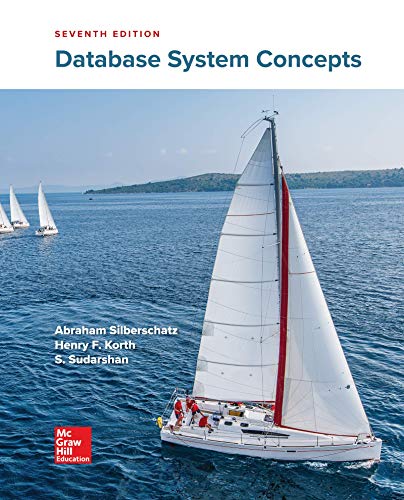
JAVA PROGRAM
PLEASE MODIFY THIS PROGRAM SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES THE TEST CASE and take out the following from the program please: Boynames.txt is missing.\n Girlnames.txt is missing.\n Both data files are missing.\n THE TEST CASE DOES NOT ASK FOR IT. PLEASE REMOVE IT. I HAVE PROVIDED THE TEST CASE THAT FAILED AND THE INPUTS AS A SCREENSHOT.
import java.io.*;
import java.util.*;
public class NameSearcher {
private static List<String> loadFileToList(String filename) throws FileNotFoundException {
List<String> namesList = new ArrayList<>();
File file = new File(filename);
if (!file.exists()) {
throw new FileNotFoundException(filename);
}
try (Scanner scanner = new Scanner(file)) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine().trim();
String[] names = line.split("\s+");
for (String name : names) {
namesList.add(name.toLowerCase());
}
}
}
return namesList;
}
private static Integer searchNameInList(String name, List<String> namesList) {
int index = namesList.indexOf(name.toLowerCase());
return index == -1 ? null : index + 1;
}
public static void main(String[] args) {
List<String> boyNames = new ArrayList<>(); // Initialize with an empty list
List<String> girlNames = new ArrayList<>(); // Initialize with an empty list
boolean boyFileExists = false;
boolean girlFileExists = false;
try {
boyNames = loadFileToList("Boynames.txt");
boyFileExists = true;
} catch (FileNotFoundException e) {
// No need to display the message about missing file
}
try {
girlNames = loadFileToList("Girlnames.txt");
girlFileExists = true;
} catch (FileNotFoundException e) {
// No need to display the message about missing file
}
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("Enter a name to search or type QUIT to exit: ");
String input = scanner.nextLine().trim();
if (input.equalsIgnoreCase("QUIT")) {
break;
}
String capitalizedInput = input.substring(0, 1).toUpperCase() + input.substring(1).toLowerCase();
Integer boyIndex = searchNameInList(input, boyNames);
Integer girlIndex = searchNameInList(input, girlNames);
if (boyIndex == null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was not found in either list.");
} else if (boyIndex != null && girlIndex == null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular boy names list (line " + boyIndex + ").");
} else if (boyIndex == null && girlIndex != null) {
System.out.println("The name '" + capitalizedInput + "' was found in popular girl names list (line " + girlIndex + ").");
} else {
System.out.println("The name '" + capitalizedInput + "' was found in both lists: boy names (line " + boyIndex + ") and girl names (line " + girlIndex + ").");
}
}
scanner.close();
}
}
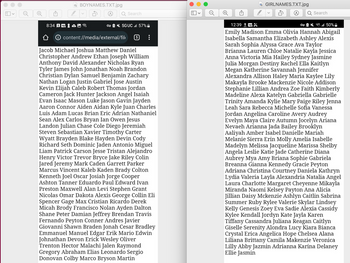
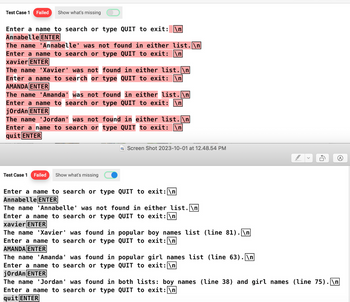

Step by stepSolved in 3 steps with 2 images

- JAVA PROGRAM ASAP The program does not run in hypergrade can you please MODIFY THIS program ASAP BECAUSE it does not pass all the test cases when I upload it to hypergrade. I have provided the correct test cases as a screenshot as well as the failed test cases. It says 0 out of 4 passed. The program must pass the test case when uploaded to Hypergrade. Files data are down below: text1.txt StopAndSmellTheRoses. text2.txt ATrueRebelYouAre!EveryoneWasImpressed.You'llDoWellToContinueInTheSameSpirit.PleaseExplainABitMoreInTheWayOfFootnotes.FromTheGivenTextIt'sNotClearWhatAreWeReadingAbout. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.Scanner;class Main { // Driver code public static void main(String[] args) { // scanner object used to take user input Scanner sc = new Scanner(System.in); // loop iterates until user enters "quit" or "QUIT" while (true) { System.out.print("Please enter…arrow_forwardJava Program ASAP ************This program must work in hypergrade and pass all the test cases.********** Please give me a new program becausse when I upload the program to hypergrade it does not pass the test cases. It says 1 out of 4 passed. Thank you! For Test Case 1 first print out Please enter the file name or type QUIT to exit:\ then you type text1.txtENTER and it displays Stop And Smell The Roses./n there needs to be nothing after that. For test case 2 first print out Please enter the file name or type QUIT to exit: then you type txt1.txtENTER then it reads out File 'txt1.txt' is not found.\n Then it didplays Please re-enter the file name or type QUIT to exit:\n after the test file is not found. then you type in text1.txt and it displays stop and smell the roses.\n. For test case 3 first print out Please enter the file name or type QUIT to exit: then you type text2.txtENTER and it displays A true rebel you are! Everyone was impressed. You'll do well to continue in…arrow_forwardJAVA PROGRAM PLEASE PLEASE PLEASE MODIFY THIS PROGRAM SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES THE TEST BECAUSE IT DOES NOT RUN IN HYPERGRADE. THEN TAKE OUT THE FOLLOWING FROM THE PROGRAM System.err.println("Error: " + filename + " is missing."); System.err.println("Error: Boynames.txt is missing."); and System.err.println("Error: Girlnames.txt is missing."); ALSO MAKE SURE IT PRINTS OUT THE FOLLOWING TEST CASE: Enter a name to search or type QUIT to exit:\nAnnabelleENTERThe name 'Annabelle' was not found in either list.\nEnter a name to search or type QUIT to exit:\nxavierENTERThe name 'Xavier' was found in popular boy names list (line 81).\nEnter a name to search or type QUIT to exit:\nAMANDAENTERThe name 'Amanda' was found in popular girl names list (line 63).\nEnter a name to search or type QUIT to exit:\njOrdAnENTERThe name 'Jordan' was found in both lists: boy names (line 38) and girl names (line 75).\nEnter a name to search or type QUIT to exit:\nquitENTER IT HAS TO SAY…arrow_forward
- Assume you are considering writing a method and are deciding what should happen when given input that is outside of perfect input. Which of the following is not a way to handle this? Group of answer choices 1. Use better JUnit testing 2. Throw an exception 3. Try to reasonably auto-correct 4. Prevent the errorarrow_forwardThe files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. You also will use a file named DebugEmployeeIDException.java with the DebugTwelve4.java file. // An employee ID can't be more than 999 // Keep executing until user enters four valid employee IDs // This program throws a FixDebugEmployeeIDException import java.util.*; public class DebugTwelve4 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String inStr, outString = ""; final int MAX = 999; int[] emps = new int[4]; for(int x = 0; x < emps.length; ++x) { System.out.println("Enter employee ID number"); inStr = input.next(); try { emps[x] = Integer.parseInt(inStr); if(emps[x] > MAX) { throw(new…arrow_forwardLab Tasks – complete these Use the information provided here, in the slideshow and in chapter 12 of our text to do the following exercise. Step 1: On the desktop or wherever you will be saving your java program, use notepador other basic text editor (NOT WORD) to create a .txt file. In this file, type out a list of 10 integers (one on each line or with a space between each). Save. Do this manually. Not with Java. Step 2. Now write a program with the following: 1) In the program creating a File object that connects to the .txt file that you just created. 2) Add code that will check if the file exists and if it doesn’t, it will create it. (Yes, we know it definitely exists but do this part anyway.) 3) Set up a Scanner so that you can read the values from the file and print them to the screen using a loop. You will probably need to use the .hasNext() method. Also, you may want to set up a counter to keep track of how many values there are for future use. (Note that the .length()…arrow_forward
- Java Proram ASAP Please improve and adjust the program which is down below with the futher moddifications because it does not pass the test cases in Hypergrade. Please remove /n from the program and for test case 4 after this line: Please re-enter the file name or type QUIT to exit:\n quitENTER there needs to be nothing. import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.compareTo("QUIT") == 0) { break; } else { String filePath = new File("").getAbsolutePath(); filePath = filePath.concat("/"); filePath = filePath.concat(input); File file = new File(filePath); if (file.exists() && !file.isDirectory()) {…arrow_forwardJava Program ASAP ************This program must work in hypergrade and pass all the test cases.********** The text files are located in Hypergrade. The program down below does not work in Hypergrade and does not pass the test cases. Please modify it or create a new program so when I upload to hypergrade it passes the test cases. Thank you! For Test Case 1 first print out Please enter the file name or type QUIT to exit:\ then you type text1.txtENTER and it displays Stop And Smell The Roses./n there needs to be nothing after that. For test case 2 first print out Please enter the file name or type QUIT to exit: then you type txt1.txtENTER then it reads out File 'txt1.txt' is not found.\n Then it didplays Please re-enter the file name or type QUIT to exit:\n after the test file is not found. then you type in text1.txt and it displays stop and smell the roses.\n. For test case 3 first print out Please enter the file name or type QUIT to exit: then you type text2.txtENTER and it…arrow_forwardYou guys giving me AI answer. Don't post AI generated answer or plagiarised answer. If I see these things I'll give you multiple downvotes and will report immediately.arrow_forward
- JAVA PROGRAM Please Modify this program with further modifications as listed below: The program must pass the test case when uploaded to Hypergrade. ALSO, change the following in the program: System.out.print("Enter a name (or 'QUIT' to exit): "); to: Enter a name to search or type QUIT to exit and take out this: System.out.println(filename + " is missing. Exiting..."); import java.io.*;import java.util.*;public class Main { public static void main(String[] args) { List<String> girlsNames = loadNames("GirlNames.txt"); List<String> boysNames = loadNames("BoyNames.txt"); Scanner scanner = new Scanner(System.in); while (true) { System.out.print("Enter a name (or 'QUIT' to exit): "); String input = scanner.nextLine(); if (input.equalsIgnoreCase("QUIT")) { break; } searchAndDisplay(input, girlsNames, boysNames); } scanner.close(); } private static…arrow_forwardJava Proram ASAP There is an extra /n in the program in test case 1-3 and after Please re-enter the file name or type QUIT to exit:\n quitENTER in test case 4 there needs to be nothing as shown in the screenshot. The text files are located in Hypergrade. Please modify this code below so it passes the test cases. Also I have the correct test case as a screenshot. import java.io.*;import java.util.Scanner;public class ConvertText { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.compareTo("QUIT") == 0) { break; } else { String filePath = new File("").getAbsolutePath(); filePath = filePath.concat("/"); filePath = filePath.concat(input); File file = new File(filePath); if…arrow_forwardConsider the statementScanner a = new Scanner(System.in); Here Scanner is the class name, a is the name of object, new keyword is used to allocate the memory and System.in is the input stream.Following methods of Scanner classare used in the program below :-1) nextInt to input an integer2) nextFloat to input a float3) nextLine to input a stringJava programming source codearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
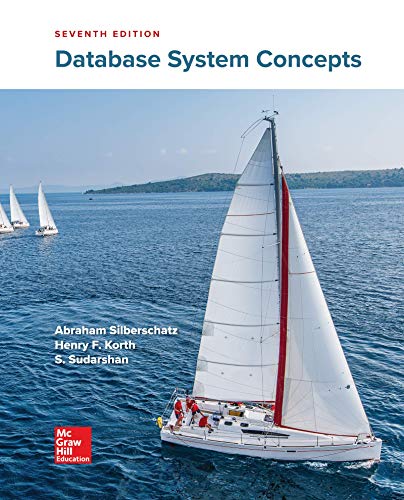
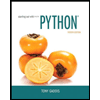
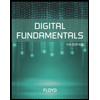
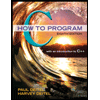
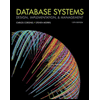
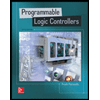