Java Program ASAP Please modify Map morseCodeMap = readMorseCodeTable("morse.txt"); in the program so it reads the two text files and passes the test cases. Down below is a working code. Also dont add any import libraries in the program just modify the rest of the code so it passes the test cases. import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.HashMap; import java.util.Map; import java.util.Scanner; public class MorseCodeConverter { public static void main(String[] args) { Map morseCodeMap = readMorseCodeTable("morse.txt"); Scanner scanner = new Scanner(System.in); System.out.print("Please enter the file name or type QUIT to exit:\n"); do { String fileName = scanner.nextLine().trim(); if (fileName.equalsIgnoreCase("QUIT")) { break; } try { String text = convertMorseCodeToText(fileName, morseCodeMap); System.out.println(text); } catch (IOException e) { System.out.println("File '" + fileName + "' is not found."); System.out.print("Please re-enter the file name or type QUIT to exit:\n"); } } while (true); scanner.close(); } private static Map readMorseCodeTable(String fileName) { Map morseCodeMap = new HashMap<>(); try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) { String line; while ((line = reader.readLine()) != null) { String[] parts = line.split("\\s+"); if (parts.length == 2) { morseCodeMap.put(parts[1], parts[0]); } } } catch (IOException e) { e.printStackTrace(); } return morseCodeMap; } private static String convertMorseCodeToText(String fileName, Map morseCodeMap) throws IOException { StringBuilder result = new StringBuilder(); try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) { String line; while ((line = reader.readLine()) != null) { String[] morseWords = line.split("[.!?]"); for (String morseWord : morseWords) { String[] morseLetters = morseWord.trim().split("\\s+"); for (String morseLetter : morseLetters) { if (morseCodeMap.containsKey(morseLetter)) { result.append(morseCodeMap.get(morseLetter)); } } result.append(" "); // Add a space between words } result.append(System.lineSeparator()); // Add a newline between sentences } } return result.toString().trim(); } } text1.txt StopAndSmellTheRoses. text2.txt ATrueRebelYouAre!EveryoneWasImpressed.You'llDoWellToContinueInTheSameSpirit. PleaseExplainABitMoreInTheWayOfFootnotes.FromTheGivenTextIt'sNotClearWhatAreWeReadingAbout. Test Case 1 Please enter the file name or type QUIT to exit:\n text1.txtENTER Stop and smell the roses.\n Test Case 2 Please enter the file name or type QUIT to exit:\n txt1.txtENTER File 'txt1.txt' is not found.\n Please re-enter the file name or type QUIT to exit:\n text1.txtENTER Stop and smell the roses.\n Test Case 3 Please enter the file name or type QUIT to exit:\n text2.txtENTER A true rebel you are! Everyone was impressed. You'll do well to continue in the same spirit.\n Please explain a bit more in the way of footnotes. From the given text it's not clear what are we reading about.\n Test Case 4 Please enter the file name or type QUIT to exit:\n somefile.txtENTER File 'somefile.txt' is not found.\n Please re-enter the file name or type QUIT to exit:\n anotherbadfile.txtENTER File 'anotherbadfile.txt' is not found.\n Please re-enter the file name or type QUIT to exit:\n quitENTER
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class MorseCodeConverter {
public static void main(String[] args) {
Map<String, String> morseCodeMap = readMorseCodeTable("morse.txt");
Scanner scanner = new Scanner(System.in);
System.out.print("Please enter the file name or type QUIT to exit:\n");
do {
String fileName = scanner.nextLine().trim();
if (fileName.equalsIgnoreCase("QUIT")) {
break;
}
try {
String text = convertMorseCodeToText(fileName, morseCodeMap);
System.out.println(text);
} catch (IOException e) {
System.out.println("File '" + fileName + "' is not found.");
System.out.print("Please re-enter the file name or type QUIT to exit:\n");
}
} while (true);
scanner.close();
}
private static Map<String, String> readMorseCodeTable(String fileName) {
Map<String, String> morseCodeMap = new HashMap<>();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split("\\s+");
if (parts.length == 2) {
morseCodeMap.put(parts[1], parts[0]);
}
}
} catch (IOException e) {
e.printStackTrace();
}
return morseCodeMap;
}
private static String convertMorseCodeToText(String fileName, Map<String, String> morseCodeMap) throws IOException {
StringBuilder result = new StringBuilder();
try (BufferedReader reader = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = reader.readLine()) != null) {
String[] morseWords = line.split("[.!?]");
for (String morseWord : morseWords) {
String[] morseLetters = morseWord.trim().split("\\s+");
for (String morseLetter : morseLetters) {
if (morseCodeMap.containsKey(morseLetter)) {
result.append(morseCodeMap.get(morseLetter));
}
}
result.append(" "); // Add a space between words
}
result.append(System.lineSeparator()); // Add a newline between sentences
}
}
return result.toString().trim();
}
}
text1.txt
PleaseExplainABitMoreInTheWayOfFootnotes.FromTheGivenTextIt'sNotClearWhatAreWeReadingAbout.
Test Case 1
text1.txtENTER
Stop and smell the roses.\n
Test Case 2
txt1.txtENTER
File 'txt1.txt' is not found.\n
Please re-enter the file name or type QUIT to exit:\n
text1.txtENTER
Stop and smell the roses.\n
Test Case 3
text2.txtENTER
A true rebel you are! Everyone was impressed. You'll do well to continue in the same spirit.\n
Please explain a bit more in the way of footnotes. From the given text it's not clear what are we reading about.\n
Test Case 4
somefile.txtENTER
File 'somefile.txt' is not found.\n
Please re-enter the file name or type QUIT to exit:\n
anotherbadfile.txtENTER
File 'anotherbadfile.txt' is not found.\n
Please re-enter the file name or type QUIT to exit:\n
quitENTER

Step by step
Solved in 3 steps with 1 images

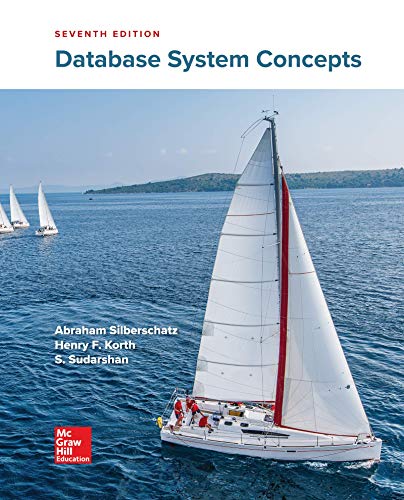
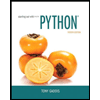
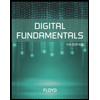
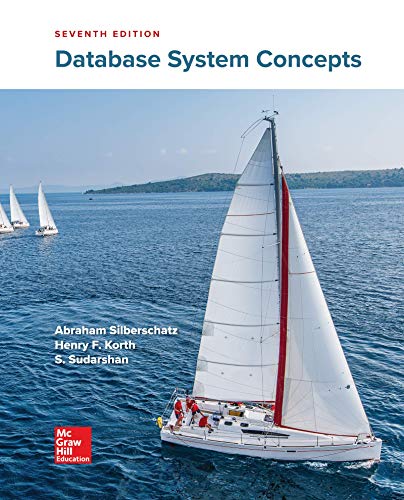
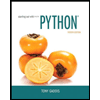
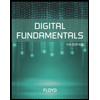
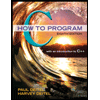
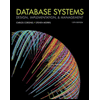
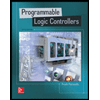