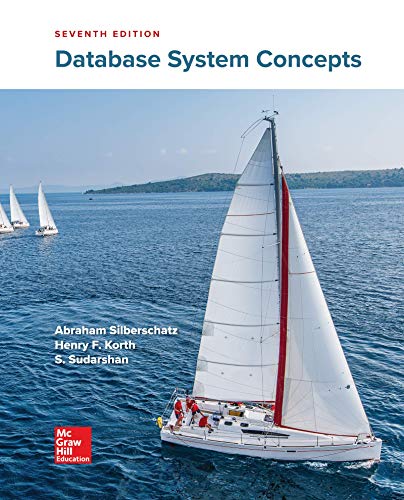
In C++, create a class for a 3-dimensional array. To make it more general, allow the actual dimensionality to be 0d, 1d, 2d, or 3d. It should support indexing with operator(). If the programmer subscripts with fewer indices than you 'need' (0 for a 1d, 0 or 1 for a 2d, 0 or 1 or 2 for a 3d -- note that you can't under-subscript a 0d array), you should return the appropriately sized sub-array (of your class's type). (Note: This will require multiple overloads of operator() with different numbers of index arguments.)
Since, as a template, math operations don't make sense, you'll have to for-go addition, etc. But you could use + and += for concatenation. Basically aligning the common dimensions of the two arrays and concatenating along the remaining dimension. (There can be only one for this to make sense. If there are none remaining, there are options for those kinds of things!) Make sure to overload it for proper dimensionality:
Of course, you should also support input (the programmer must set the dimensions ahead of time) and output (make it look nice like those above) and fun operations like transpose (operator~? but what is a 3d transpose...*ick*).
And how do you exactly store a dynamic array of more than one dimension? Well, the easiest way is to create a 1d array of all the items and then map the 3d subscripts into 1d:
actual dimensions:
x,y,z 3dX[i][j][k] --> 1dX[i*y*z+j*z+k]
Write a driver to show off your 3d (or less) class' abilities.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Write a program that stores dynamically allocated array of Movie (using calloc() function). Movie is the Structure with seven fields: name, genre (science fiction – action – drama/love), ticketPrices, list of actors/actresses, ratings(number of tickets sold), cost of production and profit. In program implementation user enters the following: Movie Name Movie1 Movie2 Movie3 Genre Science Fiction Action Drama-Love List of Actors /Actresses Ratings(number of tickets sold) 720 750 300 Ticket Prices $45.3 $50.2 $2.3 Cost of production $5000.5 $7000.1 $100 Net Profit - Print the Movie names, list of actors/actresses, ratings(number of tickets sold), ticket prices, cost of production and the total net profit of each movie(net_profit_of_movie) - Write a function that calculates the net_profit_of_movie; which is the multiplication of ticket prices and…arrow_forwardAn attacker has been successfully modifying the purchase price of items purchased on the company's web site. The security administrators verify the web server and Oracle database have not been compromised directly. They have also verified the IDS logs and found no attacks that could have caused this. What is the mostly likely way the attacker has been able to modify the purchase price? Select one: a. By using SQL Injection b. By using remote file inclusion c. By changing hidden values d. By using directory traversal attackarrow_forwardIn C++ code. Create a class called publication that stores the title (char array) and price (float) of a publication. From this class derive two classes: book, which adds a page count (int) and tape, which adds a playing time in minutes (float). Each of the three classes should have a getdata() function to get its data from the user using input from the keyboard and a putdata() function todisplay the data. Write a main function that creates an array of pointers to publication. In a loop, ask the user for data about a particular type of book or tape to hold data. Put the pointer to the object in the array. When the user has finished entering data for all the books and tapes, display the resulting data for all the books and tapes entered, using a for loop and a single statement such as: pubarr[j] -> putdata(); To display the data from each object in the array.arrow_forward
- Help with writing a program a C program implements a bubble sort algorith on an array of integers, and use command line paramameters to populate an array with data. The program should follow below guidelines if possible: If there are no command-line arguments at all when the program is run, the program should print out instructions on its use (a "usage message"). There should be one common usage message (consider a method/function for printing the usage message) for any type of usage error. The program will accept an A or D as the second command line argument (after the program name). This letter will tell you whether the bubble sort should sort in ascending or descending fashion. Anything other than A or D in that position should display the usage message and terminate the program. The program will be able to accept up to 32 numbers (integers) on the command line. If there are more than 32 numbers on the command line, or no numbers at all, the program should print out the usage…arrow_forward1. Given the declaration Circle[] x = new Circle[10], which of the following statement is most accurate? A. X contains an array of ten int values. B. x contains an array of ten objects of the Circle type. C. x contains a reference to an array and each element in the array can hold a reference to a Circle object. D. x contains a reference to an array and each element in the array can hold a Circle object. 2. Suppose s1 and s2 are two strings. Which of the following statements or expressions are incorrect? (3 A. int i 51.length(): B. boolean b =51.compareTo(s2); C. String s3 = s1 + 52; D. s1.charAt(0) = '5'; E. char c s1[0]; F. char c s1.charAt(s1.length0):arrow_forwardWrite a C++ program that computes for the sum, difference, product, and quotient of two floating-point numbers with two decimal places in the quotient.arrow_forward
- You are working for a university to maintain a list of grades and some related statistics for a student. Class and Data members: Create a class called Student that stores a student’s grades (integers) in a vector (do not use an array). The class should have data members that store a student’s name and the course for which the grades are earned. Constructor(s): The class should have a 2-argument constructor that receives the student’s name and course as parameters and sets the appropriate data members to these values. Member Functions: The class should have functions as follows: Member functions to set and get the student’s name and course variables. A member function that adds a single grade to the vector. Only positive grades are allowed. Call this function AddGrade. A member function to sort the vector in ascending order. A member function to compute the average (x̄) of the grades in the vector. The formula for calculating an average is x̄ = ∑xi / n where xi is the value of each…arrow_forwardWritten in Python with docstring please if applicable Thank youarrow_forwardWrite a simple trivia quiz game using c++ Start by creating a Trivia class that contains information about a single trivia question. The class should contain a string for the question, a string for the answer to the question, and an integer representing the dollar amount the question is worth (harder questions should be worth more). Add appropriate constructor and accessor functions. In your main function create either an array or a vector of type Trivia and hard-code at least five trivia questions of your choice. Your program should then ask each question to the player, input the player’s answer, and check if the player’s answer matches the actual answer. If so, award the player the dollar amount for that question. If the player enters the wrong answer your program should display the correct answer. When all questions have been asked display the total amount that the player has won.arrow_forward
- use c++ programming language to create a derived, or child class for Cylinder, that is, a Cone class. The same function, with the same parameters, is used. However, the formula is different for a cone. // The formula is: V = (1/3) * pi * (r^2) * harrow_forwardIn C# Use a one-dimensional array to solve the following problem. Write a class called ScoreFinder. This class receives a single dimensional integer array representing passer ratings for NFL players as an argument for its constructor. The test class will provides this argument. The test class then calls upon a method from the ScoreFinder class to start the process of finding a specific rating, which will be provided by the user. The method will iterate through the array and find any matching values. For all the matches that are found, the method will note the index of the cell along with its value. At completion, the method will print a list of all the indices and the associated scores along with a count of all the matching values.arrow_forwardWrite a program that will sort a prmiitive array of data using the following guidelines - DO NOT USE VECTORS, COLLECTIONS, SETS or any other data structures from your programming language. The codes for both the money and currency should stay the same, the main focus should be on the main file code (Programming language Java) Create a helper function called 'RecurInsSort' such that: It is a standalone function not part of any class from the prior lab or any new class you feel like creating here, Takes in the same type of parameters as any standard Insertion Sort with recursion behavior, i.e. void RecurInsSort(Currency arr[], int size) Prints out how the array looks every time a recursive step returns back to its caller The objects in the array should be Money objects added or manipulated using Currency references/pointers. It is OK to print out the array partially when returning from a particular step as long as the process of sorting is clearly demonstrated in the output. In…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
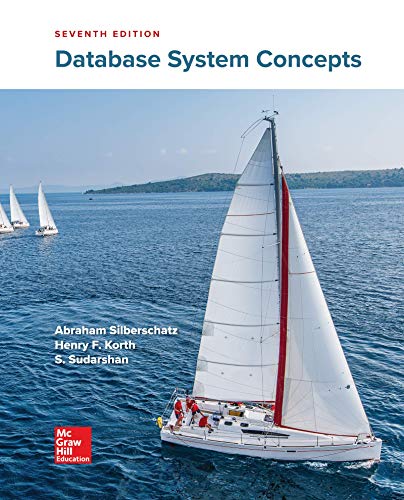
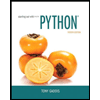
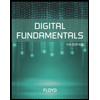
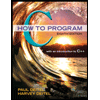
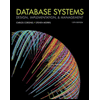
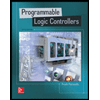