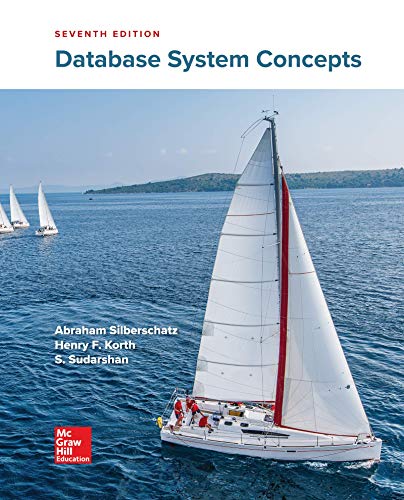
#include <iostream> #include <queue> //This contains the STL's efficient implementation of a priority queue using a heap using namespace std; /* In this lab you will implement a data structure that supports 2 primary operations: - insert a new item - remove and return the smallest item A data structure that supports these two operations is called a "priority queue". There are many ways to implement a priority queue, with differernt efficiencies for the two primary operations. For this lab, please do not attempt (at least at first) to implement something fancy (like a heap). Just use your mind to do something simple. Analyze the efficiency of both insertion and removal for your implementation. Afterwards, feel free to investigagte "min heaps" to see a very clever and fast priority queue implementation. Feel free to try to implement it. After implementuing your priority queue, use it to implement a sorting

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- 5. fast please Convert the following infix expression into its prefix and postfix equivalents: a / b - (c + d * e) / f * g For any postfix, show the final transformed expression using the stack method for transformation as explained in class showing all the relevant steps. For the prefix, show any needed reversion and the final transformed expression using the stack method for transformation as explained in class showing all the relevant steps. Draw and sequence your diagrams clearlyarrow_forwardgiven code lab4 #include <stdio.h>#include <stdlib.h> /* typical C boolean set-up */#define TRUE 1#define FALSE 0 typedef struct StackStruct{int* darr; /* pointer to dynamic array */int size; /* amount of space allocated */int inUse; /* top of stack indicator - counts how many values are on the stack */} Stack; void init (Stack* s){s->size = 2;s->darr = (int*) malloc ( sizeof (int) * s->size );s->inUse = 0;} void push (Stack* s, int val){/* QUESTION 7 *//* check if enough space currently on stack and grow if needed */ /* add val onto stack */s->darr[s->inUse] = val;s->inUse = s->inUse + 1;} int isEmpty (Stack* s){if ( s->inUse == 0)return TRUE;elsereturn FALSE;} int top (Stack* s){return ( s->darr[s->inUse-1] );} /* QUESTION 9.1 */void pop (Stack* s){if (isEmpty(s) == FALSE)s->inUse = s->inUse - 1;} void reset (Stack* s){/* Question 10: how to make the stack empty? */ } int main (int argc, char** argv){Stack st1; init (&st1);…arrow_forward4) Priority Queue Application Homework • Unanswered Situation: The order in which patients at a certain emergency room are seen by the doctor is based on arrival time minus 10 minutes for each major wound, minus 5 minutes for each minor wound, and plus 3 minutes for each time the patient annoys the nurse. You can assume a maximum wait time of 2 days. If a priority queue is used in the software at the nurses' station, would you use a minimum heap or a maximum heap to implement the priority queue? (Thought-provoker: Do you think if you annoyed the nurse enough, s/he would smack you around enough for you to move to the head of the line?)arrow_forward
- C++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the main code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS int main(int argc, char** argv) { SLLStack* stack = new SLLStack(); int test; int length; string str; char top; bool flag = true; cin >> test; switch (test) { case 0: getline(cin, str); length = str.length(); for(int i = 0; i < length; i++){ if(str[i] == '{' || str[i] == '(' || str[i] == '['){ stack->push(str[i]); } else if (str[i] == '}' || str[i] == ')' || str[i] == ']'){ if(!stack->isEmpty()){ top = stack->top(); if(top == '{' && str[i] == '}' || top == '(' && str[i] == ')' ||…arrow_forwardStack: Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1) bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1) void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1) Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1) Write non-parameterized constructor for the above class. Write Copy constructor for the above class. Write Destructor for the above class. Now write a global function show stack which…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
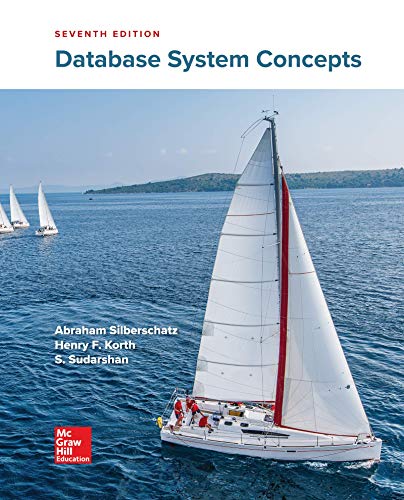
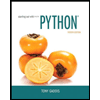
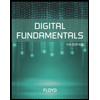
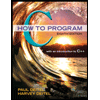
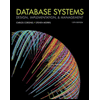
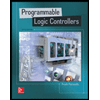