, after the helper function adds the results of its two recursive calls, it saves the sum in the dictionary with the current argument of the function as the key. Note: The program should output in the following format: n fib(n) 2 1 4 3 8 21 16 987 32 2178309 ---------------------------------------------------------------------------------- def…arrow_forwardQuestion: First write a function mulByDigit :: Int -> BigInt -> BigInt which takes an integer digit and a big integer, and returns the big integer list which is the result of multiplying the big integer with the digit. You should get the following behavior: ghci> mulByDigit 9 [9,9,9,9] [8,9,9,9,1] Your implementation should not be recursive. Now, using mulByDigit, fill in the implementation of bigMul :: BigInt -> BigInt -> BigInt Again, you have to fill in implementations for f , base , args only. Once you are done, you should get the following behavior at the prompt: ghci> bigMul [9,9,9,9] [9,9,9,9] [9,9,9,8,0,0,0,1] ghci> bigMul [9,9,9,9,9] [9,9,9,9,9] [9,9,9,9,8,0,0,0,0,1] ghci> bigMul [4,3,7,2] [1,6,3,2,9] [7,1,3,9,0,3,8,8] ghci> bigMul [9,9,9,9] [0] [] Your implementation should not be recursive. Code: import Prelude hiding (replicate, sum)import Data.List (foldl') foldLeft :: (a -> b -> a) -> a -> [b] -> afoldLeft =…arrow_forwardWrite a RECURSIVE function, without using any loops, that prints the contents of a matrix with 3 columns. The function should take the matrix and the number of rows as arguments. void print_matrix(int arr[][3], int num_rows);arrow_forward
- 1. The sorted values array contains 16 integers 5, 7, 10, 13, 13, 20, 21, 25, 30,32, 40, 45, 50, 52, 57, 60. Indicate the sequence of recursive calls that are made tobinaraySearch, given an initial invocation of binarySearch(32, 0, 15).show only the recursive calls. For example, initial invocation is binarySearch(45,0,15)where the target is 45, first is 0 and last is 15.arrow_forwarddo it in c++ i need it fast in 10 minutesarrow_forwardData Structures and Algorithms in C/C++ a) Implement the addLargeNumbers function with the following prototype: void addLargeNumbers(const char *pNum1, const char *pNum2); This function should output the result of adding the two numbers passed in as strings. Here is an example call to this function with the expected output: /* Sample call to addLargeNumbers */ addLargeNumbers("592", "3784"); /* Expected output */ 4376 b) Implement a test program that demonstrates adding at least three pairs of large numbers (numbers larger than can be represented by a long). c) (1 point) Make sure your source code is well-commented, consistently formatted, uses no magic numbers/values, follows programming best-practices, and is ANSI-compliant.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
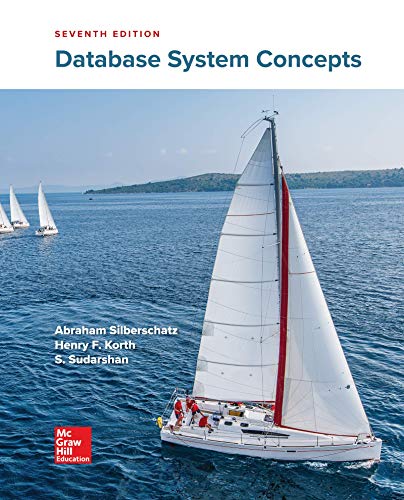
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
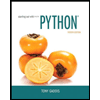
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
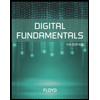
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
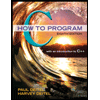
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
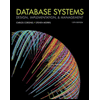
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
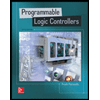
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education