IN SCALA PLEASE COULD YOU COMPLETE THE FUNCTIONS: is_pow_of_two, is_hard, last_odd //(1) Complete the collatz function below. It should // recursively calculate the number of steps needed // until the collatz series reaches the number 1. // If needed, you can use an auxiliary function that // performs the recursion. The function should expect // arguments in the range of 1 to 1 Million. def collatz(n: Long) : Long = { if(n == 1) 0else if(n % 2 == 0) 1 + collatz(n/2) else1 + collatz(n*3 + 1) } //(2) Complete the collatz_max function below. It should // calculate how many steps are needed for each number // from 1 up to a bound and then calculate the maximum number of // steps and the corresponding number that needs that many // steps. Again, you should expect bounds in the range of 1 // up to 1 Million. The first component of the pair is // the maximum number of steps and the second is the // corresponding number. def collatz_max(bnd: Long) : (Long, Long) = { ((1.toLong to bnd).toList.map(n => collatz(n)).max, (1.toLong to bnd).toList.map(n => collatz(n)).indexOf((1.toLong to bnd).toList.map(n => collatz(n)).max) + 1) } //(3) Implement a function that calculates the last_odd // number in a collatz series. For this implement an // is_pow_of_two function which tests whether a number // is a power of two. The function is_hard calculates // whether 3n + 1 is a power of two. Again you can // assume the input ranges between 1 and 1 Million, // and also assume that the input of last_odd will not // be a power of 2. def is_pow_of_two(n: Long) : Boolean = ??? def is_hard(n: Long) : Boolean = ??? def last_odd(n: Long) : Long = ???
IN SCALA PLEASE COULD YOU COMPLETE THE FUNCTIONS: is_pow_of_two, is_hard, last_odd //(1) Complete the collatz function below. It should // recursively calculate the number of steps needed // until the collatz series reaches the number 1. // If needed, you can use an auxiliary function that // performs the recursion. The function should expect // arguments in the range of 1 to 1 Million. def collatz(n: Long) : Long = { if(n == 1) 0else if(n % 2 == 0) 1 + collatz(n/2) else1 + collatz(n*3 + 1) } //(2) Complete the collatz_max function below. It should // calculate how many steps are needed for each number // from 1 up to a bound and then calculate the maximum number of // steps and the corresponding number that needs that many // steps. Again, you should expect bounds in the range of 1 // up to 1 Million. The first component of the pair is // the maximum number of steps and the second is the // corresponding number. def collatz_max(bnd: Long) : (Long, Long) = { ((1.toLong to bnd).toList.map(n => collatz(n)).max, (1.toLong to bnd).toList.map(n => collatz(n)).indexOf((1.toLong to bnd).toList.map(n => collatz(n)).max) + 1) } //(3) Implement a function that calculates the last_odd // number in a collatz series. For this implement an // is_pow_of_two function which tests whether a number // is a power of two. The function is_hard calculates // whether 3n + 1 is a power of two. Again you can // assume the input ranges between 1 and 1 Million, // and also assume that the input of last_odd will not // be a power of 2. def is_pow_of_two(n: Long) : Boolean = ??? def is_hard(n: Long) : Boolean = ??? def last_odd(n: Long) : Long = ???
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.1: Function And Parameter Declarations
Problem 10E
Related questions
Question
IN SCALA PLEASE
COULD YOU COMPLETE THE FUNCTIONS: is_pow_of_two, is_hard, last_odd
//(1) Complete the collatz function below. It should
// recursively calculate the number of steps needed
// until the collatz series reaches the number 1.
// If needed, you can use an auxiliary function that
// performs the recursion. The function should expect
// arguments in the range of 1 to 1 Million.
def collatz(n: Long) : Long = {
if(n == 1) 0else
if(n % 2 == 0) 1 + collatz(n/2) else1 + collatz(n*3 + 1)
}
//(2) Complete the collatz_max function below. It should
// calculate how many steps are needed for each number
// from 1 up to a bound and then calculate the maximum number of
// steps and the corresponding number that needs that many
// steps. Again, you should expect bounds in the range of 1
// up to 1 Million. The first component of the pair is
// the maximum number of steps and the second is the
// corresponding number.
def collatz_max(bnd: Long) : (Long, Long) = {
((1.toLong to bnd).toList.map(n => collatz(n)).max, (1.toLong to bnd).toList.map(n => collatz(n)).indexOf((1.toLong to bnd).toList.map(n => collatz(n)).max) + 1)
}
//(3) Implement a function that calculates the last_odd
// number in a collatz series. For this implement an
// is_pow_of_two function which tests whether a number
// is a power of two. The function is_hard calculates
// whether 3n + 1 is a power of two. Again you can
// assume the input ranges between 1 and 1 Million,
// and also assume that the input of last_odd will not
// be a power of 2.
def is_pow_of_two(n: Long) : Boolean = ???
def is_hard(n: Long) : Boolean = ???
def last_odd(n: Long) : Long = ???
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
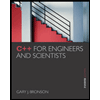
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
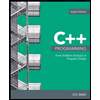
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
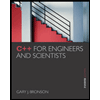
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
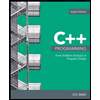
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning