IN SCALA COULD YOU COMPLETE THE FUNCTIONS // type of tokens type Toks = List[String] // the operations in the basic version of the algorithm val ops = List("+", "-", "*", "/") // the precedences of the operators val precs = Map("+" -> 1, "-" -> 1, "*" -> 2, "/" -> 2) // helper function for splitting strings into tokens def split(s: String) : Toks = s.split(" ").toList // (1) Implement below the shunting yard algorithm. The most // convenient way to this in Scala is to implement a recursive // function and to heavily use pattern matching. The function syard // takes some input tokens as first argument. The second and third // arguments represent the stack and the output of the shunting yard // algorithm. // // In the marking, you can assume the function is called only with // an empty stack and an empty output list. You can also assume the // input os only properly formatted (infix) arithmetic expressions // (all parentheses will be well-nested, the input only contains // operators and numbers). // You can implement any additional helper function you need. I found // it helpful to implement two auxiliary functions for the pattern matching: // def is_op(op: String) : Boolean = ??? def prec(op1: String, op2: String) : Boolean = ??? def syard(toks: Toks, st: Toks = Nil, out: Toks = Nil) : Toks = ??? // test cases //syard(split("3 + 4 * ( 2 - 1 )")) // 3 4 2 1 - * + //syard(split("10 + 12 * 33")) // 10 12 33 * + //syard(split("( 5 + 7 ) * 2")) // 5 7 + 2 * //syard(split("5 + 7 / 2")) // 5 7 2 / + //syard(split("5 * 7 / 2")) // 5 7 * 2 / //syard(split("9 + 24 / ( 7 - 3 )")) // 9 24 7 3 - / + //syard(split("3 + 4 + 5")) // 3 4 + 5 + //syard(split("( ( 3 + 4 ) + 5 )")) // 3 4 + 5 + //syard(split("( 3 + ( 4 + 5 ) )")) // 3 4 5 + + //syard(split("( ( ( 3 ) ) + ( ( 4 + ( 5 ) ) ) )")) // 3 4 5 + + // (2) Implement a compute function that evaluates an input list // in postfix notation. This function takes a list of tokens // and a stack as argumenta. The function should produce the // result as an integer using the stack. You can assume // this function will be only called with proper postfix // expressions. def compute(toks: Toks, st: List[Int] = Nil) : Int = ??? // test cases // compute(syard(split("3 + 4 * ( 2 - 1 )"))) // 7 // compute(syard(split("10 + 12 * 33"))) // 406 // compute(syard(split("( 5 + 7 ) * 2"))) // 24 // compute(syard(split("5 + 7 / 2"))) // 8 // compute(syard(split("5 * 7 / 2"))) // 17 // compute(syard(split("9 + 24 / ( 7 - 3 )"))) // 15
IN SCALA COULD YOU COMPLETE THE FUNCTIONS // type of tokens type Toks = List[String] // the operations in the basic version of the algorithm val ops = List("+", "-", "*", "/") // the precedences of the operators val precs = Map("+" -> 1, "-" -> 1, "*" -> 2, "/" -> 2) // helper function for splitting strings into tokens def split(s: String) : Toks = s.split(" ").toList // (1) Implement below the shunting yard algorithm. The most // convenient way to this in Scala is to implement a recursive // function and to heavily use pattern matching. The function syard // takes some input tokens as first argument. The second and third // arguments represent the stack and the output of the shunting yard // algorithm. // // In the marking, you can assume the function is called only with // an empty stack and an empty output list. You can also assume the // input os only properly formatted (infix) arithmetic expressions // (all parentheses will be well-nested, the input only contains // operators and numbers). // You can implement any additional helper function you need. I found // it helpful to implement two auxiliary functions for the pattern matching: // def is_op(op: String) : Boolean = ??? def prec(op1: String, op2: String) : Boolean = ??? def syard(toks: Toks, st: Toks = Nil, out: Toks = Nil) : Toks = ??? // test cases //syard(split("3 + 4 * ( 2 - 1 )")) // 3 4 2 1 - * + //syard(split("10 + 12 * 33")) // 10 12 33 * + //syard(split("( 5 + 7 ) * 2")) // 5 7 + 2 * //syard(split("5 + 7 / 2")) // 5 7 2 / + //syard(split("5 * 7 / 2")) // 5 7 * 2 / //syard(split("9 + 24 / ( 7 - 3 )")) // 9 24 7 3 - / + //syard(split("3 + 4 + 5")) // 3 4 + 5 + //syard(split("( ( 3 + 4 ) + 5 )")) // 3 4 + 5 + //syard(split("( 3 + ( 4 + 5 ) )")) // 3 4 5 + + //syard(split("( ( ( 3 ) ) + ( ( 4 + ( 5 ) ) ) )")) // 3 4 5 + + // (2) Implement a compute function that evaluates an input list // in postfix notation. This function takes a list of tokens // and a stack as argumenta. The function should produce the // result as an integer using the stack. You can assume // this function will be only called with proper postfix // expressions. def compute(toks: Toks, st: List[Int] = Nil) : Int = ??? // test cases // compute(syard(split("3 + 4 * ( 2 - 1 )"))) // 7 // compute(syard(split("10 + 12 * 33"))) // 406 // compute(syard(split("( 5 + 7 ) * 2"))) // 24 // compute(syard(split("5 + 7 / 2"))) // 8 // compute(syard(split("5 * 7 / 2"))) // 17 // compute(syard(split("9 + 24 / ( 7 - 3 )"))) // 15
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
IN SCALA COULD YOU COMPLETE THE FUNCTIONS
// type of tokens
type Toks = List[String]
// the operations in the basic version of the algorithm
val ops = List("+", "-", "*", "/")
// the precedences of the operators
val precs = Map("+" -> 1,
"-" -> 1,
"*" -> 2,
"/" -> 2)
// helper function for splitting strings into tokens
def split(s: String) : Toks = s.split(" ").toList
// (1) Implement below the shunting yard algorithm. The most
// convenient way to this in Scala is to implement a recursive
// function and to heavily use pattern matching. The function syard
// takes some input tokens as first argument. The second and third
// arguments represent the stack and the output of the shunting yard
// algorithm.
//
// In the marking, you can assume the function is called only with
// an empty stack and an empty output list. You can also assume the
// input os only properly formatted (infix) arithmetic expressions
// (all parentheses will be well-nested, the input only contains
// operators and numbers).
// You can implement any additional helper function you need. I found
// it helpful to implement two auxiliary functions for the pattern matching:
//
def is_op(op: String) : Boolean = ???
def prec(op1: String, op2: String) : Boolean = ???
def syard(toks: Toks, st: Toks = Nil, out: Toks = Nil) : Toks = ???
// test cases
//syard(split("3 + 4 * ( 2 - 1 )")) // 3 4 2 1 - * +
//syard(split("10 + 12 * 33")) // 10 12 33 * +
//syard(split("( 5 + 7 ) * 2")) // 5 7 + 2 *
//syard(split("5 + 7 / 2")) // 5 7 2 / +
//syard(split("5 * 7 / 2")) // 5 7 * 2 /
//syard(split("9 + 24 / ( 7 - 3 )")) // 9 24 7 3 - / +
//syard(split("3 + 4 + 5")) // 3 4 + 5 +
//syard(split("( ( 3 + 4 ) + 5 )")) // 3 4 + 5 +
//syard(split("( 3 + ( 4 + 5 ) )")) // 3 4 5 + +
//syard(split("( ( ( 3 ) ) + ( ( 4 + ( 5 ) ) ) )")) // 3 4 5 + +
// (2) Implement a compute function that evaluates an input list
// in postfix notation. This function takes a list of tokens
// and a stack as argumenta. The function should produce the
// result as an integer using the stack. You can assume
// this function will be only called with proper postfix
// expressions.
def compute(toks: Toks, st: List[Int] = Nil) : Int = ???
// test cases
// compute(syard(split("3 + 4 * ( 2 - 1 )"))) // 7
// compute(syard(split("10 + 12 * 33"))) // 406
// compute(syard(split("( 5 + 7 ) * 2"))) // 24
// compute(syard(split("5 + 7 / 2"))) // 8
// compute(syard(split("5 * 7 / 2"))) // 17
// compute(syard(split("9 + 24 / ( 7 - 3 )"))) // 15
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
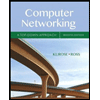
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
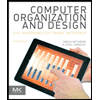
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
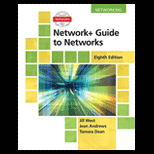
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
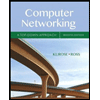
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
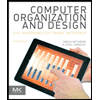
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
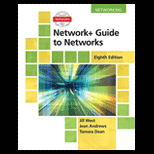
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
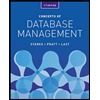
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
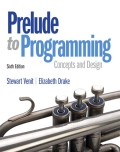
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
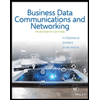
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY