Instructions Study the prewritten code to make sure you understand it. Write the code that searches the array for the name of the add-in ordered by the customer. Write the code that prints the name and price of the add-in or the error message, and then write the code that prints the cost of the total order. Execute the program by clicking the Run button at the bottom of the screen. Use the following data: Cream Caramel Whiskey chocolate Chocolate Cinnamon Vanilla GIVEN CODE // JumpinJava.cpp - This program looks up and prints the names and prices of coffee orders. // Input: Interactive // Output: Name and price of coffee orders or error message if add-in is not found #include #include using namespace std; int main() { // Declare variables. string addIn; // Add-in ordered const int NUM_ITEMS = 5; // Named constant // Initialized array of add-ins string addIns[] = {"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"}; // Initialized array of add-in prices double addInPrices[] = {.89, .25, .59, 1.50, 1.75}; bool foundIt = false; // Flag variable int x; // Loop control variable double orderTotal = 2.00; // All orders start with a 2.00 charge // Get user input cout << "Enter coffee add-in or XXX to quit: "; cin >> addIn; // Write the rest of the program here. return 0; } // End of main()
In this lab, you use what you have learned about parallel arrays to complete a partially completed C++
- Either print the name and price for a coffee add-in from the Jumpin’ Jive Coffee Shop
- Or it should print the message Sorry, we do not carry that.
Read the problem description carefully before you begin. The file provided for this lab includes the necessary variable declarations and input statements. You need to write the part of the program that searches for the name of the coffee add-in(s) and either prints the name and price of the add-in or prints the error message if the add-in is not found. Comments in the code tell you where to write your statements.
Instructions
- Study the prewritten code to make sure you understand it.
- Write the code that searches the array for the name of the add-in ordered by the customer.
- Write the code that prints the name and price of the add-in or the error message, and then write the code that prints the cost of the total order.
-
Execute the program by clicking the Run button at the bottom of the screen. Use the following data:
Cream
Caramel
Whiskey
chocolate
Chocolate
Cinnamon
Vanilla
GIVEN CODE

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

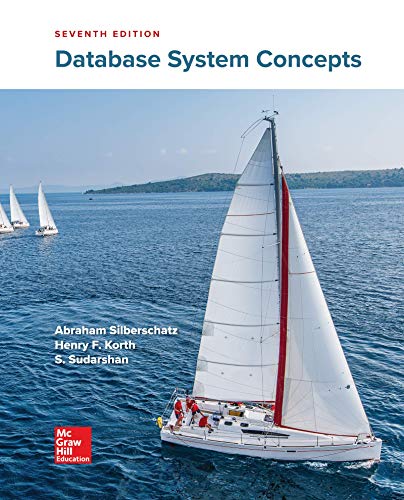
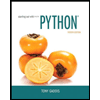
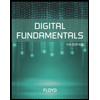
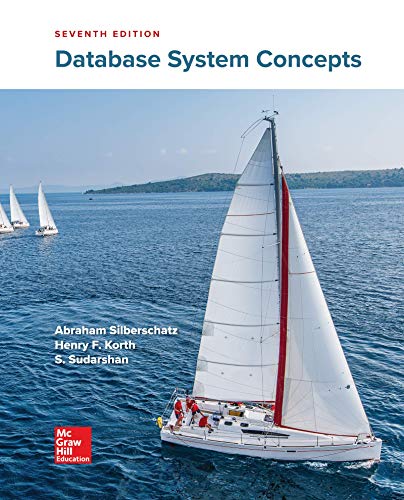
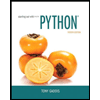
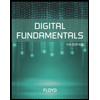
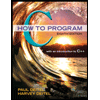
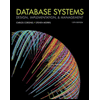
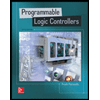