Implement all the classes using Java programming language from the given UML Class diagram. Note: This problem requires you to submit only one class: Ball.java. Do NOT include "public static void main()" method inside all of these classes. Graders will be testing your classes, using the unit-testing framework JUnit 4. A class called Ball is designed as shown in the class diagram. The Ball class contains the following private instance variables: x, y and radius, which represent the ball's center (x, y) co-ordinates and the radius, respectively. xDelta (Δx) and yDelta (Δy), which represent the displacement (movement) per step, in the x and y direction respectively. The Ball class contains the following public methods: A constructor which accepts x, y, radius, speed, and direction as arguments. For user friendliness, user specifies speed (in pixels per step) and direction (in degrees in the range of (-180°, 180°]). For the internal operations, the speed and direction are to be converted to (Δx, Δy) in the internal representation. Note that the y-axis of the Java graphics coordinate system is inverted, i.e., the origin (0, 0) is located at the top-left corner. Δx = d × cos(θ) Δy = -d × sin(θ) Hint: You will find Math.cos() and Math.toRadians(direction) static methods usefull. Don't forget that "d" is a speed. Getter and setter for all the instance variables. A method move() which move the ball by one step. x += Δx y += Δy reflectHorizontal() which reflects the ball horizontally (i.e., hitting a vertical wall) Δx = -Δx Δy no changes reflectVertical() (the ball hits a horizontal wall).Δx no changes Δy = -Δy toString() which prints the message "Ball at (x, y) of velocity (Δx, Δy)". Write and submit the Ball class.
Implement all the classes using Java programming language from the given UML Class diagram. Note: This problem requires you to submit only one class: Ball.java. Do NOT include "public static void main()" method inside all of these classes. Graders will be testing your classes, using the unit-testing framework JUnit 4. A class called Ball is designed as shown in the class diagram. The Ball class contains the following private instance variables: x, y and radius, which represent the ball's center (x, y) co-ordinates and the radius, respectively. xDelta (Δx) and yDelta (Δy), which represent the displacement (movement) per step, in the x and y direction respectively. The Ball class contains the following public methods: A constructor which accepts x, y, radius, speed, and direction as arguments. For user friendliness, user specifies speed (in pixels per step) and direction (in degrees in the range of (-180°, 180°]). For the internal operations, the speed and direction are to be converted to (Δx, Δy) in the internal representation. Note that the y-axis of the Java graphics coordinate system is inverted, i.e., the origin (0, 0) is located at the top-left corner. Δx = d × cos(θ) Δy = -d × sin(θ) Hint: You will find Math.cos() and Math.toRadians(direction) static methods usefull. Don't forget that "d" is a speed. Getter and setter for all the instance variables. A method move() which move the ball by one step. x += Δx y += Δy reflectHorizontal() which reflects the ball horizontally (i.e., hitting a vertical wall) Δx = -Δx Δy no changes reflectVertical() (the ball hits a horizontal wall).Δx no changes Δy = -Δy toString() which prints the message "Ball at (x, y) of velocity (Δx, Δy)". Write and submit the Ball class.
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 2GZ
Related questions
Question
Implement all the classes using Java
Note: This problem requires you to submit only one class: Ball.java.
Do NOT include "public static void main()" method inside all of these classes. Graders will be testing your classes, using the unit-testing framework JUnit 4.
A class called Ball is designed as shown in the class diagram.
The Ball class contains the following private instance variables:
- x, y and radius, which represent the ball's center (x, y) co-ordinates and the radius, respectively.
- xDelta (Δx) and yDelta (Δy), which represent the displacement (movement) per step, in the x and y direction respectively.
The Ball class contains the following public methods:
- A constructor which accepts x, y, radius, speed, and direction as arguments. For user friendliness, user specifies speed (in pixels per step) and direction (in degrees in the range of (-180°, 180°]). For the internal operations, the speed and direction are to be converted to (Δx, Δy) in the internal representation. Note that the y-axis of the Java graphics coordinate system is inverted, i.e., the origin (0, 0) is located at the top-left corner.
- Δx = d × cos(θ) Δy = -d × sin(θ)
Hint: You will find Math.cos() and Math.toRadians(direction) static methods usefull. Don't forget that "d" is a speed.
- Getter and setter for all the instance variables.
- A method move() which move the ball by one step. x += Δx y += Δy
- reflectHorizontal() which reflects the ball horizontally (i.e., hitting a vertical wall) Δx = -Δx Δy no changes
- reflectVertical() (the ball hits a horizontal wall).Δx no changes Δy = -Δy
- toString() which prints the message "Ball at (x, y) of velocity (Δx, Δy)".
Write and submit the Ball class.
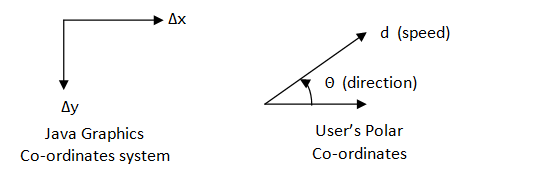
Transcribed Image Text:Ax
d (speed)
O (direction)
Ay
User's Polar
Java Graphics
Co-ordinates system
Co-ordinates
![Ball
-x:float
-y:float
-radius:int
Each move step advances x and y
by Ax and Ay. Ax and Ay could be
positive or negative.
|-xDelta:float
-yDelta:float
+Ball(x:float,y:float,radius:int
speed:int, direction:int)
+getX():float
+setX(x:float):void
+getY():float
+setY(y:float):void
+getRadius ():int
+setRadius (radius:int):void
+getXDelta():float
+setXDelta(xDelta:float):void
+getYDelta():float
+setYDelta(yDelta:float):void
+move ():void•-
+reflectHorizontal():voide
+reflectVertical():void•
+toString():String
Move one step:
х +3 Дх; у +3D Ду;
Ax = -Ax
Ay = -Ay
"Ball[(x,y),speed%-D(Ax,Ay)]"](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff3dad014-c6bb-4735-812f-053559c6a885%2F8d80b90b-1361-49cd-81b0-9d65b1ebc209%2Fjpq118q_processed.png&w=3840&q=75)
Transcribed Image Text:Ball
-x:float
-y:float
-radius:int
Each move step advances x and y
by Ax and Ay. Ax and Ay could be
positive or negative.
|-xDelta:float
-yDelta:float
+Ball(x:float,y:float,radius:int
speed:int, direction:int)
+getX():float
+setX(x:float):void
+getY():float
+setY(y:float):void
+getRadius ():int
+setRadius (radius:int):void
+getXDelta():float
+setXDelta(xDelta:float):void
+getYDelta():float
+setYDelta(yDelta:float):void
+move ():void•-
+reflectHorizontal():voide
+reflectVertical():void•
+toString():String
Move one step:
х +3 Дх; у +3D Ду;
Ax = -Ax
Ay = -Ay
"Ball[(x,y),speed%-D(Ax,Ay)]"
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
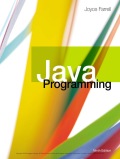
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
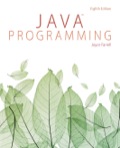
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
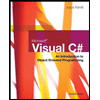
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
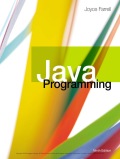
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
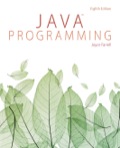
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
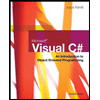
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,