In this lab, you use a counter-controlled while loop in a C++ program provided for you. When completed, the program should print the numbers 0 through 10, along with their values multiplied by 2 and by 10. The data file contains the necessary variable declarations and some output statements. Instructions Ensure the source code file named Multiply.cpp is open in the code editor. Write a counter-controlled while loop that uses the loop control variable to take on the values 0 through 10. Remember to initialize the loop control variable before the program enters the loop. In the body of the loop, multiply the value of the loop control variable by 2 and by 10. Remember to change the value of the loop control variable in the body of the loop. Execute the program by clicking the Run button. Record the output of this program. Strictly use the given code // Multiply.cpp - This program prints the numbers 0 through 10 along // with these values multiplied by 2 and by 10. // Input: None // Output: Prints the numbers 0 through 10 along with their values multiplied by 2 and by 10. #include #include using namespace std; int main() { string head1 = "Number: "; string head2 = "Multiplied by 2: "; string head3 = "Multiplied by 10: "; int numberCounter; // Numbers 0 through 10 int byTen; // Stores the number multiplied by 10 int byTwo; // Stores the number multiplied by 2 const int NUM_LOOPS = 10; // Constant used to control loop // This is the work done in the housekeeping() function cout << "0 through 10 multiplied by 2 and by 10." << endl; // This is the work done in the detailLoop() function // Write while loop here // This is the work done in the endOfJob() function return 0; } // End of main()
In this lab, you use a counter-controlled while loop in a C++ program provided for you. When completed, the program should print the numbers 0 through 10, along with their values multiplied by 2 and by 10. The data file contains the necessary variable declarations and some output statements.
Instructions
-
Ensure the source code file named Multiply.cpp is open in the code editor.
-
Write a counter-controlled while loop that uses the loop control variable to take on the values 0 through 10. Remember to initialize the loop control variable before the program enters the loop.
-
In the body of the loop, multiply the value of the loop control variable by 2 and by 10. Remember to change the value of the loop control variable in the body of the loop.
-
Execute the program by clicking the Run button. Record the output of this program.
Strictly use the given code

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

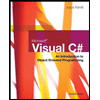
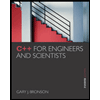
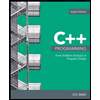
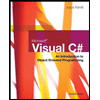
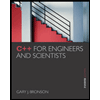
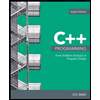