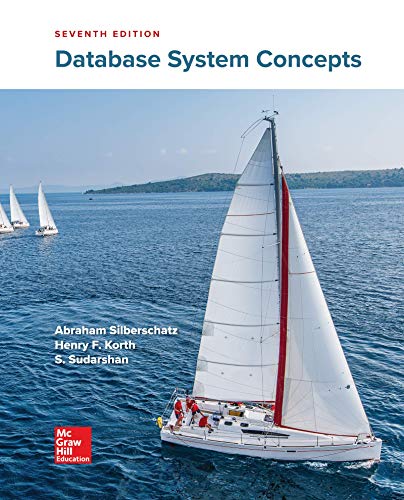
Concept explainers
I need a C code for this.
Instructions:
- In the code editor, you are provided with a function, findElephant() that accepts a 2D array and returns the largest element in the array. This function has already been implemented so do not edit this.
- You are also provided with the 2D array in the main() function.
- Your only task is to call the findElephant() function and pass the 2D array, and print the return value.
This is the current code:
#include<stdio.h>
#include<limits.h>
int findElephant(int[][100]);
int main(void) {
int matrix[100][100] = {
{1, 3, 33, 109, 2, 65, 32, 28, 129, 75},
{2, 44, 59, 8, 231, 632, 55, 205, 98, 1},
{76, 2, 321, 16, 209, 65, 12, 167, 259, 29},
{0, 103, 99, 3, 2, 65, 32, 321, 53, 75},
{44, 264, 50, 7, 142, 65, 100, 28, 98, 33},
{87, 22, 99, 67, 22, 412, 99, 502, 52, 24},
{91, 93, 233, 101, 330, 65, 98, 28, 98, 76},
{12, 65, 59, 99, 2, 65, 32, 333, 10, 88},
{3, 24, 101, 331, 542, 65, 32, 239, 338, 175},
{76, 3, 59, 21, 229, 65, 32, 28, 98, 75},
};
return 0;
}
// NOTE: DO NOT EDIT THIS FUNCTION
int findElephant(int arr[][100]) {
int largest = INT_MIN;
for(int row = 0; row < 100; row++) {
for(int col = 0; col < 100; col++) {
if(arr[row][col] > largest) {
largest = arr[row][col];
}
}
}
return largest;
}
The output should be:
Elephant: 10

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Java code Screenshot and output is mustarrow_forwardHow do you put a 2D array code into a function? (C++ only)arrow_forwardIn C++ please follow the instructions Write two code blocks -- one code block to declare a bag and its companion type-tracking array (both using the STL vector), and another code block to create and declare a Cat object and put it into the bag. Name the arrays and variables as you wish. Use any data type tracking and any designation for cats -- your choices. Assume that struct Cat is already defined and that all required libraries are properly included -- just write the two separate code blocks, separated by one or more blank lines.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
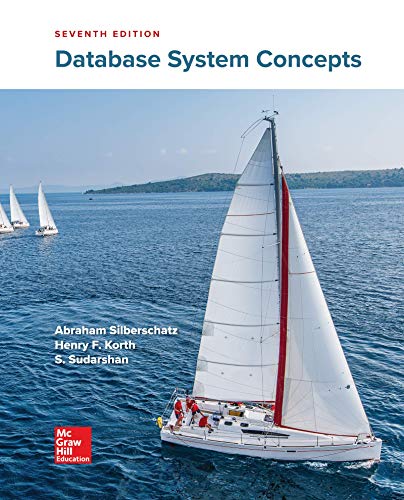
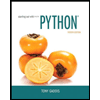
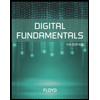
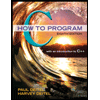
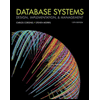
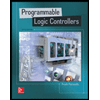