Incoroporate or use below code as reference #include #include #include typedef struct node_struct { int item; struct node_struct *next; } node; /** * 10->NULL * We want to insert 20 * First call ([10], 20) [has not finished yet]
Incoroporate or use below code as reference #include #include #include typedef struct node_struct { int item; struct node_struct *next; } node; /** * 10->NULL * We want to insert 20 * First call ([10], 20) [has not finished yet]
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
1st Edition
ISBN:9780357392676
Author:FREUND, Steven
Publisher:FREUND, Steven
Chapter10: Data Analysis With Power Tools And Creating Macros
Section: Chapter Questions
Problem 2AYK
Related questions
Question
Incoroporate or use below code as reference
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
typedef struct node_struct {
int item;
struct node_struct *next;
} node;
/**
* 10->NULL
* We want to insert 20
* First call ([10], 20) [has not finished yet]
* {10, {20, NULL}}
*
*
* Second call (NULL, 20) {20, NULL}
*/
node* insert_unordered(node *temp_root, int item) {
if (temp_root == NULL) {
temp_root = malloc(sizeof(node));
temp_root->item = item;
temp_root->next = NULL;
} else {
temp_root->next = insert_unordered(temp_root->next, item);
}
return temp_root;
}
/**
* This is atypical linked list because
* (i) it does not allow duplicates and
* (ii) it is sorted
* 10->20, i want to insert 15
* 10->15->20
*
*/
![Submission details: Make a directory and name it, using your first and last names,
applying the camel notation, for example haniGirgis. Please name the main file,
where execution starts, as hw2.cpp. Compress your directory by the zip utility, and
submit the compressed file using Blackboard.
Introduction
Suppose you had a document (perhaps a newspaper article, or an unattributed
manuscript for a book), and you were interested in knowing who wrote it. One way
to try to determine the authorship of the anonymous document is by comparing
properties of the anonymous document with properties of known documents, and
seeing if there is enough similarity to make a judgment of authorship.
Some simple properties one might use to distinguish different authors include:
• Vocabulary (ie. the set of words an author uses).
• Word frequencies (i.e. the frequencies with which an author uses words).
• Bigram frequencies (i.e. the frequencies of two consecutive words).
• Bigram probabilities (i.e. the probability that one word follows another
word).
Terminology
• A unigram is a sequence of words of length one (i.e. a single word).
A bigram is a sequence of words of length two.
The conditional probability of an event E2 given another event E1, written
p(E2|E1), is the probability that E2 will occur given that event El has already
occurred.
We write p(w(k)]w(k-1)) for the conditional probability of a word w in position k,
w(k), given the immediately preceding word, w(k-1). You determine the conditional
probabilities by determining unigram counts (the number of times each word
appears, written c(w(k)), bigram counts (the number of times each pair of words
appears, written c(w(k-1) w(k)), and then dividing each bigram count by the
unigram count of the first word in the bigram:
p(WORD(k)|WORD(k-1)) = c(WORD(k-1) WORD(k}) / c(WORD(k-1))
For example, if the word "time" occurs seven times in a text, and "time of" occurs
three times, then the probability of "of" occurring after "time" is 3/7.
Project description
In this project, you will be determining conditional probabilities of bigrams. To do
this, you will write a C program, which reads in a file of text and produces three
output files, as described below.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F157e66f5-e794-4af2-988e-1885b818a80a%2F713c2df6-f70d-4d93-a043-8693381a58dd%2Fwpm1fr_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Submission details: Make a directory and name it, using your first and last names,
applying the camel notation, for example haniGirgis. Please name the main file,
where execution starts, as hw2.cpp. Compress your directory by the zip utility, and
submit the compressed file using Blackboard.
Introduction
Suppose you had a document (perhaps a newspaper article, or an unattributed
manuscript for a book), and you were interested in knowing who wrote it. One way
to try to determine the authorship of the anonymous document is by comparing
properties of the anonymous document with properties of known documents, and
seeing if there is enough similarity to make a judgment of authorship.
Some simple properties one might use to distinguish different authors include:
• Vocabulary (ie. the set of words an author uses).
• Word frequencies (i.e. the frequencies with which an author uses words).
• Bigram frequencies (i.e. the frequencies of two consecutive words).
• Bigram probabilities (i.e. the probability that one word follows another
word).
Terminology
• A unigram is a sequence of words of length one (i.e. a single word).
A bigram is a sequence of words of length two.
The conditional probability of an event E2 given another event E1, written
p(E2|E1), is the probability that E2 will occur given that event El has already
occurred.
We write p(w(k)]w(k-1)) for the conditional probability of a word w in position k,
w(k), given the immediately preceding word, w(k-1). You determine the conditional
probabilities by determining unigram counts (the number of times each word
appears, written c(w(k)), bigram counts (the number of times each pair of words
appears, written c(w(k-1) w(k)), and then dividing each bigram count by the
unigram count of the first word in the bigram:
p(WORD(k)|WORD(k-1)) = c(WORD(k-1) WORD(k}) / c(WORD(k-1))
For example, if the word "time" occurs seven times in a text, and "time of" occurs
three times, then the probability of "of" occurring after "time" is 3/7.
Project description
In this project, you will be determining conditional probabilities of bigrams. To do
this, you will write a C program, which reads in a file of text and produces three
output files, as described below.
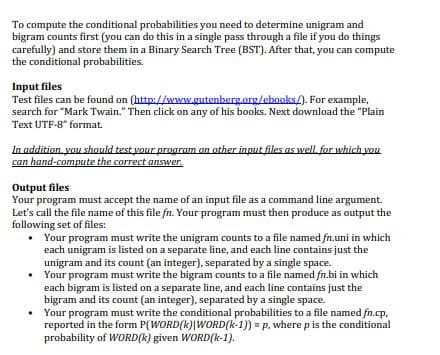
Transcribed Image Text:To compute the conditional probabilities you need to determine unigram and
bigram counts first (you can do this in a single pass through a file if you do things
carefully) and store them in a Binary Search Tree (BST). After that, you can compute
the conditional probabilities.
Input files
Test files can be found on (http://www.gutenberg.org/ebooks/). For example,
search for "Mark Twain." Then click on any of his books. Next download the "Plain
Text UTF-8" format.
In addition, you should test your program on other input files as well for which vou
can hand-compute the correct answer.
Output files
Your program must accept the name of an input file as a command line argument.
Let's call the file name of this file fn. Your program must then produce as output the
following set of files:
• Your program must write the unigram counts to a file named fn.uni in which
each unigram is listed on a separate line, and each line contains just the
unigram and its count (an integer), separated by a single space.
• Your program must write the bigram counts to a file named fn.bi in which
each bigram is listed on a separate line, and each line contains just the
bigram and its count (an integer), separated by a single space.
Your program must write the conditional probabilities to a file named fn.cp,
reported in the form P(WORD(k)|WORD(k-1)) = p, where p is the conditional
probability of WORD(k) given WORD(k-1).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L