In the code editor, you are provided with the definition of a struct Dog. This struct needs a character value for its gender, which could either be 'm' for a male Dog or 'f' for a female Dog. Furthermore, an array of Dogs has already been created an each of their genders has been set. Then, on line 16, a call to the function giveDogs() was made where the dogs array was passed. Your task is to implement this giveDogs() function which has the following details: Return type - void Name - giveDogs Parameters a pointer/holder of a Dogs array size of the Dogs array Description - loops through the Dogs array and prints the correct message (refer to the sample output)
In the code editor, you are provided with the definition of a struct Dog. This struct needs a character value for its gender, which could either be 'm' for a male Dog or 'f' for a female Dog. Furthermore, an array of Dogs has already been created an each of their genders has been set. Then, on line 16, a call to the function giveDogs() was made where the dogs array was passed. Your task is to implement this giveDogs() function which has the following details: Return type - void Name - giveDogs Parameters a pointer/holder of a Dogs array size of the Dogs array Description - loops through the Dogs array and prints the correct message (refer to the sample output)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need a C code of this ASAP please.
Instructions:
- In the code editor, you are provided with the definition of a struct Dog. This struct needs a character value for its gender, which could either be 'm' for a male Dog or 'f' for a female Dog.
- Furthermore, an array of Dogs has already been created an each of their genders has been set. Then, on line 16, a call to the function giveDogs() was made where the dogs array was passed.
-
Your task is to implement this giveDogs() function which has the following details:
- Return type - void
- Name - giveDogs
-
Parameters
- a pointer/holder of a Dogs array
- size of the Dogs array
- Description - loops through the Dogs array and prints the correct message (refer to the sample output)
Main code:
#include<stdio.h>
typedef struct {
char gender;
} Dog;
void giveDogs(Dog*, int);
int main(void) {
Dog dogs[100];
for(int i = 0; i < 100; i++) {
dogs[i].gender = i % 2 == 0 ? 'm' : 'f';
}
giveDogs(dogs, 100);
return 0;
}
void giveDogs(Dog *dogs, int size) {
// TODO: Implement this
}
Output should be:
Output
Note that the dog number starts at 1, not 0.
Dog·#1·(gender·=·m)
Dog·#2·(gender·=·f)
Dog·#3·(gender·=·m)
Dog·#4·(gender·=·f)
Dog·#5·(gender·=·m)
Dog·#6·(gender·=·f)
Dog·#7·(gender·=·m)
Dog·#8·(gender·=·f)
Dog·#9·(gender·=·m)
Dog·#10·(gender·=·f)
Dog·#11·(gender·=·m)
Dog·#12·(gender·=·f)
Dog·#13·(gender·=·m)
.
.
.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
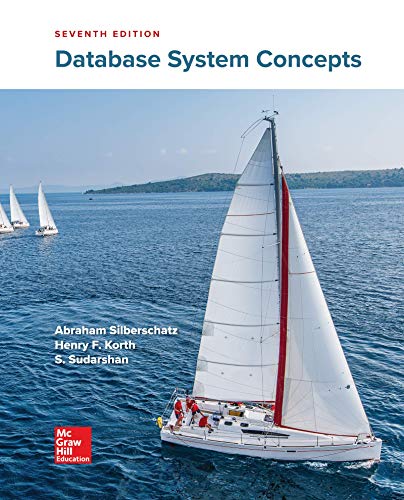
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
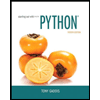
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
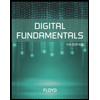
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
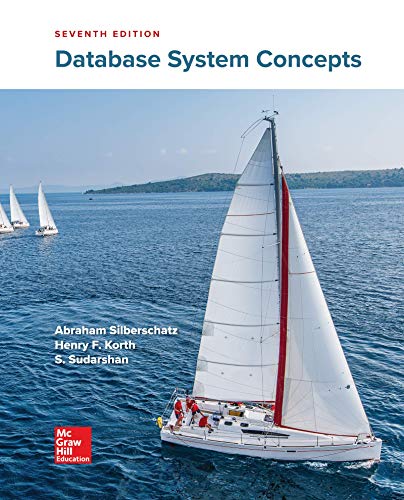
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
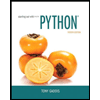
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
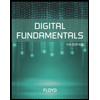
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
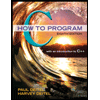
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
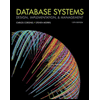
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
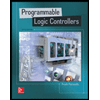
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education