Write a Python function `removeDuplicates(myList)` that removes duplicate values from a list. While the algorithm for this problem might seem trivial, its implementation is not. In general, it is not advisable to modify a list while you are iterating on it, as in the following pseudo-code: for element in myList: if : # recipe for disaster
Write a Python function `removeDuplicates(myList)` that removes duplicate values from a list. While the algorithm for this problem might seem trivial, its implementation is not. In general, it is not advisable to modify a list while you are iterating on it, as in the following pseudo-code: for element in myList: if : # recipe for disaster
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Write a Python function `removeDuplicates(myList)` that removes duplicate values from a list. While the
for element in myList:
if <condition>:
<remove element(s) from myList> # recipe for disaster
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
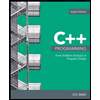
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
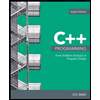
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning