Please write the Java code for a Phone class that represents a mobile phone
Please write the Java code for a Phone class that represents a mobile phone, as detailed below:
- It should have instance variables (attributes) for make, model, color (all stored as Strings) and capacity (stored as int: the storage capacity in GB)
- Please define one constructor with parameters for all those attributes.
- The Phone class will be immutable, except its storage capacity can be changed. So please write only one mutator method, called .setCapacity.
- Please write accessor methods for each instance variable.
- Please write a .toString() method to display all the Phone's information.
- Please write a .equals method to compare two Phone objects, returning true if all their attributes are the same.
Finish the Jave program to show the outputs bellow:
Code:
class Main
{
public static void main(String[] args)
{
Phone craigPhone, bettoPhone, tobyPhone;
craigPhone = new Phone("Samsung", "Galaxy S21 5G", "Phantom Violet", 256);
bettoPhone = new Phone("Apple", "iPhone 13 Pro", "Graphite", 512);
tobyPhone = new Phone("Apple", "iPhone 13 Pro", "Graphite", 512);
System.out.println("The brightest color here is: " + craigPhone.getColor());
if(craigPhone.getCapacity() < bettoPhone.getCapacity())
{
System.out.println("This phone:");
System.out.println(craigPhone);
System.out.println("has lower capacity than the:");
System.out.println(bettoPhone);
}
else
{
System.out.println("Error! This phone:");
System.out.println(craigPhone);
System.out.println("should have lower capacity than the:");
System.out.println(bettoPhone);
}
if(bettoPhone.equals(tobyPhone))
{
System.out.println("We have two of these:");
System.out.println(bettoPhone);
}
else
{
System.out.println("Error! This phone:");
System.out.println(tobyPhone);
System.out.println("should be equal to:");
System.out.println(bettoPhone);
}
tobyPhone.setCapacity(1000); // upgrade to 1TB
if(bettoPhone.equals(tobyPhone))
{
System.out.println("Error! After upgrade, this phone:");
System.out.println(tobyPhone);
System.out.println("should have more capacity than:");
System.out.println(bettoPhone);
}
else
{
System.out.println("After upgrade they aren't equal, because this phone:");
System.out.println(tobyPhone);
System.out.println("has more capacity than:");
System.out.println(bettoPhone);
}
}
}
Output:

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

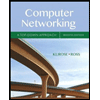
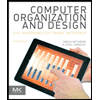
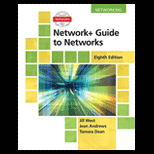
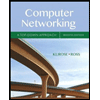
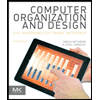
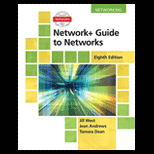
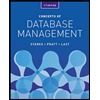
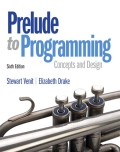
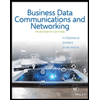