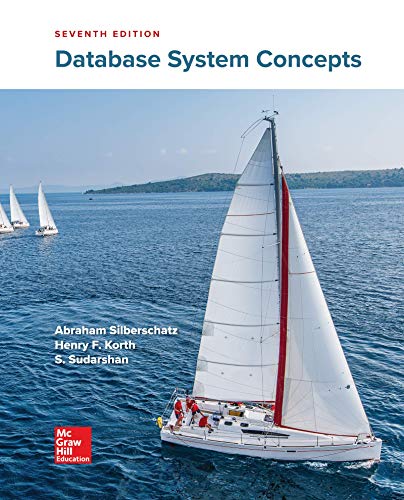
Intellij - Java
Write a program which does the following:
-
Create a class with the main() method.
-
Take a string input from the user in main() method.
-
Write another method called “countNumberOfConsonants” that gets a “String” value as a
method parameter.
-
In main() method, and pass the string entered by the user to this
“countNumberOfConsonants” method.
-
Write logic in “countNumberOfConsonants” to count the number of consonants in the
passed string value.
-
Return the count from “countNumberOfConsonants” method and print the result in main()
method.
Example:
Enter a string: Fairfield University
Number of consonants in “Fairfield University”: 11Note: In the output line, the user's entered string is displayed inside double quotes. Check Lecture-8 slides on escape sequence character on how to print output with double quotes.
Program 2:
Write a program which does the following:
-
Create a class with the main() method.
-
Take a string array input from the user in main() method. Note: Do not hardcode the string
array in code.
-
After the String array input loop, the array should be:
words[] = {"today", "is", "a", "lovely", "spring","day", "not", "too", "hot", "not", "too", "cold"};
-
Print the contents of this array using Arrays.toString() method.
-
Write a method called handleString() that performs the following:
-
Takes a String[] array as method parameter (similar to main method).
-
Loop through the array passed as parameter, and for each word in the array:
-
If the length of the word is even, get the index of the last letter & print that index. Use length() and indexOf() String object methods.
-
If the string length is odd, get the character at the 1st position in the string & print that letter. Use length() and charAt() String object methods.
-
-

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Write in Javaarrow_forwardusepropositional logic to prove the arguments valid (A ^ B) ^ (B -> A’) -> (C ^ B’)arrow_forwardjava Finish the method called profit() that will take two int arguments and returns a String saying if there is a profit, a loss, or no loss. The first argument is the buyPrice value and the second argument is the sellPrice value. There is a profit if the sell price is more than the buy price. This is a return method with two int parametersarrow_forward
- Javaarrow_forwardJava Jiffy labarrow_forward6. Design a Java class with a main method that does the following. You must include a meaningful comment for main and each method a. Main invokes a method readScores to read input from a file (name of your choice) that contains the name of the bowlers (no more than 25) and their bowling scores (two scores per bowler). The program stops reading values when it has reached the end of the file. For example, the file might contain: Bob 203 176 Jane 210 253 Jack 300 189 Eileen 220 298 • As the method reads the data, it stores the name into a String array and the average of the two bowling scores into an array of double. • The method returns the actual number of bowlers read in b. Main then invokes a method computeOverallAvg to: compute (a) the overall average score of all the bowlers combined, (b) the average score and name of the bowler with the highest average score and (c) the average score and name of the bowler with the lowest average scores. print to the console (a) the overall average…arrow_forward
- Here is the question: Create a program that contains a String that holds your favorite inspirational quote. Pass the quote to the calculateSpaces method and return the number of spaces to be displayed in the main method. Here is the code given: public class CountSpaces { public static void main(String[] args) { // write your code here } public static int calculateSpaces(String inString) { // write your code here } }arrow_forwardNeed help with 8,9, and 10 if possible. Java Codingarrow_forwardCould you help me with this C++ code please: Modify the class Song you created by doing thefollowing:(a) Add methods set_title(title), set_artist(artist) andset_year(year) that set the title, artist and recording year of thesong to the given values. The first two methods take a string as argument, the third one takes an integer.(b) Add a method set(title, artist, year) that sets the title,artist and recording year of the song to the given values. The firsttwo arguments are strings, the third one is an integer.(c) Replace the equals method by an equality operator (==).(d) Replace the less_than method by a less-than operator (<).(e) Replace the print method by an output operator (<<).(f) Replace the read method by an input operator (>>).(g) Organize your code into separate files as explained in Section 2.4 ofthe notes.Once again, you’ll need to decide how arguments should be passed to themethods and operators.Declare methods to be constant, as appropriate. Declare methods…arrow_forward
- public class utils { * Modify the method below. The method below, myMethod, will be called from a testing function in VPL. * write one line of Java code inside the method that adds one string * to another. It will look something like this: * Assume that 1. String theInput is 2. string mystring is ceorge неllo, пу nane is * we want to update mystring so that it is неllo, пу nane is Ceorge */ public static string mymethod(string theInput){ System.out.println("This method combines two strings."); Systen.out.println("we combined two strings to make mystring); return mystring;arrow_forwardIn python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forward!! E! 4 2 You are in process of writing a class definition for the class Book. It has three data attributes: book title, book author, and book publisher. The data attributes should be private. In Python, write an initializer method that will be part of your class definition. The attributes will be initialized with parameters that are passed to the method from the main program. Note: You do not need to write the entire class definition, only the initializer method lili lilıarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
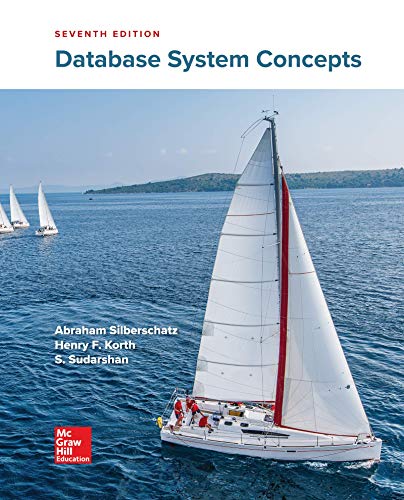
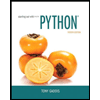
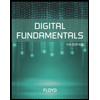
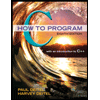
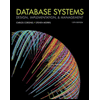
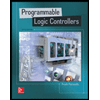