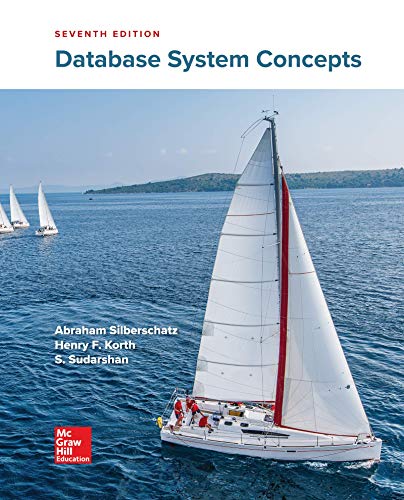
#include <iostream>
#include <assert.h>
using namespace std;
/*
The structure to be used for representing a doubly-linked link. This struct
declaration should not be modified in any way.
*/
template <class E>
struct DLink
{
E theElement;
DLink<E> *nextPtr;
DLink<E> *prevPtr;
};
/*
Complete this implementation variant of the doubly-linked list. Use the same convention. The DLink declaration to be used here is just a struct and should not be modified in any way. All of the operations of the original DLink class must be done in the methods of this class. Use
assertions to ensure the correct operation of this ADT. All memory allocations should be checked with assertions and all discarded memory must be properly deallocated.
*/
template <class E>
class DList
{
DLink<E> *head;
DLink<E> *tail;
DLink<E> *curr;
int cnt;
public:
// Return the size of the list
int length()
{
return cnt;
}
// The constructor with initial list size
DList(int size)
{
this();
}
// The default constructor
DList()
{
//
// ??? - implement this method
//
}
// The class destructor
~DList()
{
//
// ??? - implement this method
//
}
// Empty the list
void clear()
{
//
// ??? - implement this method
//
}
// Set current to first element
void moveToStart()
{
//
// ??? - implement this method
//
}
// Set current element to end of list
void moveToEnd()
{
//
// ??? - implement this method
//
}
// Advance current to the next element
void next()
{
//
// ??? - implement this method
//
}
// Return the current element
E getValue()
{
//
// ??? - implement this method
//
}
// Insert value at current position
void insert(E it)
{
//
// ??? - implement this method
//
}
// Append value at the end of the list
void append(E it)
{
//
// ??? - implement this method
//
}
// Remove and return the current element
E remove()
{
//
// ??? - implement this method
//
}
// Advance current to the previous element
void prev()
{
//
// ??? - implement this method
//
}
// Return position of the current element
int currPos()
{
//
// ??? - implement this method
//
}
// Set current to the element at the given position
void moveToPos(int pos)
{
//
// ??? - implement this method
//
}
};
/*
This is the main function for testing the implementation of the DList class.
This function can be freely modified.
*/
int main(void)
{
int i;
DList<int> theList;
// populate the list
for (i = 0; i < 10; ++i)
{
theList.append(i);
}
while (i < 20)
{
theList.insert(i);
++i;
}
// display the contents of the list
theList.moveToStart();
for (i = 0; i < theList.length(); ++i)
{
cout << theList.getValue() << " ";
theList.next();
}
cout << "\n";
// display the contents of the list in reverse order
theList.moveToEnd();
for (i = 0; i < theList.length(); ++i)
{
theList.prev();
cout << theList.getValue() << " ";
}
cout << "\n";
// replace the contents of the list
theList.clear();
for (i = 0; i < 10; ++i)
{
theList.append(i + 100);
}
// display the contents of the list
theList.moveToStart();
for (i = 0; i < theList.length(); ++i)
{
cout << theList.getValue() << " ";
theList.next();
}
cout << "\n";
// remove two elements at the specified position
theList.moveToPos(5);
cout << theList.currPos() << "\n";
theList.remove();
theList.remove();
// display the contents of the list
theList.moveToStart();
for (i = 0; i < theList.length(); ++i)
{
cout << theList.getValue() << " ";
theList.next();
}
cout << "\n";
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- /** * This class will use Nodes to form a linked list. It implements the LIFO * (Last In First Out) methodology to reverse the input string. * **/ public class LLStack { private Node head; // Constructor with no parameters for outer class public LLStack( ) { // to do } // This is an inner class specifically utilized for LLStack class, // thus no setter or getters are needed private class Node { private Object data; private Node next; // Constructor with no parameters for inner class public Node(){ // to do // to do } // Parametrized constructor for inner class public Node (Object newData, Node nextLink) { // to do: Data part of Node is an Object // to do: Link to next node is a type Node } } // Adds a node as the first node element at the start of the list with the specified…arrow_forward#include <iostream> usingnamespace std; class Queue { int size; int* queue; public: Queue(){ size = 0; queue = new int[100]; } void add(int data){ queue[size]= data; size++; } void remove(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } else{ for(int i =0; i < size -1; i++){ queue[i]= queue[i +1]; } size--; } } void print(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } for(int i =0; i < size; i++){ cout<<queue[i]<<" <- "; } cout << endl; } //your code goes here }; int main(){ Queue q1; q1.add(42); q1.add(2); q1.add(8); q1.add(1); Queue q2; q2.add(3); q2.add(66); q2.add(128); q2.add(5); Queue q3 = q1+q2; q3.print();…arrow_forward// FILL IN THE BLANKS (LINKED-LISTS CODE) (C++)#include<iostream>using namespace std; struct ________ {int data ;struct node *next; }; node *head = ________;node *createNode() { // allocate a memorynode __________;temp = new node ;return _______ ;} void insertNode(){node *temp, *traverse;int n;cout<< "Enter -1 to end "<<endl;cout<< "Enter the values to be added in list"<<endl;cin>>n; while(n!=-1){temp = createNode(); // allocate memorytemp->data = ________;temp->next = ________;if ( ___________ == NULL){head = _________;} else {traverse = ( );while (traverse->next != ________{traverse = traverse-> ___________;} traverse->next= temp;} cout<<"Enter the value to be added in the list"<<endl;cin>>n; }} void printlist(){node *traverse = head; // if head == NULLwhile (traverse != NULL) { cout<<traverse->data<<" ";traverse = traverse->next;}} int main(){int option; do{cout<<"\n =============== MAIN…arrow_forward
- 1. Write code to define LinkedQueue<T> and CircularArrayQueue<T> classes which willimplement QueueADT<T> interface. Note that LinkedQueue<T> and CircularArrayQueue<T>will use linked structure and circular array structure, respectively, to define the followingmethods.(i) public void enqueue(T element); //Add an element at the rear of a queue(ii) public T dequeue(); //Remove and return the front element of the queue(iii) public T first(); //return the front element of the queue without removing it(iv) public boolean isEmpty(); //Return True if the queue is empty(v) public int size(); //Return the number of elements in the queue(vi) public String toString();//Print all the elements of the queuea. Create an object of LinkedQueue<String> class and then add some names of your friendsin the queue and perform some dequeue operations. At the end, check the queue sizeand print the entire queue.b. Create an object from CircularArrayQueue<T> class and…arrow_forwardStack Implementation in C++make code for an application that uses the StackX class to create a stack.includes a brief main() code to test this class.arrow_forwardC++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.** MUST use given Template below: #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const {…arrow_forward
- what is the problem in the display function ? the code : #include <iostream>using namespace std; class node{public: int data; node* next;}; void display(node * head) // printing the data of the nodes{ node* temp = head; while (temp != NULL) { cout << temp->data << endl; temp = temp->next; }} // add void push(node** head, int newdata) // { 5, 10 , 20 , 30 , 40 }{ node* newnode = new node(); newnode->data = newdata; newnode->next = *head; *head = newnode;} void append(node** head, int newdata) // { 10 , 20 , 30 , 40 }{ node* newnode = new node(); newnode->data = newdata; newnode->next = NULL; node* last = *head; if (*head == NULL) { *head = newnode; return; } while (last->next != NULL) { last = last->next; } last->next = newnode;} void insertafter(node*…arrow_forwardgiven code lab4 #include <stdio.h>#include <stdlib.h> /* typical C boolean set-up */#define TRUE 1#define FALSE 0 typedef struct StackStruct{int* darr; /* pointer to dynamic array */int size; /* amount of space allocated */int inUse; /* top of stack indicator - counts how many values are on the stack */} Stack; void init (Stack* s){s->size = 2;s->darr = (int*) malloc ( sizeof (int) * s->size );s->inUse = 0;} void push (Stack* s, int val){/* QUESTION 7 *//* check if enough space currently on stack and grow if needed */ /* add val onto stack */s->darr[s->inUse] = val;s->inUse = s->inUse + 1;} int isEmpty (Stack* s){if ( s->inUse == 0)return TRUE;elsereturn FALSE;} int top (Stack* s){return ( s->darr[s->inUse-1] );} /* QUESTION 9.1 */void pop (Stack* s){if (isEmpty(s) == FALSE)s->inUse = s->inUse - 1;} void reset (Stack* s){/* Question 10: how to make the stack empty? */ } int main (int argc, char** argv){Stack st1; init (&st1);…arrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
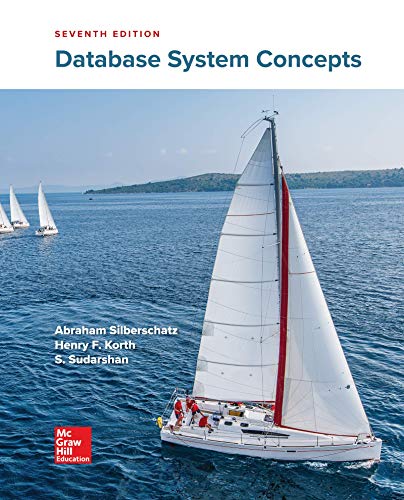
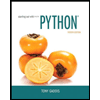
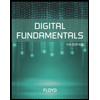
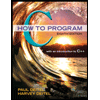
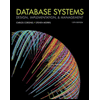
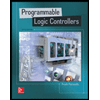