#include #include #include #include using namespace std::chrono; using namespace std; void randomVector(int vector[], int size) { for (int i = 0; i < size; i++) { //ToDo: Add Comment vector[i] = rand() % 100; } } int main(){ unsigned long size = 100000000; srand(time(0)); int *v1, *v2, *v3; //ToDo: Add Comment auto start = high_resolution_clock::now(); //ToDo: Add Comment v1 = (int *) malloc(size * sizeof(int *)); v2 = (int *) malloc(size * sizeof(int *)); v3 = (int *) malloc(size * sizeof(int *)); randomVector(v1, size); randomVector(v2, size); //ToDo: Add Comment for (int i = 0; i < size; i++) { v3[i] = v1[i] + v2[i]; } auto stop = high_resolution_clock::now(); //ToDo: Add Comment auto duration = duration_cast(stop - start); cout << "Time taken by function: " << duration.count() << " microseconds" << endl; return 0; } Complete the code by adding appropriate comments in the designated lines. Develop a roadmap to parallelise this program. You should start with decomposition of the program/problem into sub-tasks - i.e. partitioning data/tasks. Document your list of sub-tasks or activities you plan to do in parallel vs activities that need to be in sequence. Implement your parallel algorithm in C or C++ using pthread or std::thread library. Evaluate the performance of your program (using execution time as a metric), to assess the speed up achieved. Compare the results with the sequential program. Varry the partition size and analyse how it can impact the executing time of the program.
#include <iostream>
#include <cstdlib>
#include <time.h>
#include <chrono>
using namespace std::chrono;
using namespace std;
void randomVector(int
{
for (int i = 0; i < size; i++)
{
//ToDo: Add Comment
vector[i] = rand() % 100;
}
}
int main(){
unsigned long size = 100000000;
srand(time(0));
int *v1, *v2, *v3;
//ToDo: Add Comment
auto start = high_resolution_clock::now();
//ToDo: Add Comment
v1 = (int *) malloc(size * sizeof(int *));
v2 = (int *) malloc(size * sizeof(int *));
v3 = (int *) malloc(size * sizeof(int *));
randomVector(v1, size);
randomVector(v2, size);
//ToDo: Add Comment
for (int i = 0; i < size; i++)
{
v3[i] = v1[i] + v2[i];
}
auto stop = high_resolution_clock::now();
//ToDo: Add Comment
auto duration = duration_cast<microseconds>(stop - start);
cout << "Time taken by function: "
<< duration.count() << " microseconds" << endl;
return 0;
}
Complete the code by adding appropriate comments in the designated lines. Develop a roadmap to parallelise this program. You should start with decomposition of the
program/problem into sub-tasks - i.e. partitioning data/tasks. Document your list of sub-tasks or
activities you plan to do in parallel vs activities that need to be in sequence. Implement your parallel

Step by step
Solved in 3 steps

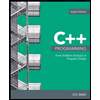
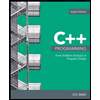