Java programing Ships Study the UML diagram carefully. The green spots signify public. The red squares signify private. Superclass Ship is abstract because it contains the abstract method purpose. This class also implements the Comparable interface to compare ships by tonnage. Ship also has a public static integer named numShips that is incremented in the constructor to serve as a counter for the number of ships. After coding these classes, write a program named TestShips that uses them as follows: ⦁ Create an array of declared type Ship that contains at least six ships (two of each concrete subclass). You can make up all of the values for the data members of each ship. ⦁ The Strings returned by purpose() can be determined by viewing the Possible Output below. ⦁ Process the array in an enhanced for loop to display for each ship: ⦁ The actual type using the getClass() method inherited from class Object. ⦁ The output of the toString() method. ⦁ The value returned by the purpose() method. ⦁ Create a Ship arraylist from the array. ⦁ Add one more CruiseShip instance to this arraylist. ⦁ Sort the arraylist by tonnage from low to high. ⦁ Report the value of the static numShips variable. ⦁ Finish up by using an ordinary for loop to run the toString() method of the arraylist elements. Possible Output An unordered fleet of various ships class chap11new.CruiseShip Ship name: Magic, year launched: 1998, GT 83338 2700 passenger capacity, operating in the Caribbean A holiday vessel for vacationers class chap11new.CruiseShip Ship name: Titanic, year launched: 1912, GT 46328 2435 passenger capacity, operating in the Atlantic Ocean A holiday vessel for vacationers class chap11new.CargoShip Ship name: Algeciras, year launched: 2020, GT 228283 Capacity of containers is 391 Hauling cargo across the seas class chap11new.CargoShip Ship name: Seawise Giant, year launched: 1979, GT 260941 Capacity of crude oil is 564763 Hauling cargo across the seas class chap11new.WarShip Ship name: USS Nimitz, year launched: 1972, GT 97000 Type: super carrier, operated by United States Navy A ship for defense or possibly attack class chap11new.WarShip Ship name: USS Zumwalt DDG 1000, year launched: 2013, GT 15656 Type: destroyer, operated by United States Navy A ship for defense or possibly attack Fleet size is now 7 Fleet sorted by tonnage after adding Symphony of the Seas Ship name: USS Zumwalt DDG 1000, year launched: 2013, GT 15656 Type: destroyer, operated by United States Navy Ship name: Titanic, year launched: 1912, GT 46328 2435 passenger capacity, operating in the Atlantic Ocean Ship name: Magic, year launched: 1998, GT 83338 2700 passenger capacity, operating in the Caribbean Ship name: USS Nimitz, year launched: 1972, GT 97000 Type: super carrier, operated by United States Navy Ship name: Symphony of the Seas, year launched: 2018, GT 228081 6680 passenger capacity, operating in the Atlantic Ocean Ship name: Algeciras, year launched: 2020, GT 228283 Capacity of containers is 391
Java programing
Ships
Study the UML diagram carefully. The green spots signify public. The red squares signify private. Superclass Ship is abstract because it contains the abstract method purpose. This class also implements the Comparable interface to compare ships by tonnage. Ship also has a public static integer named numShips that is incremented in the constructor to serve as a counter for the number of ships.
After coding these classes, write a program named TestShips that uses them as follows:
⦁ Create an array of declared type Ship that contains at least six ships (two of each concrete subclass). You can make up all of the values for the data members of each ship.
⦁ The Strings returned by purpose() can be determined by viewing the Possible Output below.
⦁ Process the array in an enhanced for loop to display for each ship:
⦁ The actual type using the getClass() method inherited from class Object.
⦁ The output of the toString() method.
⦁ The value returned by the purpose() method.
⦁ Create a Ship arraylist from the array.
⦁ Add one more CruiseShip instance to this arraylist.
⦁ Sort the arraylist by tonnage from low to high.
⦁ Report the value of the static numShips variable.
⦁ Finish up by using an ordinary for loop to run the toString() method of the arraylist elements.
Possible Output
An unordered fleet of various ships
class chap11new.CruiseShip
Ship name: Magic, year launched: 1998, GT 83338
2700 passenger capacity, operating in the Caribbean
A holiday vessel for vacationers
class chap11new.CruiseShip
Ship name: Titanic, year launched: 1912, GT 46328
2435 passenger capacity, operating in the Atlantic Ocean
A holiday vessel for vacationers
class chap11new.CargoShip
Ship name: Algeciras, year launched: 2020, GT 228283
Capacity of containers is 391
Hauling cargo across the seas
class chap11new.CargoShip
Ship name: Seawise Giant, year launched: 1979, GT 260941
Capacity of crude oil is 564763
Hauling cargo across the seas
class chap11new.WarShip
Ship name: USS Nimitz, year launched: 1972, GT 97000
Type: super carrier, operated by United States Navy
A ship for defense or possibly attack
class chap11new.WarShip
Ship name: USS Zumwalt DDG 1000, year launched: 2013, GT 15656
Type: destroyer, operated by United States Navy
A ship for defense or possibly attack
Fleet size is now 7
Fleet sorted by tonnage after adding Symphony of the Seas
Ship name: USS Zumwalt DDG 1000, year launched: 2013, GT 15656
Type: destroyer, operated by United States Navy
Ship name: Titanic, year launched: 1912, GT 46328
2435 passenger capacity, operating in the Atlantic Ocean
Ship name: Magic, year launched: 1998, GT 83338
2700 passenger capacity, operating in the Caribbean
Ship name: USS Nimitz, year launched: 1972, GT 97000
Type: super carrier, operated by United States Navy
Ship name: Symphony of the Seas, year launched: 2018, GT 228081
6680 passenger capacity, operating in the Atlantic Ocean
Ship name: Algeciras, year launched: 2020, GT 228283
Capacity of containers is 391
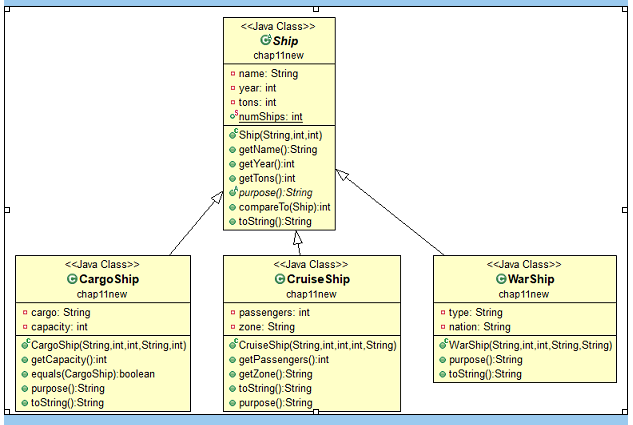

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

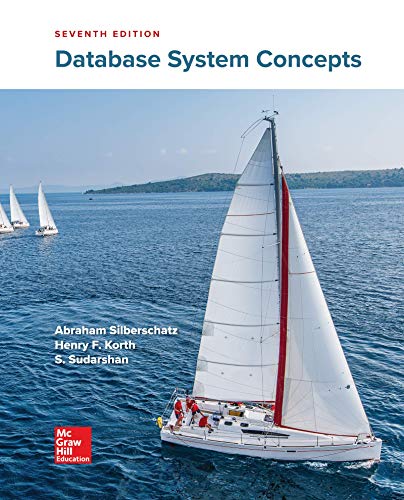
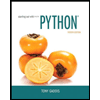
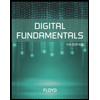
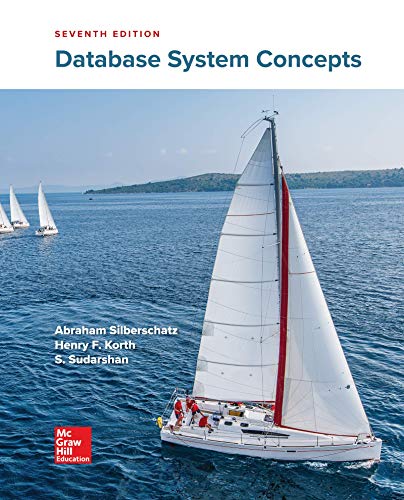
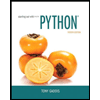
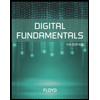
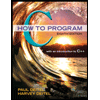
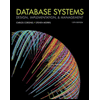
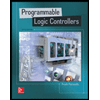