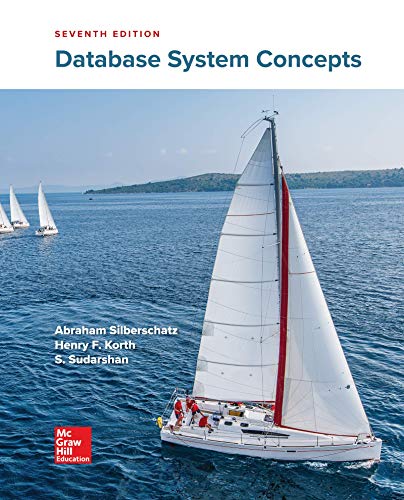
import java.io.*;
import java.util.*;
public class Main{
public static void main(String[] args) {
List<String> girlsNames = loadNames("Girlnames.txt");
List<String> boysNames = loadNames("Boynames.txt");
if (girlsNames == null && boysNames == null) {
System.out.println("Both files are missing. Exiting...");
return;
} else if (girlsNames == null) {
System.out.println("Girlnames.txt is missing. Exiting...");
return;
} else if (boysNames == null) {
System.out.println("Boynames.txt is missing. Exiting...");
return;
}
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Enter a name (or 'QUIT' to exit): ");
String input = scanner.nextLine();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
searchAndDisplay(input, girlsNames, boysNames);
}
scanner.close();
}
private static List<String> loadNames(String filename) {
List<String> names = new ArrayList<>();
try (BufferedReader reader = new BufferedReader(new FileReader(filename))) {
String line;
while ((line = reader.readLine()) != null) {
names.add(line.trim());
}
} catch (IOException e) {
return null;
}
return names;
}
private static void searchAndDisplay(String name, List<String> girlsNames, List<String> boysNames) {
int girlIndex = searchName(name, girlsNames);
int boyIndex = searchName(name, boysNames);
String capitalizedName = name.substring(0, 1).toUpperCase() + name.substring(1).toLowerCase();
if (girlIndex == -1 && boyIndex == -1) {
System.out.println("The name '" + capitalizedName + "' was not found in either list.");
} else if (girlIndex != -1 && boyIndex == -1) {
System.out.println("The name '" + capitalizedName + "' was found in popular girl names list (line " + (girlIndex + 1) + ").");
} else if (girlIndex == -1 && boyIndex != -1) {
System.out.println("The name '" + capitalizedName + "' was found in popular boy names list (line " + (boyIndex + 1) + ").");
} else {
System.out.println("The name '" + capitalizedName + "' was found in both lists: boy names (line " + (boyIndex + 1) + ") and girl names (line " + (girlIndex + 1) + ").");
}
}
private static int searchName(String name, List<String> namesList) {
for (int i = 0; i < namesList.size(); i++) {
if (namesList.get(i).equalsIgnoreCase(name)) {
return i;
}
}
return -1;
}
}
if (girlsNames == null && boysNames == null) {
System.out.println("Both files are missing. Exiting...");
return;
} else if (girlsNames == null) {
System.out.println("Girlnames.txt is missing. Exiting...");
return;
} else if (boysNames == null) {
System.out.println("Boynames.txt is missing. Exiting...");
return;
}
THE TEXT FILES ARE LOCATED IN HYPERGRADE I provided them and the failed test case as a screenshot. Thank you.
Test Case 1
Enter a name to search or type QUIT to exit:\n
AnnabelleENTER
The name 'Annabelle' was not found in either list.\n
Enter a name to search or type QUIT to exit:\n
xavierENTER
The name 'Xavier' was found in popular boy names list (line 81).\n
Enter a name to search or type QUIT to exit:\n
AMANDAENTER
The name 'Amanda' was found in popular girl names list (line 63).\n
Enter a name to search or type QUIT to exit:\n
jOrdAnENTER
The name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\n
Enter a name to search or type QUIT to exit:\n
quitENTER
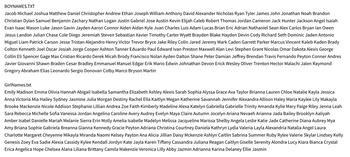
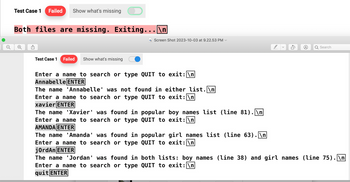

Step by stepSolved in 4 steps with 1 images

- Redesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardimport java.util.Scanner; public class Inventory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); InventoryNode headNode; InventoryNode currNode; InventoryNode lastNode; String item; int numberOfItems; int i; // Front of nodes list headNode = new InventoryNode(); lastNode = headNode; int input = scnr.nextInt(); for(i = 0; i < input; i++ ) { item = scnr.next(); numberOfItems = scnr.nextInt(); currNode = new InventoryNode(item, numberOfItems); currNode.insertAtFront(headNode, currNode); lastNode = currNode; } // Print linked list currNode = headNode.getNext(); while (currNode != null) { currNode.printNodeData(); currNode…arrow_forwardpreLabC.java 1 import java.util.Random; 2 import java.util.StringJoiner; 3 4- public class preLabC { 5 6- 7 8 9 10 11 12 13 14 15 16 17 18 19 20 } public static String myMethod(MathVector inputVector) { String vectorStringValue = new String(); // empty String object System.out.println("here is the contents object, inputVector: + inputVector); // get a String out of that MathVector object. Do it here. On the next line. // return the double value here. return vectorStringValue; "1 ▸ Compilation Description A MathVector object will be passed to your method. Return its contents as a String. If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector object as a String. This makes it useful for displaying to the user. /** * Returns a String representation of this vector. The String should be in the format "[1, 2, 3]" * * @return a String representation of this vector * @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method. */…arrow_forward
- Given the following class declaration: class List { public: List(); List(const List&); List& operator=(const List&); -List(); // Inserts element in the specified position, returns true if // it was able to insert. // Example, if the list is [4, 2, 1], and the user // calls list.Insert(6, 1) the list would become [4, 6, 2, 1] bool Insert(double element, size_t position) ; // Returns the position of element if found, if not found returns -1 int Index0f(double element)const ; // Removes the element in the given position double Remove(size_t position) ; // Gets the number in the given position double Get(size_t position)const ; // Returns a string representation of the list, elements // separated by commas and the list between brackets string ToString()const ; // Returns the number of elements in the list size_t Size)const; // Returns true if the list is empty, false otherwise bool IsEmpty)const; // Clears the whole list void Clear(); private: struct Node{ double number; Node* next; };…arrow_forwardIN JAVA PLEASE, need help finding an element in the list /** * Returns whether the given value exists in the list. * @param value - value to be searched. * @return true if specified value is present in the list, false otherwise. */ public int indexOf(int value) { //Implement this method return -1; }arrow_forwardStarter code for ShoppingList.java import java.util.*;import java.util.LinkedList; public class ShoppingList{ public static void main(String[] args) { Scanner scnr=new Scanner(System.in); LinkedList<ListItem>shoppingList=new LinkedList<ListItem>();//declare LinkedList String item; int i=0,n=0;//declare variables item=scnr.nextLine();//get input from user while(item.equals("-1")!=true)//get inputuntil user not enter -1 { shoppingList.add(new ListItem(item));//add into shoppingList LinkedList n++;//increment n item=scnr.nextLine();//get item from user } for(i=0;i<n;i++) { shoppingList.get(i).printNodeData();//call printNodeData()for each object } }} class ListItem{ String item; //constructor ListItem(String item) { this.item=item; } void printNodeData() { System.out.println(item); }}arrow_forward
- Run the program to tell me there is an error, BSTChecker.java:3: error: duplicate class: Node class Node { ^ BSTChecker.java:63: error: class LabProgram is public, should be declared in a file named LabProgram.java public class LabProgram { ^ 2 errors This is my program, please fix it. import java.util.*; class Node { int key; Node left, right; public Node(int key) { this.key = key; left = right = null; } } class BSTChecker { public static Node checkBSTValidity(Node root) { return checkBSTValidityHelper(root, null, null); } private static Node checkBSTValidityHelper(Node node, Integer min, Integer max) { if (node == null) { return null; } if ((min != null && node.key <= min) || (max != null && node.key >= max)) { return node; } Node leftResult = checkBSTValidityHelper(node.left, min, node.key); if (leftResult != null) { return leftResult; } Node rightResult = checkBSTValidityHelper(node.right, node.key, max); if (rightResult != null) { return rightResult; }…arrow_forwardimport java.util.Scanner; public class Playlist { // TODO: Write method to ouptut list of songs public static void main (String[] args) { Scanner scnr = new Scanner(System.in); SongNode headNode; SongNode currNode; SongNode lastNode; String songTitle; int songLength; String songArtist; // Front of nodes list headNode = new SongNode(); lastNode = headNode; // Read user input until -1 entered songTitle = scnr.nextLine(); while (!songTitle.equals("-1")) { songLength = scnr.nextInt(); scnr.nextLine(); songArtist = scnr.nextLine(); currNode = new SongNode(songTitle, songLength, songArtist); lastNode.insertAfter(currNode); lastNode = currNode; songTitle = scnr.nextLine(); } // Print linked list…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
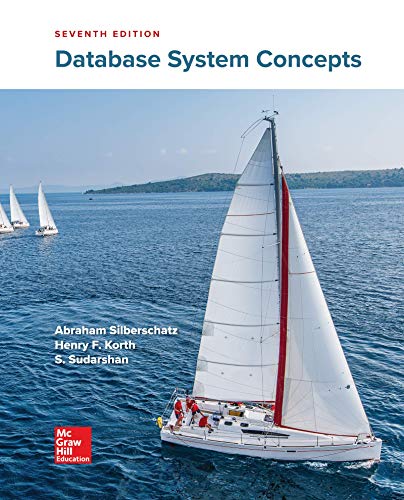
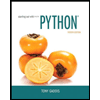
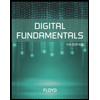
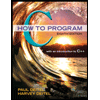
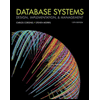
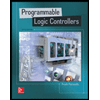