Part 1: Static Stack In this part, you are going to design a stack of characters. Assume a simple static array implementation. Complete the code below as specified by the comments below. const int MAX = 5; // define an alias for the element type class Stack { private: // declare an array of chars with MAX slots // and an integer to hold the index of the top element public: // Write the prototype of the following public methods // constructor, push, pop, isEmpty, isFull, displayAll } ; // implement the public methods here int main () Stack s; char c; cout << " initial stack contents" << endl; s.displayAl1(); s.pop (c); s.push ('a'); cout << endl << " stack contents after pushing a: s.displayAll (); << endl; s.push( 'b'); cout << endl << " stack contents after pushing b: " << endl; s.displayAl1(); s.push ('c'); s.push ( 'd'); s.push ( 'e'); s.push ( 'f'); s.push ( 'g'); cout << endl << " stack contents after pushing c-g: " << endl; s.displayAl1 (); s.pop (c); cout << endl << c << endl; cout << endl << " stack contents after popping one element: << endl; s.displayAl1 (); s.pop(c); cout << endl << " popped element: << c << endl; cout << endl << " stack contents after popping another element: " << endl; s.displayAl1 (); s.pop (c); S.pop (c); s.pop(c); cout << endl << " stack contents after popping 3 more elements: << endl; s.displayAl1(); s.pop (c); s.push ( 'a'); s.push ( 'b'); cout << endl << final stack contents << endl; s.displayAl1 (); return 0; }
Part 1: Static Stack In this part, you are going to design a stack of characters. Assume a simple static array implementation. Complete the code below as specified by the comments below. const int MAX = 5; // define an alias for the element type class Stack { private: // declare an array of chars with MAX slots // and an integer to hold the index of the top element public: // Write the prototype of the following public methods // constructor, push, pop, isEmpty, isFull, displayAll } ; // implement the public methods here int main () Stack s; char c; cout << " initial stack contents" << endl; s.displayAl1(); s.pop (c); s.push ('a'); cout << endl << " stack contents after pushing a: s.displayAll (); << endl; s.push( 'b'); cout << endl << " stack contents after pushing b: " << endl; s.displayAl1(); s.push ('c'); s.push ( 'd'); s.push ( 'e'); s.push ( 'f'); s.push ( 'g'); cout << endl << " stack contents after pushing c-g: " << endl; s.displayAl1 (); s.pop (c); cout << endl << c << endl; cout << endl << " stack contents after popping one element: << endl; s.displayAl1 (); s.pop(c); cout << endl << " popped element: << c << endl; cout << endl << " stack contents after popping another element: " << endl; s.displayAl1 (); s.pop (c); S.pop (c); s.pop(c); cout << endl << " stack contents after popping 3 more elements: << endl; s.displayAl1(); s.pop (c); s.push ( 'a'); s.push ( 'b'); cout << endl << final stack contents << endl; s.displayAl1 (); return 0; }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 1TF
Related questions
Question
in C++ langauge please and with the given functions.
I submitted earlier and someone did it with a peek(); function which i didnt need and was confusing me
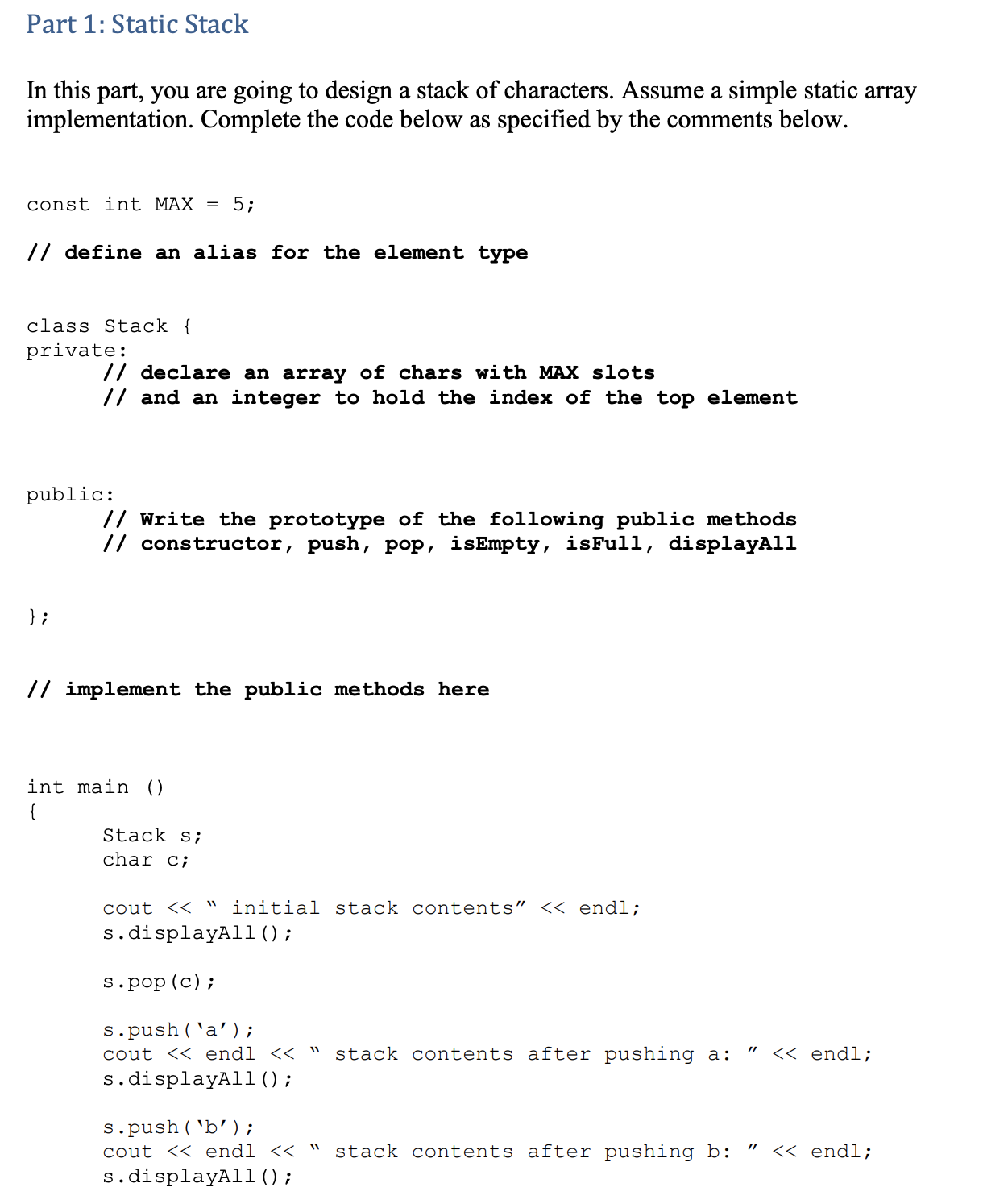
Transcribed Image Text:Part 1: Static Stack
In this part, you are going to design a stack of characters. Assume a simple static array
implementation. Complete the code below as specified by the comments below.
const int MAX = 5;
// define an alias for the element type
class Stack {
private:
// declare an array of chars with MAX slots
// and an integer to hold the index of the top element
public:
// Write the prototype of the following public methods
// constructor, push, pop, isEmpty, isFull, displayAll
} ;
// implement the public methods here
int main ()
Stack s;
char c;
cout << " initial stack contents" << endl;
s.displayAl1();
s.pop (c);
s.push ('a');
cout << endl << " stack contents after pushing a:
s.displayAll ();
<< endl;
s.push( 'b');
cout << endl << "
stack contents after pushing b:
" << endl;
s.displayAl1();
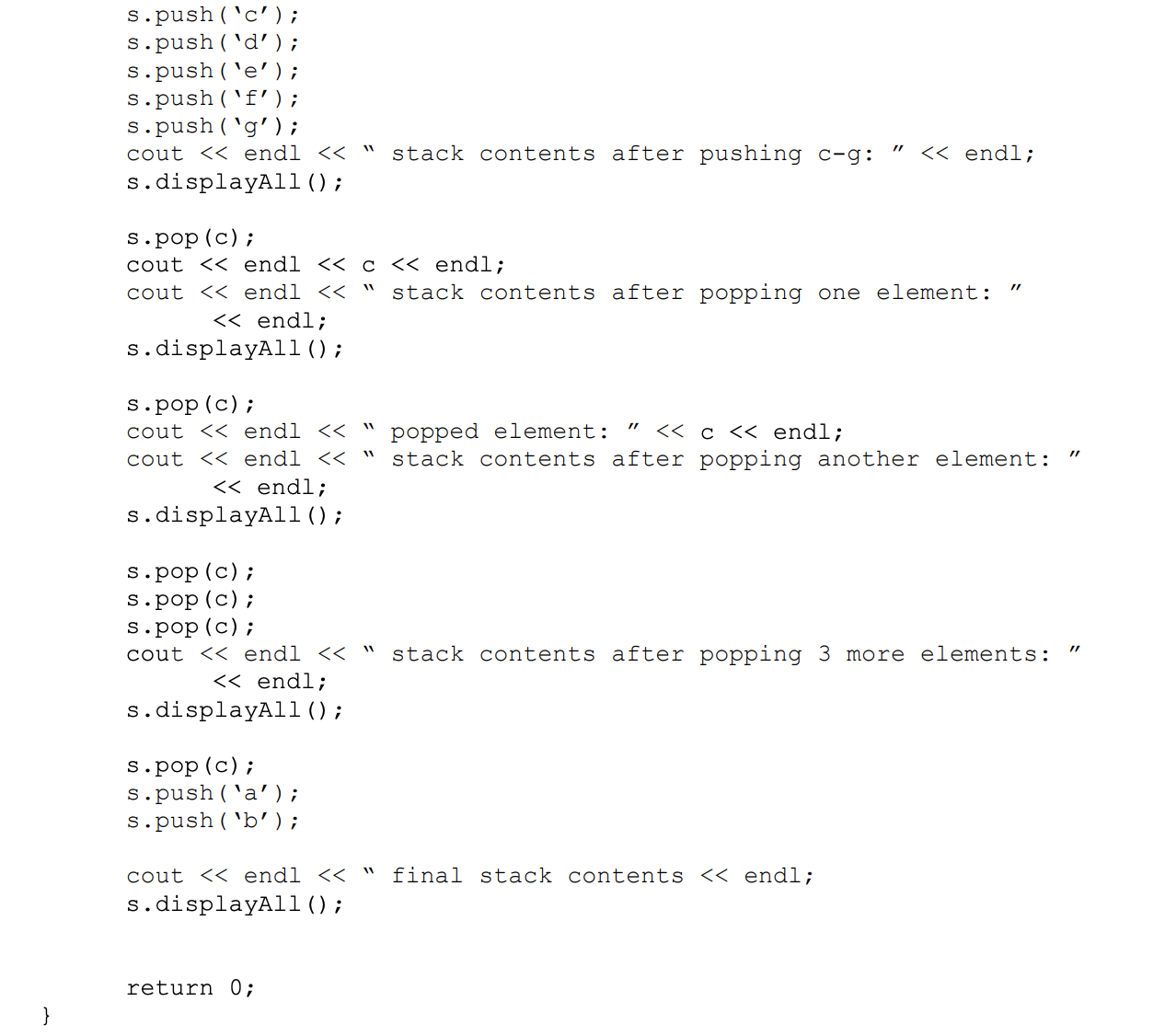
Transcribed Image Text:s.push ('c');
s.push ( 'd');
s.push ( 'e');
s.push ( 'f');
s.push ( 'g');
cout << endl << "
stack contents after pushing c-g:
" << endl;
s.displayAl1 ();
s.pop (c);
cout << endl << c << endl;
cout << endl << " stack contents after popping one element:
<< endl;
s.displayAl1 ();
s.pop(c);
cout << endl << "
popped element:
<< c << endl;
cout << endl << " stack contents after popping another element: "
<< endl;
s.displayAl1 ();
s.pop (c);
S.pop (c);
s.pop(c);
cout << endl << "
stack contents after popping 3 more elements:
<< endl;
s.displayAl1();
s.pop (c);
s.push ( 'a');
s.push ( 'b');
cout << endl <<
final stack contents << endl;
s.displayAl1 ();
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
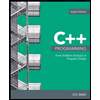
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
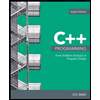
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning