Use java or python to solve Note: 1) Assume that the Node class and Linked Lists constructors are already written. You ONLY need to write the revinsert0 method/function 2) No need to write the Tester Class. 3) Use of Built in Method is not allowed except count(). You must take an inplace approach and modify the given list. 5) You cannot use arrays to solve this problem
Use java or python to solve Note: 1) Assume that the Node class and Linked Lists constructors are already written. You ONLY need to write the revinsert0 method/function 2) No need to write the Tester Class. 3) Use of Built in Method is not allowed except count(). You must take an inplace approach and modify the given list. 5) You cannot use arrays to solve this problem
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Use java or python to solve
Note:
1) Assume that the Node class and Linked Lists constructors are already written. You ONLY
need to write the revinsert0 method/function
2) No need to write the Tester Class.
3) Use of Built in Method is not allowed except count(). You must take an inplace approach and
modify the given list.
5) You cannot use arrays to solve this problem
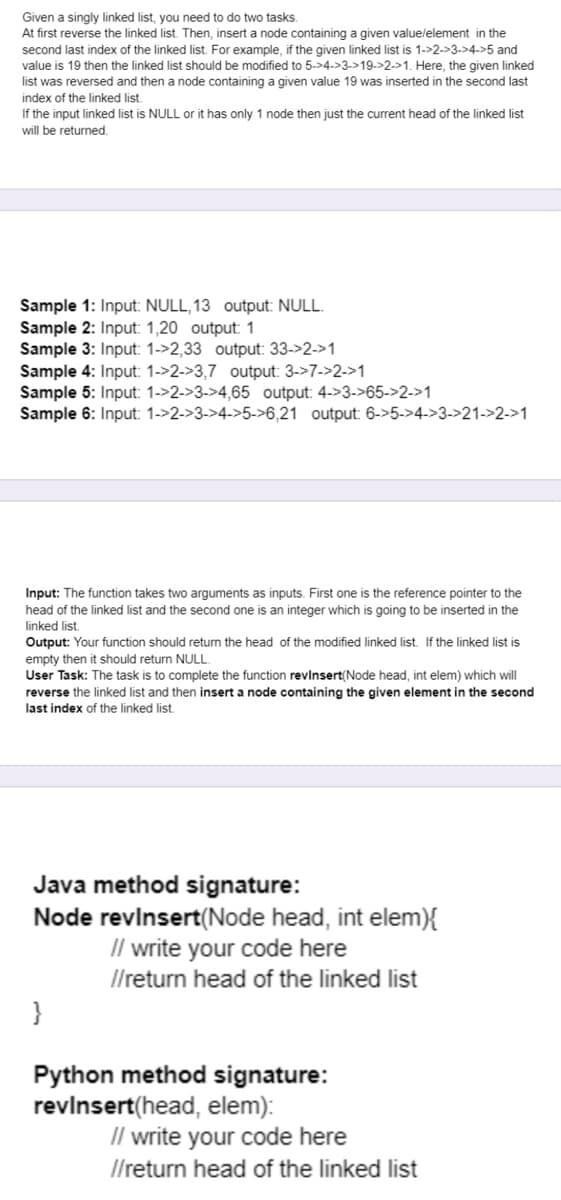
Transcribed Image Text:Given a singly linked list, you need to do two tasks.
At first reverse the linked list. Then, insert a node containing a given value/element in the
second last index of the linked list. For example, if the given linked list is 1->2->3->4->5 and
value is 19 then the linked list should be modified to 5->4->3->19->2->1. Here, the given linked
list was reversed and then a node containing a given value 19 was inserted in the second last
index of the linked list.
If the input linked list is NULL or it has only 1 node then just the current head of the linked list
will be returned.
Sample 1: Input: NULL, 13 output: NULL.
Sample 2: Input: 1,20 output: 1
Sample 3: Input: 1->2,33 output: 33->2->1
Sample 4: Input: 1->2->3,7 output: 3->7->2->1
Sample 5: Input: 1->2->3->4,65 output: 4->3->65->2->1
Sample 6: Input: 1->2->3->4->5->6,21 output: 6->5->4->3->21->2->1
Input: The function takes two arguments as inputs. First one is the reference pointer to the
head of the linked list and the second one is an integer which is going to be inserted in the
linked list.
Output: Your function should return the head of the modified linked list. If the linked list is
empty then it should return NULL.
User Task: The task is to complete the function revlnsert(Node head, int elem) which will
reverse the linked list and then insert a node containing the given element in the second
last index of the linked list.
Java method signature:
Node revlnsert(Node head, int elem){
Il write your code here
I/return head of the linked list
}
Python method signature:
revlnsert(head, elem):
// write your code here
I/return head of the linked list
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
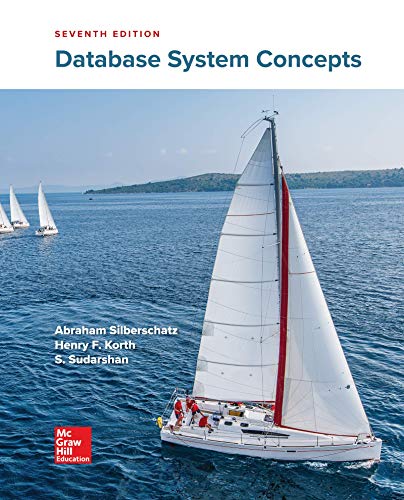
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
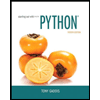
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
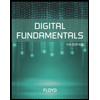
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
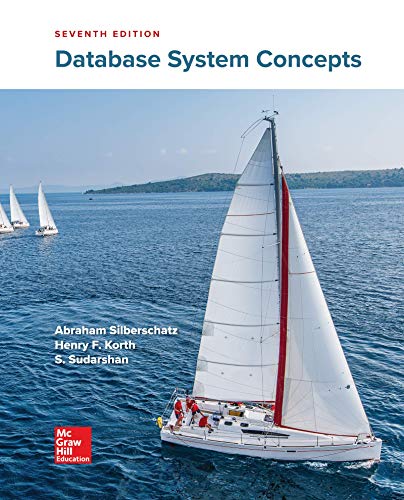
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
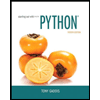
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
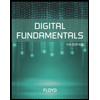
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
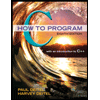
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
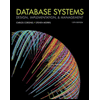
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
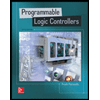
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education