It has just one Main class which tests abstract class Animal and its 3 subclasses: Dog, Cat, and Fish. It also tests the Talkers: Dog, Cat, and Radio. So your job is to write all 6 of these simple classes (they should be less than one page each)
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
It has just one Main class which tests abstract class Animal and its 3 subclasses: Dog, Cat, and Fish. It also tests the Talkers: Dog, Cat, and Radio. So your job is to write all 6 of these simple classes (they should be less than one page each) :
- Talker.java - the interface Talker, which has just one void method called speak()
- Animal.java - the abstract class Animal, which stores an animal's name. (No abstract methods). It should contain 2 methods:
- a constructor with 1 argument (the name)
- a method getName() which returns the name.
- Dog.java - the class Dog, which extends Animal and implements Talker. It should contain 3 methods:
- a constructor with no arguments, giving the dog a default name of "Fido"
- a constructor with 1 argument (the name)
- a speak() method that prints "Woof" on the screen. Use @Override
- Cat.java - the class Cat, which extends Animal and implements Talker. It should contain just 2 methods:
- a constructor with 1 argument (the name) (no default name like dogs have)
- a speak() method that prints "Meow" on the screen. Use @Override
- Fish.java - the class Fish, which is an Animal that doesn't talk. So it only needs 1 constructor (with its name).
- Radio.java - the class Radio, which is a Talker, but not an animal. Its @Override speak() method should print "blahblahblah"

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 7 images

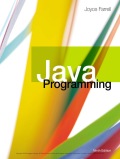
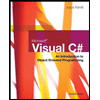
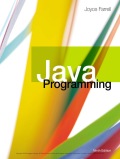
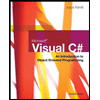