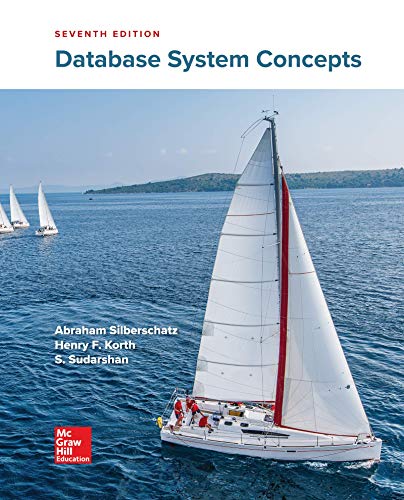
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Write the code necessary to convert the following sequence of ListNode objects:
list -> [5] -> [4] -> [3] /
Into this sequence of ListNode objects:
list -> [3] -> [4] -> [5] /
Assume that you are using ListNode class as defined in lecture and section:
public class ListNode { public int data; // data stored in this node public ListNode next; // a link to the next node in the list public ListNode() { ... } public ListNode(int data) { ... } public ListNode(int data, ListNode next) { ... } }
![LinkedIntList.java
Marty Stepp
Related Links:
Author:
Write the code necessary to convert the following sequence of ListNode objects:
list -> [5]
-> [4]
-> [3] /
Into this sequence of ListNode objects:
list -> [3]
-> [4]
-> [5] /
Assume that you are using ListNode class as defined in lecture and section:
public class ListNode {
public int data;
public ListNode next;
// data stored in this node
// a link to the next node in the list
public ListNode() { ... }
public ListNode(int data) { ... }
public ListNode(int data, ListNode next) { ... }
}
Type your solution here:](https://content.bartleby.com/qna-images/question/5a5fe103-4e76-4218-8305-c82815ca5912/6dd2ed51-c87e-4393-ad8b-c4ef6fc18537/xsk0rc_thumbnail.png)
Transcribed Image Text:LinkedIntList.java
Marty Stepp
Related Links:
Author:
Write the code necessary to convert the following sequence of ListNode objects:
list -> [5]
-> [4]
-> [3] /
Into this sequence of ListNode objects:
list -> [3]
-> [4]
-> [5] /
Assume that you are using ListNode class as defined in lecture and section:
public class ListNode {
public int data;
public ListNode next;
// data stored in this node
// a link to the next node in the list
public ListNode() { ... }
public ListNode(int data) { ... }
public ListNode(int data, ListNode next) { ... }
}
Type your solution here:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- template <class T> class List; template <class T> class Node{ friend class List<T>; private: T data; Node* link; }; template <class T> class List{ public: List(){first = 0;} void InsertBack(const T& e); void Concatenate(List<T>& b); void Reverse(); class Iterator{ …. }; Iterator Begin(); Iterator End(); private: Node* first; }; I need algorithm , I think it may use iterator to complile. The question shows on below photo.arrow_forwardpackage hw5; public class LinkedIntSet {private static class Node {private int data;private Node next; public Node(int data, Node next) {this.data = data;this.next = next;}} private Node first; // Always points to the first node of the list.// THE LIST IS ALWAYS IN SORTED ORDER!private int size; // Always equal to the number of elements in the set. /*** Construts an empty set.*/public LinkedIntSet() {throw new RuntimeException("Not implemented");} /*** Returns the number of elements in the set.* * @return the number of elements in the set.*/public int size() {throw new RuntimeException("Not implemented");} /*** Tests if the set contains a number* * @param i the number to check* @return <code>true</code> if the number is in the set and <code>false</code>* otherwise.*/public boolean contains(int i) {throw new RuntimeException("Not implemented");} /*** Adds <code>element</code> to this set if it is not already present and* returns…arrow_forwardhello. This is my code: #include <stdio.h>#include <stdlib.h>#include <string.h> typedef struct HuffmanNode { int character; float frequency; struct HuffmanNode* left; struct HuffmanNode* right;} HuffmanNode; typedef struct HeapNode { HuffmanNode* node; struct HeapNode* next;} HeapNode; HuffmanNode* create_huffman_node(int character, float frequency) { HuffmanNode* node = (HuffmanNode*)malloc(sizeof(HuffmanNode)); node->character = character; node->frequency = frequency; node->left = NULL; node->right = NULL; return node;} HeapNode* create_heap_node(HuffmanNode* node) { HeapNode* heap_node = (HeapNode*)malloc(sizeof(HeapNode)); heap_node->node = node; heap_node->next = NULL; return heap_node;} HeapNode* insert_into_heap(HeapNode* heap, HuffmanNode* node) { HeapNode* heap_node = create_heap_node(node); if (heap == NULL) { return heap_node; } // Compare nodes based on probability and…arrow_forward
- Lab 19 Building a linked list Start this lab with the code listed below. The LinkedList class defines the rudiments of the code needed to build a linked list of Node objects. You will first complete the code for its addFirst method. This method is passed an object that is to be added to the beginning of the list. Write code that links the passed object to the list by completing the following tasks in order: 1. Create a new Node object. 2. Make the data variable in the new Node object reference the object that was passed to addFirst. 3. Make the next variable in the new Node object reference the object that is currently referenced in variable first. 4. Make variable first reference the new Node. Test your code by running the main method in the LinkedListRunner class below. Explain, step by step, why each of the above operations is necessary. Why are the string objects in the reverse order from the way they were added? public class LinkedList { private Node first; public LinkedList () {…arrow_forwardAssignment1: Analyse the case study given in chapter 3 Linked listAnswer the following questions from Library.java1. Write the different class names with class keyword chapter 3 Linked list : https://drive.google.com/file/d/1NRN_3Fx3smkjwv1jgG8hzaiGfnNfeeDb/view?usp=sharingarrow_forwardTree Define a class called TreeNode containing three data fields: element, left and right. The element is a generic type. Create constructors, setters and getters as appropriate. Define a class called BinaryTree containing two data fields: root and numberElement. Create constructors, setters and getters as appropriate. Define a method balanceCheck to check if a tree is balanced. A tree being balanced means that the balance factor is -1, 0, or 1.arrow_forward
- Assume you have a class SLNode representing a node in a singly-linked list and a variable called list referencing the first element on a list of integers, as shown below: public class SLNode { private E data; private SLNode next; public SLNode( E e){ data = e; next = null; } public SLNode getNext() { return next; } public void setNext( SLNoden){ next = n; } } SLNode list; Write a fragment of Java code that would append a new node with data value 21 at the end of the list. Assume that you don't know if the list has any elements in it or not (i.e., it may be empty). Do not write a complete method, but just show a necessary fragment of code.arrow_forward#include <iostream> usingnamespace std; class Queue { int size; int* queue; public: Queue(){ size = 0; queue = new int[100]; } void add(int data){ queue[size]= data; size++; } void remove(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } else{ for(int i =0; i < size -1; i++){ queue[i]= queue[i +1]; } size--; } } void print(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } for(int i =0; i < size; i++){ cout<<queue[i]<<" <- "; } cout << endl; } //your code goes here }; int main(){ Queue q1; q1.add(42); q1.add(2); q1.add(8); q1.add(1); Queue q2; q2.add(3); q2.add(66); q2.add(128); q2.add(5); Queue q3 = q1+q2; q3.print();…arrow_forward1 Assume some Node class with info & link fields. Complete this method in class List that returns a reference to the node containing the data item in the argument find This, Assume that find this is in the list public class List { protected Node head; protected int size; fublic Public Node find (char find This)arrow_forward
- In java The following is a class definition of a linked list Node:class Node{int info;Node next;}Assume that head references a linked list and stores in order, the int values 5, 7 and 9. Show the instructions needed to delete the Node with 5 so that head would reference the list 7 and 9.arrow_forwardwhat is the problem in the display function ? the code : #include <iostream>using namespace std; class node{public: int data; node* next;}; void display(node * head) // printing the data of the nodes{ node* temp = head; while (temp != NULL) { cout << temp->data << endl; temp = temp->next; }} // add void push(node** head, int newdata) // { 5, 10 , 20 , 30 , 40 }{ node* newnode = new node(); newnode->data = newdata; newnode->next = *head; *head = newnode;} void append(node** head, int newdata) // { 10 , 20 , 30 , 40 }{ node* newnode = new node(); newnode->data = newdata; newnode->next = NULL; node* last = *head; if (*head == NULL) { *head = newnode; return; } while (last->next != NULL) { last = last->next; } last->next = newnode;} void insertafter(node*…arrow_forward1.Assume some Node class with info link fields . Complete this method in class List that returns a reference to the node containing the data item in the argument findThis, Assume that findThis is in the list public class list { protected Node head ; Protected int size ; Public Node find (char find this) { Node curr = head; while(curr != null) { if(curr.info == findThis) return curr; curr = curr.link; } return -1; } }arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
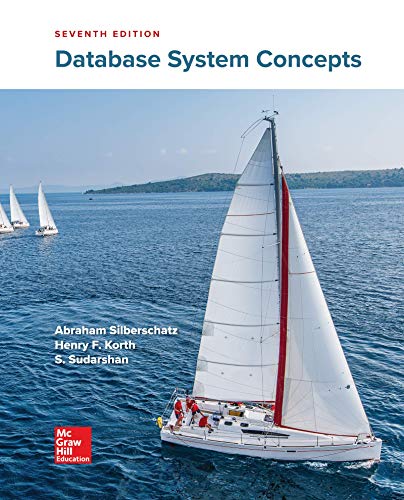
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
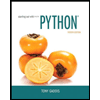
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
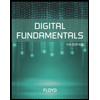
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
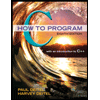
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
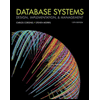
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
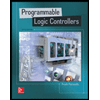
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education