This is a debugging question - The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. Code I was given - // Catch exceptions for array index out of bounds // or dividing by 0 import java.util.*; public class DebugTwelve3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String inStr; int num, result; int[] array = {12, 4, 6, 8}; System.out.println("Enter a number ") inStr = input; num = Integer.parse(inStr); try { for(int x = 0; x < array.length; ++x) { result = array[x] / num; System.out.println("Result of division is " + result); result = array[num]; System.out.println("Result accessing array is " + result); } } catch(ArithmeticException error) { System.out.println("Arithmetic error - division by 0"); } catch(IndexException error) { System.out.println("Index error - subscript out of range"); } } }
This is a debugging question - The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. Code I was given - // Catch exceptions for array index out of bounds // or dividing by 0 import java.util.*; public class DebugTwelve3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String inStr; int num, result; int[] array = {12, 4, 6, 8}; System.out.println("Enter a number ") inStr = input; num = Integer.parse(inStr); try { for(int x = 0; x < array.length; ++x) { result = array[x] / num; System.out.println("Result of division is " + result); result = array[num]; System.out.println("Result accessing array is " + result); } } catch(ArithmeticException error) { System.out.println("Arithmetic error - division by 0"); } catch(IndexException error) { System.out.println("Index error - subscript out of range"); } } }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter14: Exception Handling
Section: Chapter Questions
Problem 3PE
Related questions
Question
This is a debugging question -
The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly.
Code I was given -
// Catch exceptions for array index out of bounds
// or dividing by 0
import java.util.*;
public class DebugTwelve3
{
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
String inStr;
int num, result;
int[] array = {12, 4, 6, 8};
System.out.println("Enter a number ")
inStr = input;
num = Integer.parse(inStr);
try
{
for(int x = 0; x < array.length; ++x)
{
result = array[x] / num;
System.out.println("Result of division is " + result);
result = array[num];
System.out.println("Result accessing array is " + result);
}
}
catch(ArithmeticException error)
{
System.out.println("Arithmetic error - division by 0");
}
catch(IndexException error)
{
System.out.println("Index error - subscript out of range");
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
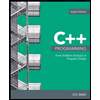
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
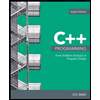
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning