In this assignment we will implement a made-up card game we'll call FaceUp. When the game starts, you deal five cards face down. Your goal is to achieve as high a score as possible. Your score only includes cards that are face up. Red cards (hearts and diamonds) award positive points, while black cards (clubs and spades) award negative points. Cards 2-10 have points worth their face value. Cards Jack, Queen, and King have value 10, and Ace is 11. The game is played by flipping over cards, either from face-down to face-up or from face-up to face-down. As you play, you are told your total score (ie, total of the face-up cards) and the total score of the cards that are face down. The challenge is that you only get up to a fixed number of flips and then the game is over. Here is an example of the output from playing the game: FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 0 Face down total: -5 Number of flips left: 5 Pick a card to flip between 1 and 5 (-1 to end game): 1 Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 10 Face down total: -15 Number of flips left: 4 Pick a card to flip between 1 and 5 (-1 to end game): 2 Queen of hearts | Jack of clubs | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 0 Face down total: -5 Number of flips left: 3 Pick a card to flip between 1 and 5 (-1 to end game): 2 Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 10 Face down total: -15 Number of flips left: 2 Pick a card to flip between 1 and 5 (-1 to end game): 10 10 is not a valid card Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 10 Face down total: -15 Number of flips left: 2 Pick a card to flip between 1 and 5 (-1 to end game): 0 0 is not a valid card Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 10 Face down total: -15 Number of flips left: 2 Pick a card to flip between 1 and 5 (-1 to end game): 5 Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | 3 of hearts Face up total: 13 Face down total: -18 Number of flips left: 1 Pick a card to flip between 1 and 5 (-1 to end game): -1 ---------------------- Your score: 13 Best possible score: 15 At each point where the program asks the user to pick a card, the game waits for user input. How to proceed You may fill in the details of the FaceUpCard and FaceUpHandclasses however you’d like, but here’s what we recommend. Add the two methods to the FaceUpCard class (described in comments in the code). Add a main method to this class and test them to make sure they work. Read through the other two new classes FaceUpHand and FaceUpGame. Make sure you understand what all of the methods in FaceUpHand are supposed to do and that you understand how they’re used in the FaceUpGame class. Implement methods in the FaceUpHand class incrementally. Write one method and then test it! To test it, again, add a main method and write a small test or two to make sure it behaves like you’d expect. If you try to implement all of the methods and then test it by simply running FaceUpGame, 1) it’s very unlikely you’ll get it perfect the first time and then 2) it’s going to be very hard to figure out where the problem is. Examples Here are several example collections of cards (each an instance of FaceUpHand), and their optimal scores: Ace of spades, 3 of hearts, 6 of hearts, King of hearts, 3 of diamonds Best possible score: 22 4 of clubs, 8 of hearts, Jack of diamonds, 8 of clubs, 6 of diamonds Best possible score: 24 3 of spades, 5 of diamonds, 3 of diamonds, 7 of spades, 5 of spades Best possible score: 8 ublic class FaceUpGame { privatestaticfinalintNUM_CARDS = 5; publicstaticvoidplayGame(intnumRounds) { FaceUpHandcards = newFaceUpHand(NUM_CARDS); Scannerin = newScanner(System.in); intflipsLeft = numRounds; while (flipsLeft > 0) { System.out.println(cards); System.out.println("Face up total: " + cards.faceUpTotal()); System.out.println("Face down total: " + cards.faceDownTotal()); System.out.println("Number of flips left: " + flipsLeft); System.out.print("Pick a card to flip between 1 and " + NUM_CARDS + " (-1 to end game): "); intnum = in.nextInt(); System.out.println(); if (num == -1) { flipsLeft = 0; } else if (num < 1 || num > NUM_CARDS) { System.out.println(num + " is not a valid card"); } else { cards.flipCard(num-1); flipsLeft--; } } in.close(); System.out.println(); System.out.println("----------------------"); System.out.println("Your score: " + cards.faceUpTotal()); System.out.println("Best possible score: " + cards.calculateOptimal()); } publicstaticvoidmain(String[] args) { playGame(5); }
FaceUp card game
In this assignment we will implement a made-up card game we'll call FaceUp. When the game starts, you deal five cards face down. Your goal is to achieve as high a score as possible. Your score only includes cards that are face up. Red cards (hearts and diamonds) award positive points, while black cards (clubs and spades) award negative points. Cards 2-10 have points worth their face value. Cards Jack, Queen, and King have value 10, and Ace is 11.
The game is played by flipping over cards, either from face-down to face-up or from face-up to face-down. As you play, you are told your total score (ie, total of the face-up cards) and the total score of the cards that are face down. The challenge is that you only get up to a fixed number of flips and then the game is over.
Here is an example of the output from playing the game:
FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 0 Face down total: -5 Number of flips left: 5 Pick a card to flip between 1 and 5 (-1 to end game): 1 Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 10 Face down total: -15 Number of flips left: 4 Pick a card to flip between 1 and 5 (-1 to end game): 2 Queen of hearts | Jack of clubs | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 0 Face down total: -5 Number of flips left: 3 Pick a card to flip between 1 and 5 (-1 to end game): 2 Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 10 Face down total: -15 Number of flips left: 2 Pick a card to flip between 1 and 5 (-1 to end game): 10 10 is not a valid card Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 10 Face down total: -15 Number of flips left: 2 Pick a card to flip between 1 and 5 (-1 to end game): 0 0 is not a valid card Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 10 Face down total: -15 Number of flips left: 2 Pick a card to flip between 1 and 5 (-1 to end game): 5 Queen of hearts | FACE-DOWN | FACE-DOWN | FACE-DOWN | 3 of hearts Face up total: 13 Face down total: -18 Number of flips left: 1 Pick a card to flip between 1 and 5 (-1 to end game): -1 ---------------------- Your score: 13 Best possible score: 15
At each point where the program asks the user to pick a card, the game waits for user input.
How to proceed
You may fill in the details of the FaceUpCard and FaceUpHandclasses however you’d like, but here’s what we recommend.
- Add the two methods to the FaceUpCard class (described in comments in the code). Add a main method to this class and test them to make sure they work.
- Read through the other two new classes FaceUpHand and FaceUpGame. Make sure you understand what all of the methods in FaceUpHand are supposed to do and that you understand how they’re used in the FaceUpGame class.
- Implement methods in the FaceUpHand class incrementally. Write one method and then test it! To test it, again, add a main method and write a small test or two to make sure it behaves like you’d expect. If you try to implement all of the methods and then test it by simply running FaceUpGame, 1) it’s very unlikely you’ll get it perfect the first time and then 2) it’s going to be very hard to figure out where the problem is.
Examples
Here are several example collections of cards (each an instance of FaceUpHand), and their optimal scores:
Ace of spades, 3 of hearts, 6 of hearts, King of hearts, 3 of diamonds Best possible score: 22
4 of clubs, 8 of hearts, Jack of diamonds, 8 of clubs, 6 of diamonds Best possible score: 24
3 of spades, 5 of diamonds, 3 of diamonds, 7 of spades, 5 of spades Best possible score: 8

![12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
public class FaceUpHand
private FaceUpCard [] cards;
/* Create a new FaceUp card game state, which consists of the
* array cards of size numCards
* @param numCards number of cards in the game/
public FaceUpHand (int numCards) {
// TODO: fill in method definition to initialize the cards array
// 1. set the instance variable cards equal to a new array (size numCards) of type FaceUpCard
// 2. create a variable dealer of type CardDealer for 1 deck
// 3. loop through the cards array and set each value to dealer.next()
}
/* Return the FaceUpCard in position cardIndex of cards
*
* @return the element of cards specified by cardIndex*/
public FaceUpCard getCard (int cardIndex) {
// TODO: fill in method definition; replace placeholder on next line
return null;
}
/* Flip the card over at this index
* Card indices start at 0 and go up the cards.length-1
* @param cardIndex the index of the card to flip over*/
public void flipCard(int cardIndex) {
// TODO: fill in method definition
}
/* Calculate the best possible score for the current cards
* @return the optimal score*/
public int calculateOptimal() {
// TODO: fill in method definition; replace placeholder on next line
return 0;
}
/* Calculate the total score for the cards that are face up
* @return the total score for face-up cards*/
public int faceUpTotal() {
// Hint: use getCardValue() and remember red cards have positive value
// and black cards have negative value
// TODO: fill in method definition; replace placeholder on next line
return 0;
/* Calculate the total score for the cards that are face down
* @return the total score for face-down cards*/
public int faceDownTotal() {
// Hint: use getCardValue() and remember red cards have positive value
// and black cards have negative value
// TODO: fill in method definition; replace placeholder on next line
return 0;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F385eb8fb-6648-4df6-8047-4f830c46ad3b%2Fcc9639bf-271c-43bf-9895-c747c562ca04%2Faq3gxai_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

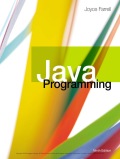
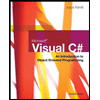
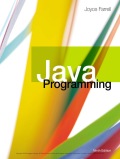
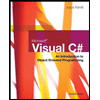