In this problem, you are going to create an Employee superclass and an HourlyEmployee subclass. The Employee class will hold the employee’s name and annual salary. You should have getters for both instance variables, but only a setter for the salary. In the HourlyEmployee class, you will add an hours instance variable as well as a method to set and get the hourly salary. You are not storing the hourly salary, but rather converting it to an annual salary. To convert an houlry salary to an annual salary, multiply the hourly rate by the number of hours per week and then by 52. You will need to do the reverse to get the hourly salary. Both classes should have a toString and examples are in the starter code. Be sure to test your classes in the EmployeeTester class! public class EmployeeTester { public static void main(String[] args) { // Start here! } } public class HourlyEmployee extends Employee { private double hoursPerWeek; // Call the Employee constructor and pass it a calculated annual salary public HourlyEmployee(String name, double hourlySalary, double hoursPerWeek){ } // Get the annual salary from the superclass and convert it back // to hourly. public double getHourlySalary(){ } // Use the input to set the annual salary in the superclass public void setHourlySalary(double hourlySalary){ } /** * Example output: * Mike makes $18.0 per hour */ public String toString(){ } } public class Employee { private String name; private double salary; public Employee(String name, double annualSalary){ } public String getName(){ } public double getAnnualSalary(){ } public void setAnnualSalary(double annualSalary){ } /** * Example output: * Mr. Karel makes $75000.0 per year */ public String toString(){ } }
In this problem, you are going to create an Employee superclass and an HourlyEmployee subclass. The Employee class will hold the employee’s name and annual salary. You should have getters for both instance variables, but only a setter for the salary. In the HourlyEmployee class, you will add an hours instance variable as well as a method to set and get the hourly salary. You are not storing the hourly salary, but rather converting it to an annual salary. To convert an houlry salary to an annual salary, multiply the hourly rate by the number of hours per week and then by 52. You will need to do the reverse to get the hourly salary. Both classes should have a toString and examples are in the starter code. Be sure to test your classes in the EmployeeTester class!
public class EmployeeTester
{
public static void main(String[] args)
{
// Start here!
}
}
public class HourlyEmployee extends Employee {
private double hoursPerWeek;
// Call the Employee constructor and pass it a calculated annual salary
public HourlyEmployee(String name, double hourlySalary, double hoursPerWeek){
}
// Get the annual salary from the superclass and convert it back
// to hourly.
public double getHourlySalary(){
}
// Use the input to set the annual salary in the superclass
public void setHourlySalary(double hourlySalary){
}
/**
* Example output:
* Mike makes $18.0 per hour
*/
public String toString(){
}
}
public class Employee {
private String name;
private double salary;
public Employee(String name, double annualSalary){
}
public String getName(){
}
public double getAnnualSalary(){
}
public void setAnnualSalary(double annualSalary){
}
/**
* Example output:
* Mr. Karel makes $75000.0 per year
*/
public String toString(){
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

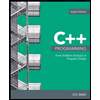
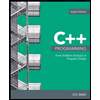