#include #define FAIL_VAL -99999 #define SUCCESS_VAL 99999 #define MAX_USER 20 #define MAX_LEN 200 char emailArr[MAX_USER][MAX_LEN]; char passArr[MAX_USER][MAX_LEN]; char nameArr[MAX_USER][MAX_LEN]; char addressArr[MAX_USER][MAX_LEN]; int user_count=0; int signupUser(char email[], char pass[]){ char Email_id[60]; int i;//p1=0,p2=0; printf("Enter your Email_id: "); scanf("%s", &Email_id); if(Email_id[i]=='@' && Email_id[i]=='.') { printf("Your Email_id is valid"); } else { printf("Email_id invalid.Please enter a valid id."); } } int loginUser(char email[], char pass[]){ //Write your code here } int buildUserProfile(char email[], char firstName[], char lastName[], char address[]){ //Write your code here } void searchByName(char name[]){ //Write your code here } void searchByAddress(char address[]){ //Write your code here } int main() { while(1) { printf("*******************************************************************\n"); printf("1. signup User. 2. login User. 3. build User Profile. 4.Search By Name. 5. Search By Address. 6.exit \n"); int choice; scanf("%d",&choice); if(choice==1){ char mail[MAX_LEN], pass[MAX_LEN]; printf("give User Email :"); scanf("\n%[^\n]s",mail); printf("give User Password :"); scanf("\n%[^\n]s",pass); signupUser(mail,pass); } if(choice==2){ char mail[MAX_LEN], pass[MAX_LEN]; printf("give User Email :"); scanf("\n%[^\n]s",mail); printf("give User Password :"); scanf("\n%[^\n]s",pass); loginUser(mail,pass); } if(choice==3){ char mail[MAX_LEN], fname[MAX_LEN], lname[MAX_LEN], address[MAX_LEN]; printf("give User Email :"); scanf("\n%[^\n]s",mail); printf("%s\n",mail); printf("give First Name :"); scanf("\n%[^\n]s",fname); printf("%s\n",fname); printf("give Last Name :"); scanf("\n%[^\n]s",lname); printf("%s\n",lname); printf("give Address :"); scanf("\n%[^\n]s",address); printf("%s\n",address); buildUserProfile(mail,fname,lname,address); } if(choice==4){ char name[MAX_LEN]; printf("give User Name :"); scanf("\n%[^\n]s",name); searchByName(name); } if(choice==5){ char address[MAX_LEN]; printf("give User Address :"); scanf("\n%[^\n]s",address); searchByAddress(address); } if (choice==6){ break; } } } Implement the function loginUser() that takes two String as input, one for user email and another for password. It will search for the given user email in emailArr. If the user email exists and the given password matches with the stored one against that email, then login will be successful and you should return the array index plus show a success message. Otherwise, login will be unsuccessful and you should return FAIL_VAL plus show a proper error message.
#include<stdio.h>
#define FAIL_VAL -99999
#define SUCCESS_VAL 99999
#define MAX_USER 20
#define MAX_LEN 200
char emailArr[MAX_USER][MAX_LEN];
char passArr[MAX_USER][MAX_LEN];
char nameArr[MAX_USER][MAX_LEN];
char addressArr[MAX_USER][MAX_LEN];
int user_count=0;
int signupUser(char email[], char pass[]){
char Email_id[60];
int i;//p1=0,p2=0;
printf("Enter your Email_id: ");
scanf("%s", &Email_id);
if(Email_id[i]=='@' && Email_id[i]=='.')
{
printf("Your Email_id is valid");
}
else
{
printf("Email_id invalid.Please enter a valid id.");
}
}
int loginUser(char email[], char pass[]){
//Write your code here
}
int buildUserProfile(char email[], char firstName[], char lastName[], char address[]){
//Write your code here
}
void searchByName(char name[]){
//Write your code here
}
void searchByAddress(char address[]){
//Write your code here
}
int main()
{
while(1)
{
printf("*******************************************************************\n");
printf("1. signup User. 2. login User. 3. build User Profile. 4.Search By Name. 5. Search By Address. 6.exit \n");
int choice;
scanf("%d",&choice);
if(choice==1){
char mail[MAX_LEN], pass[MAX_LEN];
printf("give User Email :");
scanf("\n%[^\n]s",mail);
printf("give User Password :");
scanf("\n%[^\n]s",pass);
signupUser(mail,pass);
}
if(choice==2){
char mail[MAX_LEN], pass[MAX_LEN];
printf("give User Email :");
scanf("\n%[^\n]s",mail);
printf("give User Password :");
scanf("\n%[^\n]s",pass);
loginUser(mail,pass);
}
if(choice==3){
char mail[MAX_LEN], fname[MAX_LEN], lname[MAX_LEN], address[MAX_LEN];
printf("give User Email :");
scanf("\n%[^\n]s",mail);
printf("%s\n",mail);
printf("give First Name :");
scanf("\n%[^\n]s",fname);
printf("%s\n",fname);
printf("give Last Name :");
scanf("\n%[^\n]s",lname);
printf("%s\n",lname);
printf("give Address :");
scanf("\n%[^\n]s",address);
printf("%s\n",address);
buildUserProfile(mail,fname,lname,address);
}
if(choice==4){
char name[MAX_LEN];
printf("give User Name :");
scanf("\n%[^\n]s",name);
searchByName(name);
}
if(choice==5){
char address[MAX_LEN];
printf("give User Address :");
scanf("\n%[^\n]s",address);
searchByAddress(address);
}
if (choice==6){
break;
}
}
}
Implement the function loginUser() that takes two String as input, one for user email and another for password. It will search for the given user email in emailArr. If the user email exists and the given password matches with the stored one against that email, then login will be successful and you should return the array index plus show a success message. Otherwise, login will be unsuccessful and you should return FAIL_VAL plus show a proper error message.

Step by step
Solved in 4 steps with 2 images

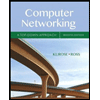
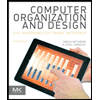
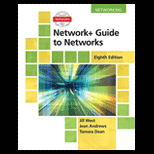
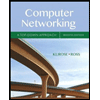
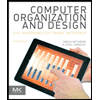
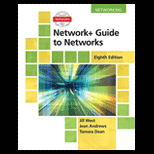
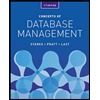
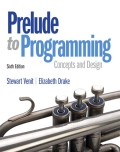
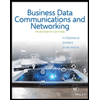