JAVA PROGRAM Create a class EmployeeImp and do the following implement interface EmployeeInterface Define the following attributes: int count and an array variable of type Employee. Write a default constructor and initialize array Employee with the size defined in EmployeeInterface Write a constructor that accepts size of an array as parameter. Create array Employee with the parameter size.
JAVA PROGRAM Create a class EmployeeImp and do the following implement interface EmployeeInterface Define the following attributes: int count and an array variable of type Employee. Write a default constructor and initialize array Employee with the size defined in EmployeeInterface Write a constructor that accepts size of an array as parameter. Create array Employee with the parameter size.
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter11: More Object-oriented Programming Concepts
Section: Chapter Questions
Problem 8PE
Related questions
Question
JAVA PROGRAM
Create a class EmployeeImp and do the following
- implement interface EmployeeInterface
- Define the following attributes: int count and an array variable of type Employee.
- Write a default constructor and initialize array Employee with the size defined in EmployeeInterface
- Write a constructor that accepts size of an array as parameter. Create array Employee with the parameter size.
Use the attached photo of diagram below as reference for the data members of each class.
Note: No need to define interface EmployeeInterface, Employee, HourlyEmployee and CommisionEmployee
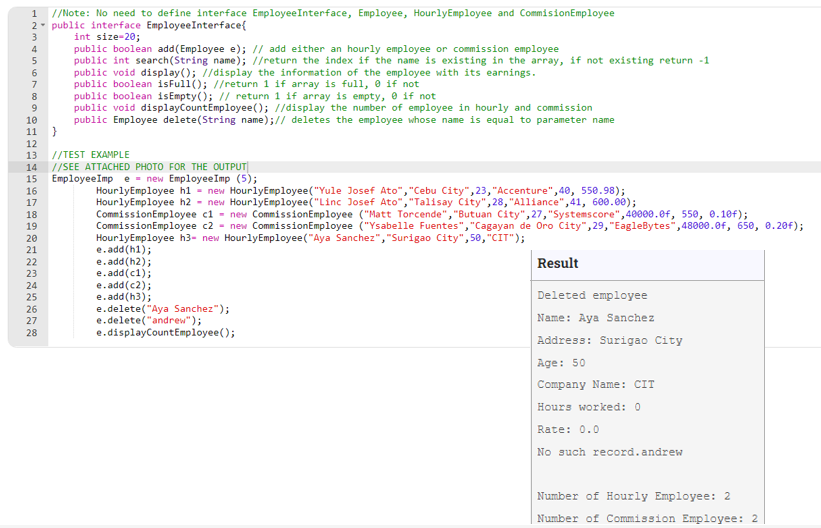
Transcribed Image Text:1
//Note: No need to define interface EmployeeInterface, Employee, HourlyEmployee and CommisionEmployee
2- public interface EmployeeInterface{
int size-20;
public boolean add(Employee e); // add either an hourly employee or commission employee
public int search(String name); //return the index if the name is existing in the array, if not existing return -1
public void display(); 7/display the information of the employee with its earnings.
public boolean isFull(); //return 1 if array is full, 0 if not
public boolean isEmpty(); // return 1 if array is empty, e if not
public void displayCountEmployee(); //display the number of employee in hourly and commission
public Employee delete(String name);// deletes the employee whose name is equal to parameter name
7
8
10
11
12
//TEST EXAMPLE
//SEE ATTACHED PHOTO FOR THE OUTPUT
15
13
14
EmployeeImp е - пеw EmployeеImp (5);
16
HourlyEmployee hl - new HourlyEmployee("Yule Josef Ato","Cebu City",23, "Accenture",40, 550.98);
HourlyEmployee h2 - new HourlyEmployee("Linc Josef Ato","Talisay City", 28, "Alliance",41, 600.00);
CommissionEmployee cl - new CommissionEmployee ("Matt Torcende", "Butuan City",27,"Systemscore",40000.ef, 550, 0.10f);
CommissionEmployee c2 - new CommissionEmployee ("Ysabelle Fuentes", "Cagayan de Oro City", 29, "EagleBytes",48000.0f, 650, 0.20f);
HourlyEmployee h3- new HourlyEmployee("Aya Sanchez", "Surigao City", 50, "CIT");
e.add(h1);
e.add(h2);
e.add(c1);
e.add(c2);
e.add(h3);
e.delete("Aya Sanchez");
e.delete("andrew");
e.displayCountEmployee();
17
18
19
20
21
22
Result
23
24
Deleted employee
25
26
27
Name: Aya Sanchez
28
Address: Surigao City
Age: 50
Company Name: CIT
Hours worked: 0
Rate: 0.0
No such record.andrew
Number of Hourly Employee: 2
Number of Commission Employee: 2
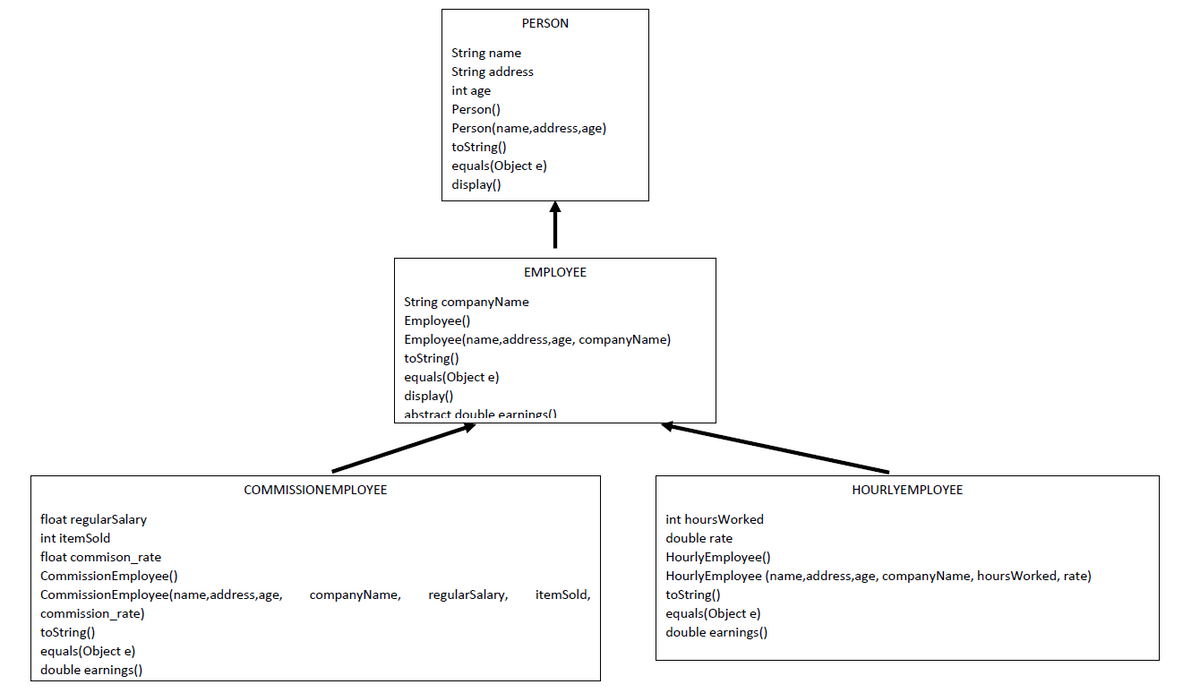
Transcribed Image Text:PERSON
String name
String address
int age
Person()
Person(name,address,age)
toString()
equals(Object e)
display()
EMPLOYEE
String companyName
Employee()
Employee(name,address,age, companyName)
toString()
equals(Object e)
display()
abs
act double earnings()
COMMISSIONEMPLOYEE
HOURLYEMPLOYEE
float regularSalary
int itemSold
int hoursWorked
double rate
float commison_rate
HourlyEmployee()
CommissionEmployee()
HourlyEmployee (name,address,age, companyName, hoursWorked, rate)
toString()
equals(Object e)
double earnings()
regularSalary,
CommissionEmployee(name,address,age,
commission_rate)
toString()
companyName,
itemSold,
equals(Object e)
double earnings()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage