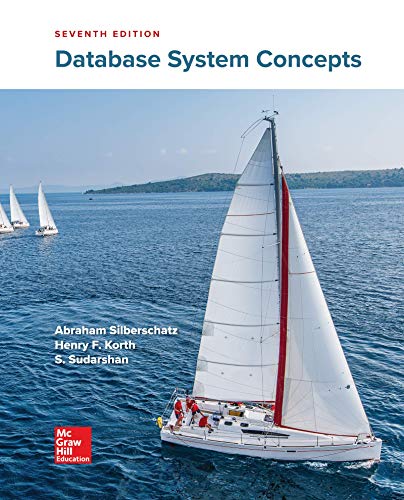
Java: Write a program to find the number of comparison using sequentialSearch and binarySearch algorithms using an array of 1200 elements. Use a random number generator to fill list by using a sorting
public class SearchSortAlgorithms<T> implements SearchSortADT<T>{
private int comparisons;
public int noOfComparisons(){
return comparisons;
}
public void initializeNoOfComparisons(){
comparisons = 0;
}
public int seqSearch(T[] list, int start, int length, T searchItem){
int loc;
boolean found = false;
for (loc = start; loc < length; loc++){
if (list[loc].equals(searchItem)){
found = true;
break;
}
}
if (found)
return loc;
else
return -1;
}
public int binarySearch(T[] list, int start, int length, T searchItem){
int first = start;
int last = length - 1;
int mid = -1;
boolean found = false;
while (first <= last && !found){
mid = (first + last) / 2;
Comparable<T> compElem = (Comparable<T>) list[mid];
if (compElem.compareTo(searchItem) == 0)
found = true;
else{
if (compElem.compareTo(searchItem) > 0)
last = mid - 1;
else
first = mid + 1;
}
}
if (found)
return mid;
else
return -1;
}
public int binSeqSearch15(T[] list, int start, int length, T searchItem){
int first = 0;
int last = length - 1;
int mid = -1;
boolean found = false;
while (last - first > 15 && !found){
mid = (first + last) / 2;
Comparable<T> compElem = (Comparable<T>) list[mid];
comparisons++;
if (compElem.compareTo(searchItem) ==0)
found = true;
else{
if (compElem.compareTo(searchItem) > 0)
last = mid - 1;
else
first = mid + 1;
}
}
if (found)
return mid;
else
return seqSearch(list, first, last, searchItem);
}
public void bubbleSort(T list[], int length){
for (int iteration = 1; iteration < length; iteration++){
for (int index = 0; index < length - iteration;
index++){
Comparable<T> compElem =
(Comparable<T>) list[index];
if (compElem.compareTo(list[index + 1]) > 0){
T temp = list[index];
list[index] = list[index + 1];
list[index + 1] = temp;
}
}
}
}
public void selectionSort(T[] list, int length){
for (int index = 0; index < length - 1; index++){
int minIndex = minLocation(list, index, length - 1);
swap(list, index, minIndex);
}
}
private int minLocation(T[] list, int first, int last) {
int minIndex = first;
for (int loc = first + 1; loc <= last; loc++){
Comparable<T> compElem = (Comparable<T>) list[loc];
if (compElem.compareTo(list[minIndex]) < 0)
minIndex = loc;
}
return minIndex;
}
private void swap(T[] list, int first, int second){
T temp;
temp = list[first];
list[first] = list[second];
list[second] = temp;
}
public void insertionSort(T[] list, int length){
for (int firstOutOfOrder = 1; firstOutOfOrder < length;
firstOutOfOrder ++){
Comparable<T> compElem =
(Comparable<T>) list[firstOutOfOrder];
if (compElem.compareTo(list[firstOutOfOrder - 1]) < 0){
Comparable<T> temp =
(Comparable<T>) list[firstOutOfOrder];
int location = firstOutOfOrder;
do{
list[location] = list[location - 1];
location--;
}
while (location > 0 &&
temp.compareTo(list[location - 1]) < 0);
list[location] = (T) temp;
}
}
}
public void quickSort(T[] list, int length){
recQuickSort(list, 0, length - 1);
}
private int partition(T[] list, int first, int last){
T pivot;
int smallIndex;
swap(list, first, (first + last) / 2);
pivot = list[first];
smallIndex = first;
for (int index = first + 1; index <= last; index++){
Comparable<T> compElem = (Comparable<T>) list[index];
if (compElem.compareTo(pivot) < 0){
smallIndex++;
swap(list, smallIndex, index);
}
}
swap(list, first, smallIndex);
return smallIndex;
}
private void recQuickSort(T[] list, int first, int last){
if (first < last){
int pivotLocation = partition(list, first, last);
recQuickSort(list, first, pivotLocation - 1);
recQuickSort(list, pivotLocation + 1, last);
}
}
public void heapSort(T[] list, int length){
buildHeap(list, length);
for (int lastOutOfOrder = length - 1; lastOutOfOrder >= 0;
lastOutOfOrder--){
T temp = list[lastOutOfOrder];
list[lastOutOfOrder] = list[0];
list[0] = temp;
heapify(list, 0, lastOutOfOrder - 1);
}
}
private void heapify(T[] list, int low, int high){
int largeIndex;
Comparable<T> temp =
(Comparable<T>) list[low];
largeIndex = 2 * low + 1;
while (largeIndex <= high){
if (largeIndex < high) {
Comparable<T> compElem =
(Comparable<T>) list[largeIndex];
if (compElem.compareTo(list[largeIndex + 1]) < 0)
largeIndex = largeIndex + 1;
}
if (temp.compareTo(list[largeIndex]) > 0)
break;
else{
list[low] = list[largeIndex];
low = largeIndex;
largeIndex = 2 * low + 1;
}
}
list[low] = (T) temp;
}
private void buildHeap(T[] list, int length){
for (int index = length / 2 - 1; index >= 0; index--)
heapify(list, index, length - 1);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Heapsort has heapified an array to: 99 95 67 42 15 40 26 and is about to start the second for loop. What is the array after the first iteration of the second for loop? Ex: 98, 36, 41arrow_forwardlet list=1200 element. Note: java programmimg, use given code. 3a. Use the binary search 3b. Use the sequential search Print the number of comparison in step 3(a) and 3(b). If the item is found in the list, print its position. import java.util.*; public class Problem5 { staticScannerconsole = newScanner(System.in); finalstaticintSIZE = 1000; publicstaticvoidmain(String[] args) { Integer[] intList = newInteger[SIZE]; SearchSortAlgorithms<Integer> intSearchObject = new SearchSortAlgorithms<Integer>(); //code } public interface SearchSortADT<T> { publicintseqSearch(T[] list, intstart, intlength, TsearchItem); publicintbinarySearch(T[] list, intstart, intlength, TsearchItem); publicvoidbubbleSort(Tlist[], intlength); publicvoidselectionSort(T[] list, intlength); publicvoidinsertionSort(T[] list, intlength); publicvoidquickSort(T[] list, intlength); publicvoidheapSort(T[] list, intlength); } public class SearchSortAlgorithms<T> implements…arrow_forwardSingle number (use XOR): Given a non-empty array of integers nums, every element appears twice except for one. Find that single one. You must implement a solution with a linear runtime complexity and use only constant extra space. Code in Java A brief explanation of how and why your code works and how it relates to the concepts learned (for example, it uses bitwise operation, stack, or takes into account a word size).arrow_forward
- (a) Write a method public static void insert(int[] a, int n, int x) that inserts x in order among the first n elements of a, assuming these elements are arranged in ascending order. Do NOT use arraylists. x is the last element in a. n does not include x. (b) Using the insert method from Part (a), write a recursive implementation of Insertion Sort.arrow_forwardimport random; #importing random module aGrades=[]; #list to store grades of all tests for i in range(0,7):aGrades.append(random.randrange(0,100)); #generating 7 random values for testsexamCount=len(aGrades)-1; #examCount is a variable to store count of all exams except last exam totalPoints=sum(aGrades)-aGrades[len(aGrades)-1]; #totalPoints is a variable to store sum of all scores excluding last exam testAverage=(totalPoints)/examCount; #testAverage is a variable used to store the average of scores except last exam finalAverage= (testAverage*0.6)+(aGrades[len(aGrades)-1]*0.4); #finalAverage is a variable to store weighted average of the tests print(aGrades); #printing list of gradesprint(testAverage); #printing test averageprint(finalAverage); #printing final average How do i rewrite the Grades List programs above using a loop instead of referencing the elements of the lists by index.arrow_forwardComplete the below function (using recursion)arrow_forward
- JAVA: Suppose that we have a sorted int array called x, with n elements in it and some room to add more elements. Write the code segment that would add a new item, called target, to the array, so that the array will still be sorted once you are done.arrow_forwardnputer Science I| for Majors home > 13.4: Recursive Helper Methods E zyBa Feedb PARTICIPATION 13.4.3: Compute recursively to determine the sum of values in an array. АCTIVITY Assume you have the following method: public static int sum(int [] a) To compute the sum of the values in an array, add the first value to the sum of the remaining values, computing recursively. Rearrange the following lines to design a recursive helper method to solve this problem. Not all lines are useful. Mouse: Drag/drop Keyboard: Grab/release Spacebar (or Enter). Move 0000 Cancel Esc Unused FindSum.java Load default template.. return a[start] + sumHelper(a, start - 1); import java.util.Scanner; return alstart] + sumHelper(a, start + 1); public class FindSum { if (start == a. length) { return a[start); } if (start == a.length - 1) { return alstart]; } public static int sum(int[0 a) if (a.length == 0) { return -1; } return sumHelper(a, 0); if (a.length == 0) { return 0; } public static int sumHelper(int [] a,…arrow_forwardImplement a function void printAll(void) to print all the items of the list.arrow_forward
- Java - This project will allow you to compare & contrast different 4 sorting techniques, the last of which will be up to you to select. You will implement the following: Bubble Sort (pair-wise) Bubble Sort (list-wise) [This is the selection sort] Merge Sort Your choice (candidates are the heap, quick, shell, cocktail, bucket, or radix sorts) [These will require independent research) General rules: Structures can be static or dynamic You are not allowed to use built in methods that are direct or indirect requirements for this project – You cannot use ANY built in sorting functions - I/O (System.in/out *) are ok. All compare/swap/move methods must be your own. (You can use string compares) Your program will be sorting names – you need at least 100 unique names (you can use the 50 given in project #3) – read them into the program in a random fashion (i.e. not in any kind of alpha order). *The more names you have, the easier it is to see trends in speed. All sorts will be from…arrow_forwardPYTHON LAB: Inserting an integer in descending order (doubly-linked list) Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 _____________________________________________________________________________________ Main.py: from IntNode import IntNode from IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py class IntNode: def __init__(self, initial_data, next = None,…arrow_forwardComplete the recursive function remove_last which creates a new list identical to the input list s but with the last element in the sequence that is equal to x removed. Hint: Remember that you can use negative indexing on lists! For example 1st [-1] refers to the last element in a list 1st, lst [-2] refers to the second to last element... def remove_last(x, s): """Create a new list that is identical to s but with the last element from the list that is equal to x removed. >>> remove_last(1, []) [] >>> remove_last(1, [1]) [] >>> [1] >>> [2] remove_last(1, [1,1]) remove_last(1, [2,1]) >>> remove_last(1, [3,1,2]) [3, 2] >>> remove_last(1, [3,1,2,1]) [3, 1, 2] >>> remove_last (5, [3, 5, 2, 5, 11]) [3, 5, 2, 11] IIIII "*** YOUR CODE HERE ***" Illustrated here is a more complete doctest that shows good testing methodology. It is a little cumbersome as documentation, but you'll want to think about it for your projects. Test every condition that might come up. Then you won't be surprised when…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
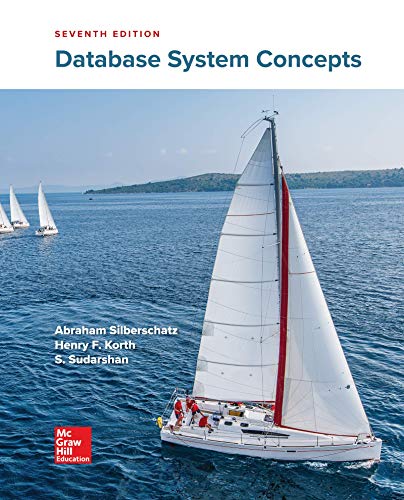
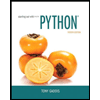
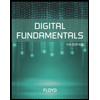
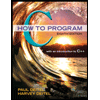
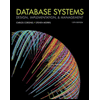
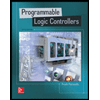