Consider the class Arthropod and the interface Edible as described below: public class Arthropod private String species public Arthropod (String type) species - type: public Arthropod (Arthropod x) species x.species: public String tostring () return ("* + species + ""); public interface Edible public int cookTime (): // time to cook in minutes Implement a class called Lobster that extends the class Arthropod and implements the Edible interface. It has a single private instance variable of type int, called claws. Your Lobster class should contain the following public methods, implemented in the order listed below. 1. Constructor with one parameter. This constructor takes one int argument, which represents the claws value for the Lobster . It will initialize the field claws to be equal to the parameter, and the field species will be set equal to the String "Lobster 2. Constructor with no parameters. This constructor will initialize a Lobster with a claws value of 2, and species equal to "Lobster". You must call the constructor from part (1) above in your implementation of this constructor. 3. Copy constructor. You must call the copy constructor of the Arthropod class in your implementation. 4. equals. To be equal means that both Lobster must have the same claws value and the same species value. 5. toString. Write a toStringmethod for this class which returns a String containing both the species and the claws in some reasonable format. 6. possibly other method(s) that are required because of the fact that this class extends Arthropod and/or implements Edible. You will lose points if you implement methods that are not necessary. If you do implement any method(s) here, just invent some very simple implementation.
Consider the class Arthropod and the interface Edible as described below: public class Arthropod private String species public Arthropod (String type) species - type: public Arthropod (Arthropod x) species x.species: public String tostring () return ("* + species + ""); public interface Edible public int cookTime (): // time to cook in minutes Implement a class called Lobster that extends the class Arthropod and implements the Edible interface. It has a single private instance variable of type int, called claws. Your Lobster class should contain the following public methods, implemented in the order listed below. 1. Constructor with one parameter. This constructor takes one int argument, which represents the claws value for the Lobster . It will initialize the field claws to be equal to the parameter, and the field species will be set equal to the String "Lobster 2. Constructor with no parameters. This constructor will initialize a Lobster with a claws value of 2, and species equal to "Lobster". You must call the constructor from part (1) above in your implementation of this constructor. 3. Copy constructor. You must call the copy constructor of the Arthropod class in your implementation. 4. equals. To be equal means that both Lobster must have the same claws value and the same species value. 5. toString. Write a toStringmethod for this class which returns a String containing both the species and the claws in some reasonable format. 6. possibly other method(s) that are required because of the fact that this class extends Arthropod and/or implements Edible. You will lose points if you implement methods that are not necessary. If you do implement any method(s) here, just invent some very simple implementation.
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 20RQ
Related questions
Question
java
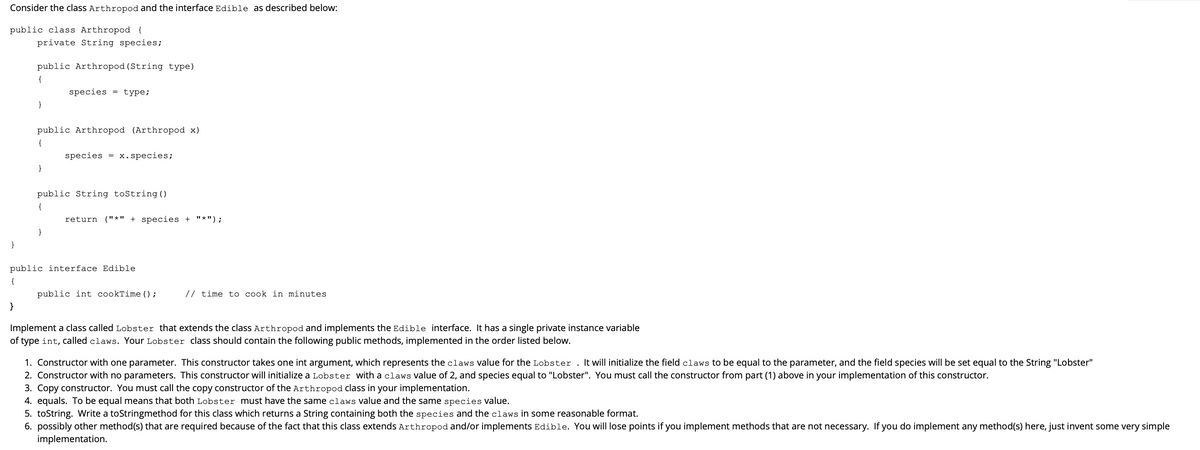
Transcribed Image Text:Consider the class Arthropod and the interface Edible as described below:
public class Arthropod {
private String species;
public Arthropod (String type)
{
species = type;
}
public Arthropod (Arthropod x)
{
species
x.species;
=
}
public String toString()
{
return ("*" + species + "*");
}
}
public interface Edible
{
public int cookTime () ;
// time to cook in minutes
}
Implement a class called Lobster that extends the class Arthropod and implements the Edible interface. It has a single private instance variable
of type int, called claws. Your Lobster class should contain the following public methods, implemented in the order listed below.
1. Constructor with one parameter. This constructor takes one int argument, which represents the claws value for the Lobster . It will initialize the field claws to be equal to the parameter, and the field species will be set equal to the String "Lobster"
2. Constructor with no parameters. This constructor will initialize a Lobster with a claws value of 2, and species equal to "Lobster". You must call the constructor from part (1) above in your implementation of this constructor.
3. Copy constructor. You must call the copy constructor of the Arthropod class in your implementation.
4. equals. To be equal means that both Lobster must have the same claws value and the same species value.
5. toString. Write a toStringmethod for this class which returns a String containing both the species and the claws in some reasonable format.
6. possibly other method(s) that are required because of the fact that this class extends Arthropod and/or implements Edible. You will lose points if you implement methods that are not necessary. If you do implement any method(s) here, just invent some very simple
implementation.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
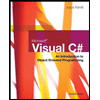
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
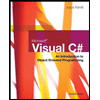
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,