ivate boolean isEvenNumber; public CheckEvenOdd(Printer printer,int maxLimit,boolean isEvenNumber) { this.maxLimit = maxLimit; this.isEvenNumber = isEvenNumber; this.printer = printer; } @Override public void run() { int number = isEvenNumber ? 2 : 1; while (number <= maxLimit) { if (isEvenNumber) { printer.printEven(number); } else {
// the language is java
according to this code, do the next steps.
/class for check even or odd number
class CheckEvenOdd implements Runnable {
private int maxLimit;
private Printer printer;
private boolean isEvenNumber;
public CheckEvenOdd(Printer printer,int maxLimit,boolean isEvenNumber) {
this.maxLimit = maxLimit;
this.isEvenNumber = isEvenNumber;
this.printer = printer;
}
@Override
public void run() {
int number = isEvenNumber ? 2 : 1;
while (number <= maxLimit) {
if (isEvenNumber) {
printer.printEven(number);
} else {
printer.printOdd(number);
}
number += 2;
}
}
}
//Printer class for print the number with thread name
class Printer {
private volatile boolean isOdd;
synchronized void printEven(int number) {
while (!isOdd) {
try {
wait();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
System.out.println(Thread.currentThread().getName() + " thread :" + number);
isOdd = false;
notify();
}
synchronized void printOdd(int number) {
while (isOdd) {
try {
wait();
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
System.out.println(Thread.currentThread().getName() + " thread :" + number);
isOdd = true;
notify();
}
}
//Main class contains two threads.
public class Main{
public static void main(String[] args) {
Printer print = new Printer();
Thread t1 = new Thread(new CheckEvenOdd(print, 50, false),"Odd");
Thread t2 = new Thread(new CheckEvenOdd(print, 50, true),"Even");
t1.start();
t2.start();
}
}


public class PrintEvenOddTeaser {
class TaskEvenOdd implements Runnable {
private int max;
private printer print;
private boolean isEvenNumber;
TaskEvenOdd(Printer print, int max , boolean isEvenNumber) {
this.print = print;
this.max=max;
this.isEvenNumber = isEvenNumber;
}
@Override
public void run(){
//System.out.println("Run method");
int number = isEvenNumber==true ? 2:1;
while(number <=max){
if(is EvenNumber) {
//System.out.println("Even :"+Thread.currentThread().getName());
print.printEven(number);
//number+=2;
}
else {
//System.out.println("Odd:"+Thread.currentThread().getName());
print.printOdd(number);
//number+=2;
}
number +=2;
}
}
}
class Printer{
boolean isOdd=false;
synchronized void printEven(int number) {
while(isOdd==false) {
try {
wait();
}
catch(InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Even:"+number) {
isOdd==false;
notifyAll();
}
synchronized void printOdd(int number){
while(isOdd== true){
try {
wait();
}
catch(InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Odd:" + number);
isOdd=true;
notifyAll();
}
}
Step by step
Solved in 2 steps with 1 images

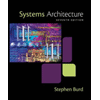
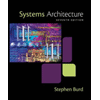