iven code is provided down below. Please do not change the existing code. The instructions are in the image. Please explain and show each step and a screenshot of the input and output. Thank you. main.cpp #include "Triangle.h" #include "Square.h" #include using namespace std; int main() { //Do not modify
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Given code is provided down below. Please do not change the existing code. The instructions are in the image. Please explain and show each step and a screenshot of the input and output. Thank you.
main.cpp
#include "Triangle.h"
#include "Square.h"
#include <iostream>
using namespace std;
int main() {
//Do not modify
return 0;
}
-------------------------------------------
Square.cpp
#include "Square.h"
#include <iostream>
using namespace std;
Square::Square(){
base = 0;
height = 0;
}
Square::Square(int b, int h) : base(b), height(h) { }
void Square::setBase(int b){
base = b;
}
void Square::setHeight(int h){
height = h;
}
void Square::calculateArea(){
// calculate Area correctly depending on shape type
}
int Square::getBase() const{
return base;
}
int Square::getHeight() const{
return height;
}
double Square::getArea() const{
return area;
}
// Overloaded << operator
// Overloaded >> operator
-------------------------------------------
Square.h
#ifndef SQUARE_H_
#define SQUARE_H_
#include <iostream>
using namespace std;
class Square {
public:
Square();
Square(int b, int h);
//Setters
void setBase(int b);
void setHeight(int h);
//Getters
int getBase() const;
int getHeight() const;
double getArea() const;
void calculateArea();
// Overloaded << operator declaration
// Overloaded >> operator declaration
private:
int base;
int height;
double area;
};
#endif
-------------------------------------------
Triangle.cpp
#include "Triangle.h"
#include <iostream>
using namespace std;
Triangle::Triangle(){
base = 0;
height = 0;
}
Triangle::Triangle(int b, int h) : base(b), height(h) { }
void Triangle::setBase(int b){
base = b;
}
void Triangle::setHeight(int h){
height = h;
}
void Triangle::calculateArea(){
// calculate Area correctly depending on shape type
}
int Triangle::getBase() const{
return base;
}
int Triangle::getHeight() const{
return height;
}
double Triangle::getArea() const{
return area;
}
// Overloaded << operator declaration
// Overloaded >> operator declaration
-------------------------------------------
Triangle.h
#ifndef TRIANGLE_H_
#define TRIANGLE_H_
#include <iostream>
using namespace std;
class Triangle {
public:
Triangle();
Triangle(int b, int h);
//Setters
void setBase(int b);
void setHeight(int h);
//Getters
int getBase() const;
int getHeight() const;
double getArea() const;
void calculateArea();
// Overloaded << operator declaration
// Overloaded >> operator declaration
private:
int base;
int height;
double area;
};
#endif
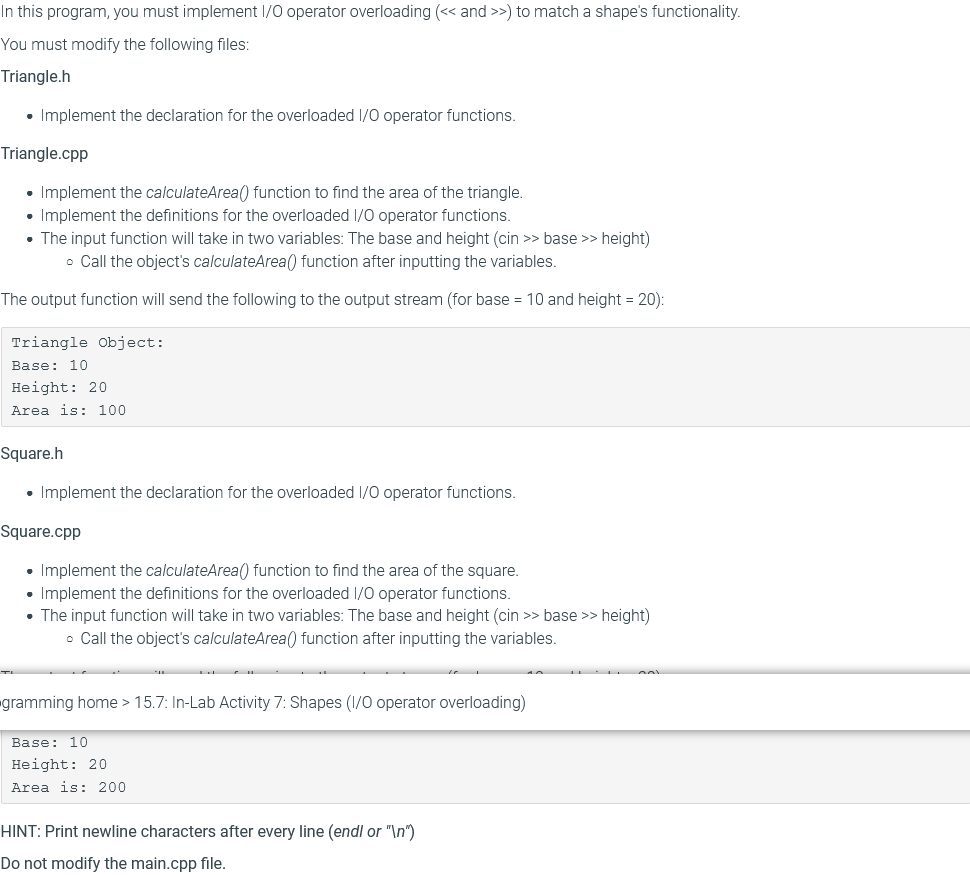

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 1 images

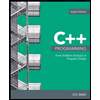
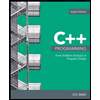