Background "dish information" review We discussed in the previous lab what goes into a dish's information, but it's here too, for easy reference. In this project, a dish's information is a dictionary object that is guaranteed to have the following keys: "name": a string that stores the dish's name. "calories": an integer representing the calorie intake for one serving of the dish. "price": a float to represent the cost of the dish. "is_vegetarian": a string ( yes/no) to check if the dish is vegetarian. "spicy_level": an integer from 1 to 4 representing the level of spiciness of the dish. An example dictionary with a single dish's information could look like: Your job is to write a function that takes a list of strings get_new_menu_dish(menu_dish_list, spicy_scale_map) that will create a new dictionary with the structure described above and in the previous lab. For instance, when we pass following arguments to the function : ["Monster Burrito", "800", "16.50", "no", "2"] and {1: 'not spicy', 2: 'mild'} , the function should return the dictionary {"name":"Monster Burrito", "calories": 800, "price": 16.50, "is_vegetarian": "no", "spicy_level": 2}. If the length of the list is wrong, return the length of the list. If one particular field is invalid, return a tuple of its name and the value it had. However, in addition you must validate the list's elements representing the dish's attributes one at a time before transforming it into a dictionary, ensuring it never crashes regardless of the input. In order to do this, we ask you to add several functions to the code that validate name, calories, price, spice level, and vegetarian status. The list of functions to implement is as follows. Examples are included for some. is_num Takes in a string and checks if it represents a number (either an integer or a decimal). We will use this function to check if the value entered for the 'price' field is valid or not. assert is_num("6.7") == True assert is_num("12r9") == False is_valid_name Takes in the name of a dish and checks whether it is the right length - between 3 and 25 characters, inclusive of both. is_valid_spicy_level Takes in a string representing level of spiciness as an int, as well as a dictionary that maps levels of spiciness to their english description, and checks whether the string is a valid level of spiciness according to the dict. is_valid_is_vegetarian Takes in a string representing whether a dish is vegetarian and checks whether it is either "yes" or "no". is_valid_calories Takes in a string representing a dish's calories, checks that it corresponds to an integer. is_valid_price Takes in a string representing a dish's price, checks whether its a numerical value or not by calling is_num function inside it. In each of these, you need to validate both that each of these arguments is a string (or list of strings, depending) and then return a boolean (either True or False) depending on whether the conditions for validity are met.
Background
"dish information" review
We discussed in the previous lab what goes into a dish's information, but it's here too, for easy reference. In this project, a dish's information is a dictionary object that is guaranteed to have the following keys:
- "name": a string that stores the dish's name.
- "calories": an integer representing the calorie intake for one serving of the dish.
- "price": a float to represent the cost of the dish.
- "is_vegetarian": a string ( yes/no) to check if the dish is vegetarian.
- "spicy_level": an integer from 1 to 4 representing the level of spiciness of the dish.
An example dictionary with a single dish's information could look like:
Your job is to write a function that takes a list of strings get_new_menu_dish(menu_dish_list, spicy_scale_map) that will create a new dictionary with the structure described above and in the previous lab.
For instance, when we pass following arguments to the function : ["Monster Burrito", "800", "16.50", "no", "2"] and {1: 'not spicy', 2: 'mild'} , the function should return the dictionary {"name":"Monster Burrito", "calories": 800, "price": 16.50, "is_vegetarian": "no", "spicy_level": 2}.
If the length of the list is wrong, return the length of the list. If one particular field is invalid, return a tuple of its name and the value it had.
However, in addition you must validate the list's elements representing the dish's attributes one at a time before transforming it into a dictionary, ensuring it never crashes regardless of the input.
In order to do this, we ask you to add several functions to the code that validate name, calories, price, spice level, and vegetarian status.
The list of functions to implement is as follows. Examples are included for some.
- is_num Takes in a string and checks if it represents a number (either an integer or a decimal). We will use this function to check if the value entered for the 'price' field is valid or not.
- assert is_num("6.7") == True
- assert is_num("12r9") == False
- is_valid_name Takes in the name of a dish and checks whether it is the right length - between 3 and 25 characters, inclusive of both.
- is_valid_spicy_level Takes in a string representing level of spiciness as an int, as well as a dictionary that maps levels of spiciness to their english description, and checks whether the string is a valid level of spiciness according to the dict.
- is_valid_is_vegetarian Takes in a string representing whether a dish is vegetarian and checks whether it is either "yes" or "no".
- is_valid_calories Takes in a string representing a dish's calories, checks that it corresponds to an integer.
- is_valid_price Takes in a string representing a dish's price, checks whether its a numerical value or not by calling is_num function inside it.
In each of these, you need to validate both that each of these arguments is a string (or list of strings, depending) and then return a boolean (either True or False) depending on whether the conditions for validity are met.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

It outputs as
Maybe this will help this was my code and i got 11/16 for it but my test feed back for get_new_menu_dish (valid) was:
When given the argument(s) [['burrito', '500', '12.90', 'yes', '2'], {0: 'a', 1: 'b', 2: 'c', 3: 'd', 44: 'e', 5: 'f', 6: 'g', 7: 'h'}], We got a return value dish_list instead of {'name': 'burrito', 'calories': 500, 'price': 12.9, 'is_vegetarian': 'yes', 'spicy_level': 2}
and my other feed back was forr get_new_menu_dish (iinvalid) was:
Input : ['burrito', '500', '12.90', 'True', '2'] Your Output : dish_list Expected Output : ('is_vegetarian', 'True')
My code:
def is_num(val):
if not isinstance(val, str):
return False
try:
float(val)
return True
except ValueError:
return False
def is_valid_name(name_str):
if not isinstance(name_str, str):
return False
if len(name_str) < 3 or len(name_str) > 25:
return False
return True
def is_valid_spicy_level(spicy_level_str, spicy_scale_map):
if not isinstance(spicy_level_str, str):
return False
if not is_num(spicy_level_str):
return False
spicy_level = int(float(spicy_level_str))
return spicy_level in spicy_scale_map.keys()
def is_valid_is_vegetarian(vegetarian_str):
if not isinstance(vegetarian_str, str):
return False
return vegetarian_str.lower() in ['yes', 'no']
def is_valid_price(price_str):
if not isinstance(price_str, str):
return False
return is_num(price_str)
def is_valid_calories(calories_str):
if not isinstance(calories_str, str):
return False
return calories_str.isdigit()
def get_new_menu_dish(dish_list, spicy_scale_map):
if not isinstance(dish_list, list) or len(dish_list) != 4:
return "dish_list"
name, calories_str, price_str, vegetarian_str, spicy_level_str = dish_list
if not is_valid_name(name):
return "name"
if not is_valid_calories(calories_str):
return "calories"
if not is_valid_price(price_str):
return "price"
if not is_valid_is_vegetarian(vegetarian_str):
return "is_vegetarian"
if not is_valid_spicy_level(spicy_level_str, spicy_scale_map):
return "spicy_level"
spicy_level = int(float(spicy_level_str))
return {"name": name, "calories": int(calories_str), "price": float(price_str), "is_vegetarian": vegetarian_str.lower() == True , "spicy_level": spicy_scale_map[spicy_level]}
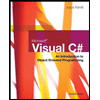
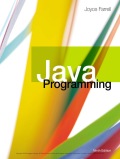
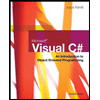
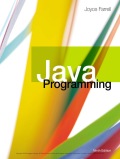