The three parallel arrays are gone — replaced with a single array of type PhonebookEntry. You should be reading in the entries using the read method of your PhonebookEntry class (which in turn uses the read methods of the Name and PhoneNumber classes). Use the equals methods of the Name and PhoneNumber classes in your lookup and reverseLookup methods. Use the toString methods to print out information. Make 100 the capacity of your Phonebook array Throw an exception (of class Exception) if the capacity of the Phonebook array is exceeded. Place a try/catch around your entire main and catch both FileNotFoundExceptions and Exceptions (remember, the order of appearance of the exception types in the catch blocks can make a difference). Do not use BufferedReader while(true) breaks The name of your application class should be Phonebook. Also, you should submit ALL your classes (i.e., Name, Strip off public from all your class definintions name class: import java.io.*; import java.util.*; public class Name { public Name(String last, String first) { this.last = last; this.first = first; } public Name(String first) { this("", first); } public boolean equals(Name same) { return first.equals(same.first) && last .equals(same.last); } public String toString() { return first + " " + last; } public String getFormal() { return first + " " + last; } public String getOfficial() { return last + ", " + first; } public static Name read(Scanner scan) { if (!scan.hasNext()) return null; String first = scan.next(); String last = scan.next(); return new Name(first, last); } public String getInitials() { return Character.toUpperCase(first.charAt(0)) + "." + Character.toUpperCase(last.charAt(0))+"."; } private String last, first; public static void main(String [] args) throws Exception { Scanner scan = new Scanner(new File("names.text")); int count = 0; Name readName = read(scan); Name readLastName =null; while(readName != null) { if(readLastName!=null && readName!=null && readLastName.equals(readName)){ System.out.println("Duplicate name \""+readName+"\" discovered"); } else{ System.out.println("name: " + readName); System.out.println("formal: " + readName.getFormal()); System.out.println("official: " + readName.getOfficial()); System.out.println("initials: " + readName.getInitials()); System.out.println(); } count++; readLastName= readName; readName = read(scan); } System.out.println("---"); System.out.println(count + " names processed."); } } Phonenumber class: import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class PhoneNumber { private String num; public PhoneNumber(String num) { this.num = num; } public String getAreaCode() { return num.substring(1, 4); } public String getExchange() { return num.substring(5, 8); } public String getLineNumber() { return num.substring(9); } public boolean isFreeToll() { if (getAreaCode().charAt(0) == '8') { return true; } return false; } public String toString() { return "phone number: " + num + "\narea code: " + getAreaCode() + "\nexchange: " + getExchange() + "\nline number: " + getLineNumber(); } public boolean equals(PhoneNumber phoneNum) { if (phoneNum.getAreaCode().compareTo(this.getAreaCode()) == 0 && phoneNum.getExchange().compareTo(this.getExchange()) == 0 && phoneNum.getLineNumber().compareTo(this.getLineNumber()) == 0) { return true; } return false; } public static String read(Scanner scan) { if (scan.hasNextLine()) { return scan.nextLine(); } return null; } public static void main(String[] args) throws FileNotFoundException { Scanner scan = new Scanner(new File("numbers.text")); PhoneNumber phoneNumNext, phoneNum = null; int counts = 0; String val = PhoneNumber.read(scan); while (val != null) { if (phoneNum == null) { phoneNum = new PhoneNumber(val); System.out.println(phoneNum + "\nis toll free: " + phoneNum.isFreeToll() + "\n"); counts++; } else { phoneNumNext = new PhoneNumber(val); if (phoneNum.equals(phoneNumNext)) { System.out.println("Duplicate phone number \"(" + phoneNumNext.getAreaCode() + ")" + phoneNumNext.getExchange() + "-" + phoneNumNext.getLineNumber() + "\" discovered"); } else { System.out.println(phoneNumNext + "\nis toll free: " + phoneNumNext.isFreeToll() + "\n"); } counts++; phoneNum = phoneNumNext; } val = PhoneNumber.read(scan); } System.out.println("---"); System.out.println(counts + " phone numbers processed."); } }
please use the name, phonenumber and phonebookentry to build this.
The three parallel arrays are gone — replaced with a single array of type PhonebookEntry.
You should be reading in the entries using the read method of your PhonebookEntry class (which in turn uses the read methods of the Name and PhoneNumber classes).
Use the equals methods of the Name and PhoneNumber classes in your lookup and reverseLookup methods.
Use the toString methods to print out information.
Make 100 the capacity of your Phonebook array
Throw an exception (of class Exception) if the capacity of the Phonebook array is exceeded.
Place a try/catch around your entire main and catch both FileNotFoundExceptions and Exceptions (remember, the order of appearance of the exception types in the catch blocks can make a difference).
Do not use
BufferedReader while(true)
breaks
The name of your application class should be Phonebook. Also, you should submit ALL your classes (i.e., Name, Strip off public from all your class definintions
name class:
import java.io.*;
import java.util.*;
public class Name {
public Name(String last, String first) {
this.last = last;
this.first = first;
}
public Name(String first) {
this("", first);
}
public boolean equals(Name same) {
return first.equals(same.first) && last .equals(same.last);
}
public String toString() {
return first + " " + last;
}
public String getFormal() {
return first + " " + last;
}
public String getOfficial() {
return last + ", " + first;
}
public static Name read(Scanner scan) {
if (!scan.hasNext())
return null;
String first = scan.next();
String last = scan.next();
return new Name(first, last);
}
public String getInitials() {
return Character.toUpperCase(first.charAt(0)) + "." + Character.toUpperCase(last.charAt(0))+".";
}
private String last, first;
public static void main(String [] args) throws Exception {
Scanner scan = new Scanner(new File("names.text"));
int count = 0;
Name readName = read(scan);
Name readLastName =null;
while(readName != null) {
if(readLastName!=null && readName!=null && readLastName.equals(readName)){
System.out.println("Duplicate name \""+readName+"\" discovered");
}
else{
System.out.println("name: " + readName);
System.out.println("formal: " + readName.getFormal());
System.out.println("official: " + readName.getOfficial());
System.out.println("initials: " + readName.getInitials());
System.out.println();
}
count++;
readLastName= readName;
readName = read(scan);
}
System.out.println("---");
System.out.println(count + " names processed.");
}
}
Phonenumber class:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class PhoneNumber {
private String num;
public PhoneNumber(String num) {
this.num = num;
}
public String getAreaCode() {
return num.substring(1, 4);
}
public String getExchange() {
return num.substring(5, 8);
}
public String getLineNumber() {
return num.substring(9);
}
public boolean isFreeToll() {
if (getAreaCode().charAt(0) == '8') {
return true;
}
return false;
}
public String toString() {
return "phone number: " + num + "\narea code: " + getAreaCode()
+ "\nexchange: " + getExchange() + "\nline number: "
+ getLineNumber();
}
public boolean equals(PhoneNumber phoneNum) {
if (phoneNum.getAreaCode().compareTo(this.getAreaCode()) == 0
&& phoneNum.getExchange().compareTo(this.getExchange()) == 0
&& phoneNum.getLineNumber().compareTo(this.getLineNumber()) == 0) {
return true;
}
return false;
}
public static String read(Scanner scan) {
if (scan.hasNextLine()) {
return scan.nextLine();
}
return null;
}
public static void main(String[] args) throws FileNotFoundException {
Scanner scan = new Scanner(new File("numbers.text"));
PhoneNumber phoneNumNext, phoneNum = null;
int counts = 0;
String val = PhoneNumber.read(scan);
while (val != null) {
if (phoneNum == null) {
phoneNum = new PhoneNumber(val);
System.out.println(phoneNum + "\nis toll free: "
+ phoneNum.isFreeToll() + "\n");
counts++;
} else {
phoneNumNext = new PhoneNumber(val);
if (phoneNum.equals(phoneNumNext)) {
System.out.println("Duplicate phone number \"("
+ phoneNumNext.getAreaCode() + ")"
+ phoneNumNext.getExchange() + "-"
+ phoneNumNext.getLineNumber() + "\" discovered");
} else {
System.out.println(phoneNumNext + "\nis toll free: "
+ phoneNumNext.isFreeToll() + "\n");
}
counts++;
phoneNum = phoneNumNext;
}
val = PhoneNumber.read(scan);
}
System.out.println("---");
System.out.println(counts + " phone numbers processed.");
}
}
For example, if the file phonebook.text contains:
Arnow David (123)456-7890
Harrow Keith (234)567-8901
Jones Jackie (345)678-9012
Augenstein Moshe (456)789-0123
Sokol Dina (567)890-1234
Tenenbaum Aaron (678)901-2345
Weiss Gerald (789)012-3456
Cox Jim (890)123-4567
Langsam Yedidyah (901)234-5678
Thurm Joseph (012)345-6789
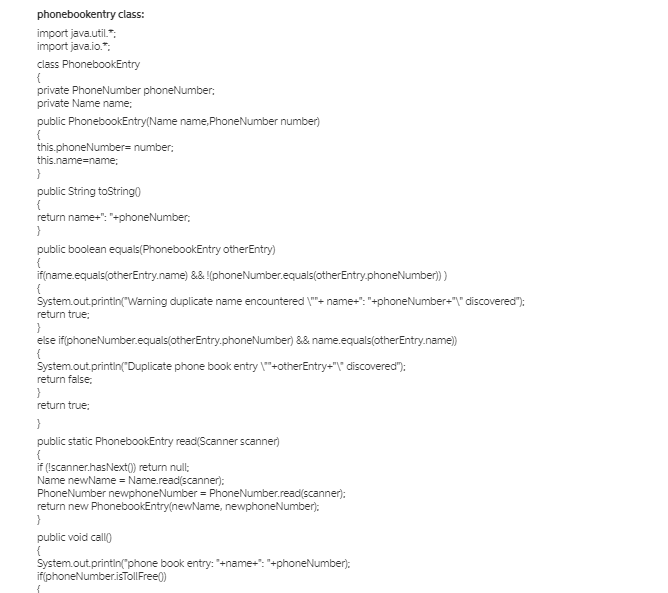
![System.out.printin("phone book entry: "+name+": "+phoneNumber):
if(phoneNumber.isTollFree()
{
System.out.printin("Dialing (toll-free) "+name+": "+phoneNumber):
System.out.printin):
else
{
System.out.printin("Dialing "+name+": "+phoneNumber):
System.out.printin("):
}
}
public static void main(String] args) throws Exception
{
String tempFirst="temp", templast="temp", tempNumber="temp":
PhoneNumber oldNumber= new PhoneNumber(tempNumber):
Name oldName= new Name(templast,tempFirst):
PhonebookEntry temp= new PhonebookEntry(oldName, oldNumber):
int count=0;
Scanner scanner = new Scanner(new File("phonebook.text")):
PhonebookEntry entry=read(scanner):
while(entry!=nul)
if(entry.equals(temp)
{
entry.call):
count++;
else
count++;
oldName=entry.name;
oldNumber=entry.phoneNumber:
temp= new PhonebookEntry(oldName,oldNumber):
entry=read(scanner):
}
System.out.printin("--):
System.out.printin(count+" phonebook entries processed."):
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffbb4ddf1-ce9d-407f-a13a-103abb2adbdc%2F9a363abc-64e1-4b03-a8c9-e192b1e5d16c%2Fhwzml7o_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

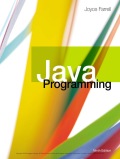
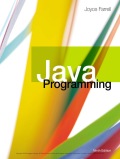