****************************Instructions***************************** Add a "copy constructor" to your dynamic-array student. Remember, the prototype looks like: Classname(const classname& otherObject); Update your test program to use the copy constructor. e.g., CourseTypeList list2(list1); ********************************************************************* Submit: Updated test program, implementation and header files (.cpp and .h) for CourseListType and CourseType zipped into a single file. //courseTypeList.h #ifndef COURSELISTTYPE_H_INCLUDED #define COURSELISTTYPE_H_INCLUDED #include #include "courseType.h" class courseTypeList { public: void print() const; void addCourse(std::string); courseTypeList(int n); ~courseTypeList(); private: int NUM_COURSES; int courseCount; courseType *courses; }; #endif // COURSELISTTYPE_H_INCLUDED =============================================== //courseTypeList.cpp #include #include #include "courseTypeList.h" void courseTypeList::print() const { for (int i = 0; i < courseCount; i++) { courses[i].printCourse(); } } void courseTypeList::addCourse(std::string name) { courses[courseCount].setCourseName(name); courses[courseCount].setCourseID(1000+courseCount); courseCount++; } courseTypeList::courseTypeList(int n = 50) { courseCount = 0; courses = new courseType[n]; NUM_COURSES = n; } courseTypeList::~courseTypeList() { delete &courses; } =============================================== //courseType.h #ifndef COURSETYPE_H_INCLUDED #define COURSETYPE_H_INCLUDED #include #include using namespace std; class courseType { public: courseType(int, string); courseType(); int getCourseID(); string getCourseName(); void setCourseID(int); void setCourseName(string); void printCourse(); private: int courseID; string courseName; }; #endif =============================================== //courseType.cpp #include "courseType.h" #include using namespace std; courseType::courseType(int id, string name) { this-> courseID = id; this-> courseName = name; } int courseType::getCourseID() { return courseID; } string courseType::getCourseName() { return courseName; } void courseType::setCourseID(int id) { courseID = id; } void courseType::setCourseName (string name) { courseName = name; } courseType::courseType() { this-> courseID = 0; this-> courseName = ""; } void courseType::printCourse() { cout << "Course ID: " << getCourseID() << endl; cout << "Course Name: " << getCourseName() << endl; } =============================================== //test.cpp #include"courseTypeList.cpp" #include using namespace std; int main() { courseTypeList c1(10); c1.addCourse("CSC"); c1.addCourse("Physics"); c1.print(); } =========================================
****************************Instructions*****************************
- Add a "copy constructor" to your dynamic-array student. Remember, the prototype looks like:
- Classname(const classname& otherObject);
- Classname(const classname& otherObject);
- Update your test
program to use the copy constructor.
- e.g., CourseTypeList list2(list1);
*********************************************************************
Submit: Updated test program, implementation and header files (.cpp and .h) for CourseListType and CourseType zipped into a single file.
//courseTypeList.h
#ifndef COURSELISTTYPE_H_INCLUDED
#define COURSELISTTYPE_H_INCLUDED
#include <string>
#include "courseType.h"
class courseTypeList {
public:
void print() const;
void addCourse(std::string);
courseTypeList(int n);
~courseTypeList();
private:
int NUM_COURSES;
int courseCount;
courseType *courses;
};
#endif // COURSELISTTYPE_H_INCLUDED
===============================================
//courseTypeList.cpp
#include <iostream>
#include <string>
#include "courseTypeList.h"
void courseTypeList::print() const {
for (int i = 0; i < courseCount; i++) {
courses[i].printCourse();
}
}
void courseTypeList::addCourse(std::string name) {
courses[courseCount].setCourseName(name);
courses[courseCount].setCourseID(1000+courseCount);
courseCount++;
}
courseTypeList::courseTypeList(int n = 50)
{
courseCount = 0;
courses = new courseType[n];
NUM_COURSES = n;
}
courseTypeList::~courseTypeList()
{
delete &courses;
}
===============================================
//courseType.h
#ifndef COURSETYPE_H_INCLUDED
#define COURSETYPE_H_INCLUDED
#include <iostream>
#include <string>
using namespace std;
class courseType {
public:
courseType(int, string);
courseType();
int getCourseID();
string getCourseName();
void setCourseID(int);
void setCourseName(string);
void printCourse();
private:
int courseID;
string courseName;
};
#endif
===============================================
//courseType.cpp
#include "courseType.h"
#include <string>
using namespace std;
courseType::courseType(int id, string name) {
this-> courseID = id;
this-> courseName = name;
}
int courseType::getCourseID() {
return courseID;
}
string courseType::getCourseName() {
return courseName;
}
void courseType::setCourseID(int id) {
courseID = id;
}
void courseType::setCourseName (string name) {
courseName = name;
}
courseType::courseType()
{
this-> courseID = 0;
this-> courseName = "";
}
void courseType::printCourse() {
cout << "Course ID: " << getCourseID() << endl;
cout << "Course Name: " << getCourseName() << endl;
}
===============================================
//test.cpp
#include"courseTypeList.cpp"
#include<iostream>
using namespace std;
int main()
{
courseTypeList c1(10);
c1.addCourse("CSC");
c1.addCourse("Physics");
c1.print();
}
=========================================

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

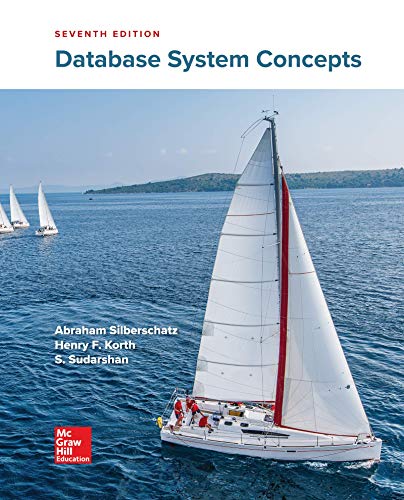
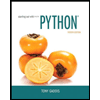
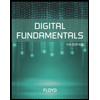
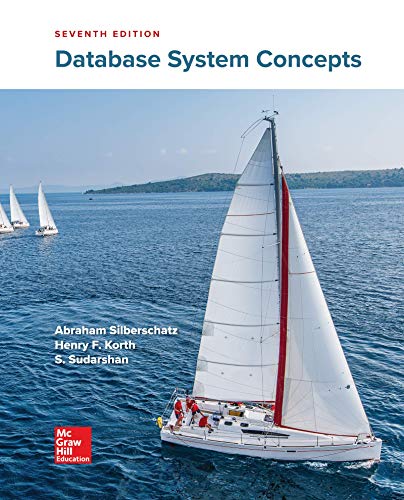
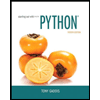
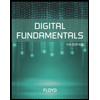
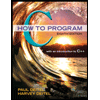
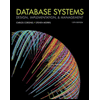
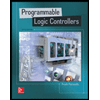