It is often said that nothing in life is certain but death and taxes. For a programmer or data scientist, however, nothir certain but encountering errors. In Python, there are two primary types of errors, both of which you are likely familiar with: syntax errors and exceptic Syntax errors occur when the proper structure of the language is not followed, while exceptions are errors that occu the execution of a program. These include errors such as ZeroDivision Error, TypeError, NameError, and many more! Under the hood, these errors are based in the concepts of object orientation, and all exceptions are class objects. If y interested in more detailed explanations of the structure of exceptions as well as how to create your own, check out from the Python documentation! In the meantime, we'll implement our own version of an Error class Complete the Error, SyntaxError, and ZeroDivision Error classes such that they create the correct messages called. • The SyntaxError and ZeroDivisionError classes inherit from the Error class and add functionality that is to those particular errors. Their code is partially implemented for you. • The add_code method adds a new helpful message to your error, while the write method should print the out you see when an error is raised. Do not worry if that code already is already defined; for this problem, it is safe to overwrite it. . You can access the parent class methods using the super function class Error: PERIO >>> err1 = Error (12, "error.py") >>> errl.write() error.py: 12 WHE definit__(self, line, file): "*** YOUR CODE HERE ****" def format (self): def write(self): *** return self.file + : + str(self.line) class Syntax Error (Error): print (self.format()) >>>erri = SyntaxError(17, "lable.py") >>>erri.write() #I# lab10.py: 17 SyntaxError: Invalid syntax >>>erri.add_code(4, "EOL while scanning string literal") >>>err2 = SyntaxError (18, "lab10.py", 4) >>>err2.write() lable.py:18 SyntaxError: EOL while scanning string literal type= 'SyntaxError' msgs (8: "Invalid syntax", 1: "Unmatched parentheses", 2: "Incorrect indentation", 3: "missing ca definit__(self, line, file, code=0): "*** YOUR CODE HERE ******" def format (self): "**YOUR CODE HERE ******** def add_code(self, code, msg): "*** YOUR CODE HERE ***" WICH class ZeroDivisionError(Error): >>>err1 = ZeroDivisionError(273, "lab10.py") Derrl.write() lable.py: 273 ZeroDivisionError: division by zero type = 'ZeroDivisionError' def _init__(self, line, file, message='division by zero'); " YOUR CODE HERE ***" def format (self): end self.type+:+self.message "YOUR CODE HERE " Use OK to test your code:
It is often said that nothing in life is certain but death and taxes. For a programmer or data scientist, however, nothir certain but encountering errors. In Python, there are two primary types of errors, both of which you are likely familiar with: syntax errors and exceptic Syntax errors occur when the proper structure of the language is not followed, while exceptions are errors that occu the execution of a program. These include errors such as ZeroDivision Error, TypeError, NameError, and many more! Under the hood, these errors are based in the concepts of object orientation, and all exceptions are class objects. If y interested in more detailed explanations of the structure of exceptions as well as how to create your own, check out from the Python documentation! In the meantime, we'll implement our own version of an Error class Complete the Error, SyntaxError, and ZeroDivision Error classes such that they create the correct messages called. • The SyntaxError and ZeroDivisionError classes inherit from the Error class and add functionality that is to those particular errors. Their code is partially implemented for you. • The add_code method adds a new helpful message to your error, while the write method should print the out you see when an error is raised. Do not worry if that code already is already defined; for this problem, it is safe to overwrite it. . You can access the parent class methods using the super function class Error: PERIO >>> err1 = Error (12, "error.py") >>> errl.write() error.py: 12 WHE definit__(self, line, file): "*** YOUR CODE HERE ****" def format (self): def write(self): *** return self.file + : + str(self.line) class Syntax Error (Error): print (self.format()) >>>erri = SyntaxError(17, "lable.py") >>>erri.write() #I# lab10.py: 17 SyntaxError: Invalid syntax >>>erri.add_code(4, "EOL while scanning string literal") >>>err2 = SyntaxError (18, "lab10.py", 4) >>>err2.write() lable.py:18 SyntaxError: EOL while scanning string literal type= 'SyntaxError' msgs (8: "Invalid syntax", 1: "Unmatched parentheses", 2: "Incorrect indentation", 3: "missing ca definit__(self, line, file, code=0): "*** YOUR CODE HERE ******" def format (self): "**YOUR CODE HERE ******** def add_code(self, code, msg): "*** YOUR CODE HERE ***" WICH class ZeroDivisionError(Error): >>>err1 = ZeroDivisionError(273, "lab10.py") Derrl.write() lable.py: 273 ZeroDivisionError: division by zero type = 'ZeroDivisionError' def _init__(self, line, file, message='division by zero'); " YOUR CODE HERE ***" def format (self): end self.type+:+self.message "YOUR CODE HERE " Use OK to test your code:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:It is often said that nothing in life is certain but death and taxes. For a programmer or data scientist, however, nothing is
certain but encountering errors.
In Python, there are two primary types of errors, both of which you are likely familiar with: syntax errors and exceptions.
Syntax errors occur when the proper structure of the language is not followed, while exceptions are errors that occur during
the execution of a program. These include errors such as ZeroDivision Error, TypeError, NameError, and many more!
Under the hood, these errors are based in the concepts of object orientation, and all exceptions are class objects. If you're
interested in more detailed explanations of the structure of exceptions as well as how to create your own, check out this article
from the Python documentation! In the meantime, we'll implement our own version of an Error class.
Complete the Error, SyntaxError, and ZeroDivisionError classes such that they create the correct messages when
called.
• The SyntaxError and ZeroDivisionError classes inherit from the Error class and add functionality that is unique
to those particular errors. Their code is partially implemented for you.
• The add_code method adds a new helpful message to your error, while the write method should print the output that
you see when an error is raised. Do not worry if that code already is already defined; for this problem, it is safe to
overwrite it.
• You can access the parent class methods using the super function
class Error:
ANE
>>> err1 = Error (12, "error.py")
>>>errl.write()
error.py: 12
67 51 37
def _init__(self, line, file):
** YOUR CODE HERE *****"
def format (self):
no
def write(self):
as TETE
return self.file + : + str(self.line)
class SyntaxError (Error):
print (self.format())
>>> err1 = SyntaxError(17, "lab10.py")
>>>errl.write()
lab10.py: 17 SyntaxError : Invalid syntax
>>>erri.add_code(4, "EOL while scanning string literal")
>>> err2 = SyntaxError (18, "lab10.py", 4)
>>> err2.write()
lab10.py: 18 SyntaxError: EOL while scanning string literal
type = 'SyntaxError'
msgs (0 : "Invalid syntax", 1: "Unmatched parentheses", 2: "Incorrect indentation", 3: "missing col
def _init_(self, line, file, code=0):
"*** YOUR CODE HERE ****"
def format (self):
ID BELE
ROICHE
*** YOUR CODE HERE ******"
def add_code(self, code, msg):
"*** YOUR CODE HERE
"
10
class ZeroDivisionError(Error);
>>> errl = ZeroDivisionError(273, "lab10.py")
Derrl.write()
lable.py: 273 ZeroDivisionError: division by zero
type = 'ZeroDivisionError'
def __init__(self, line, file, message='division by zero');
"* YOUR CODE HERE ****
def format (self):
end = self.type + ' :
" YOUR CODE HERE
Use OK to test your code:
(
01
+ self.message
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
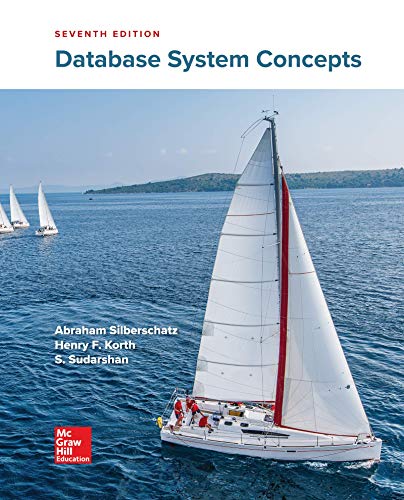
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
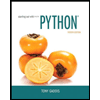
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
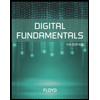
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
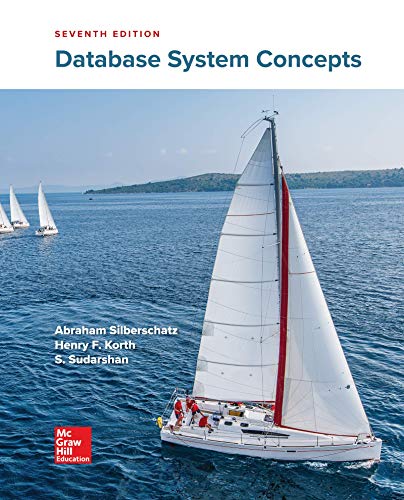
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
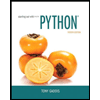
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
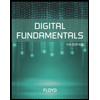
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
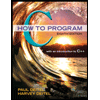
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
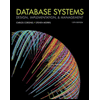
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
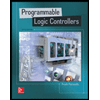
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education