In your program, you will demonstrate an understanding of all these concepts by developing your own priority queue, node, binary tree, array and/or linked list, and use of recursion to support the Huffman Code encryption algorithm. Read through the theory of the Huffman Code in the text and then write a program that can take a String and encrypt it. For example, given a String “Hello World”, the program should apply the Huffman Code algorithm on that String to create the corresponding encrypted code sequence.
Could you help me the following question in java
In this assignment, you will do an implementation of the Huffman Code.
The Huffman Code requires knowledge of a few data structure of the following:-
- Priority Queues
- Binary Trees
- Recursion
- Arrays and/or Linked Lists
In your program, you will demonstrate an understanding of all these concepts by developing your own priority queue, node, binary tree, array and/or linked list, and use of recursion to support the Huffman Code encryption
In addition, you must be able to show the various components that support creating the encrypted String. For the Huffman Code, this requires the following:
- Creating a frequency table for each character of the String (I suggest this to be an array but can be done as a linked list)
- Creating a Huffman Tree from the frequency table (This will require use of a priority queue and a binary tree)
- Creating the Huffman Code table (This should be an array and will require use of recursion to build)
The program does not need to be interactive (but may be interesting for you to do so). You can hard code the String to encode such as the one used in the text and show the result as output. I may change the String in the code to test your work. For your program output though, you must be able to show the contents of each of the supporting components listed above.
For example, in main, you may hard code a given String like “Hello World”. You then need create structures and classes for each of the 3 components listed for the Huffman Code to work. This means you’ll need to display:
- A frequency table for all the characters of the “Hello World” string
- A Huffman tree from that frequency table (you do not need to show the priority queue used to build the tree, unless you think it helps)
- A Huffman code table that shows the codes generated for each character
After all that is shown, you must finally show the code sequence for the String using the derived Huffman codes.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

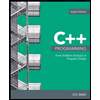
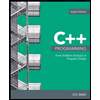