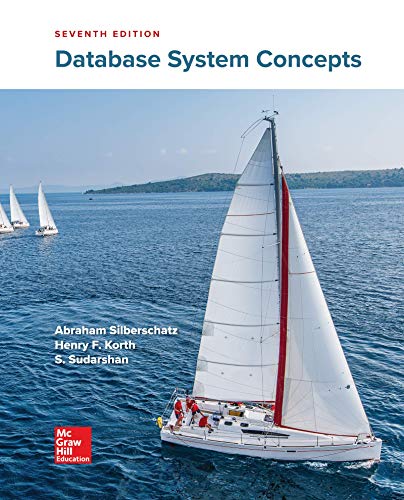
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
This is for Java
In my code, I'm missing a new line at the end of an array. I'm not sure how to do this. I get the correct output but am missing a newline at the end. Please see attached image for clarity. My code is below.
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int[] array=new int[20];
int n;
int a;
int i;
n=scnr.nextInt();
for(i = 0; i < n; i++)
array[i]=scnr.nextInt();
a=scnr.nextInt();
for(i = 0; i < n; i++)
{
if(array[i]<=a)
System.out.print(array[i] + " ");
}
}
}
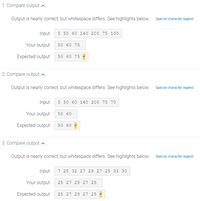
Transcribed Image Text:1: Compare output a
Output is nearly correct; but whitespace differs. See highlights below. Special character legend
Input
5 50 60 140 200 75 100
Your output
50 60 75
Expected output
50 60 75 e
2: Compare output a
Output is nearly correct; but whitespace differs. See highlights below. Special character legend
Input
5 50 60 140 200 75 70
Your output
50 60
Expected output
50 60
3: Compare output a
Output is nearly correct; but whitespace differs. See highlights below. Special character legend
Input
7 25 32 27 28 27 25 31 30
Your output
25 27 28 27 25
Expected output
25 27 28 27 25
Expert Solution

arrow_forward
Step 1: algo
for (i = 0; i < n; i++)
array[i] = scnr.nextInt();
a = scnr.nextInt();
for (i = 0; i < n; i++) {
if (array[i] <= a)
System.out.print(array[i] + " ");
}
System.out.println("\n");
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java Program ASAP Please pay attention to the screenshot for test case 2 and 3 and please make sure you match the result. Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.ArrayList;import java.util.Arrays;import java.util.InputMismatchException;import java.util.Scanner;public class FileSorting { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); while (true) { System.out.println("Please enter the file name or type QUIT to exit:"); String fileName = scanner.nextLine(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { ArrayList<String> lines = readFile(fileName); if (lines.isEmpty()) { System.out.println("File " + fileName + " is empty."); } else {…arrow_forwardwrite a code in java that has a row of 3 and a column of 3 and prints out the even numbers but if there is an odd number it instead prints it the space blankarrow_forwardI need help with fixing this java program as described in the image below: import java.util.Scanner; public class LabProgram { /* TODO: Write recursive drawTriangle() method here. */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int baseLength; baseLength = scnr.nextInt(); drawTriangle(baseLength); } }arrow_forward
- import java.util.Scanner; public class TriangleArea { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Triangle triangle1 = new Triangle(); Triangle triangle2 = new Triangle(); // TODO: Read and set base and height for triangle1 (use setBase() and setHeight()) // TODO: Read and set base and height for triangle2 (use setBase() and setHeight()) System.out.println("Triangle with smaller area:"); // TODO: Determine smaller triangle (use getArea()) // and output smaller triangle's info (use printInfo()) }} public class Triangle { private double base; private double height; public void setBase(double userBase){ base = userBase; } public void setHeight(double userHeight) { height = userHeight; } public double getArea() { double area = 0.5 * base * height; return area; } public void printInfo() { System.out.printf("Base:…arrow_forwardImplement the Board class. Make sure to read through those comments so that you know what is required. import java.util.Arrays; import java.util.Random; public class Board { // You don't have to use these constants, but they do make your code easier to read public static final byte UR = 0; public static final byte R = 1; public static final byte DR = 2; public static final byte DL = 3; public static final byte L = 4; public static final byte UL = 5; // You need a random number generator in order to make random moves. Use rand below private static final Random rand = new Random(); private byte[][] board; /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. Note that making random moves * could result in the board being solved. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { // TODO } /** * Construct a puzzle board using a 2D array of bytes to indicate the contents *…arrow_forwardWrite the Java method averageOfDoubles that is passed an array of double values, and returns the average of the array values.You can assume the array length is a positive integer.arrow_forward
- This is in Java. The assignement is to create a class. I am confused on how to write de code for an array that is up to 5. Also I am confused regarding how to meet the requirements of the constructor. This is what I have. public class InventoryOnShelf { //fields private int[] itemOnShelfList []; private int size; public InventoryOnShelf() { }arrow_forwardThis array method must be written by me. One at a time, shift all of the components to the right one at a time. position. 1 4 9 16 25 would become 25 1 4 9 16 in this case. Why can't I figure out how to make it to shift to the right instead of the left?Java 6th E. E7.10.b is the source of the issue.arrow_forwardCan you help me fix my code in Java, please? I am stuck to keep going. Thank youarrow_forward
- use java to create a card game with the implements listed for the program. If you are able to do the first couple of steps that would be helpful and for step one use an array list with the following code. The code below should create 52 cards. String[] suits = {"Hearts", "Clubs", "Spades", "Diamonds"}; String[] numbers = {"A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K"}; for(String oneSuit : suits){ for(String num : numbers){ System.out.println(oneSuit + " " + num); } }arrow_forwardSorting an array of batting averages would be useful only if you sort another array in the same sequence in Java. Explain.arrow_forwardneed help finishing/fixing this java code so it prints out all the movie names that start with the first 2 characters the user inputs the code is as follows: Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
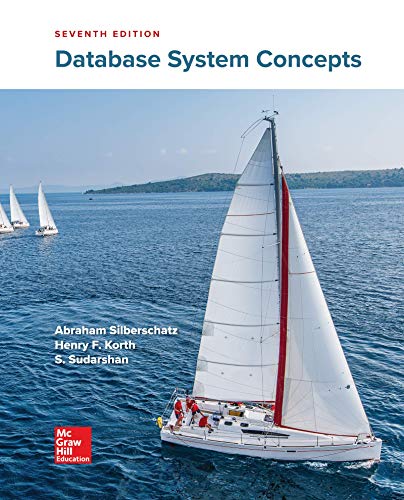
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
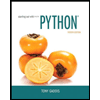
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
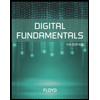
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
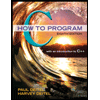
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
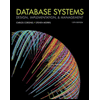
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
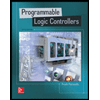
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education